
cc3100_Socket_Wifi_Server with Ethernet Interface not working
Dependencies: EthernetInterface mbed-rtos mbed
Fork of cc3100_Test_Demo by
main.cpp
00001 #include "cc3100_simplelink.h" 00002 #include "cc3100_sl_common.h" 00003 #include "fPtr_func.h" 00004 #include "cc3100.h" 00005 #include "cc3100_spi.h" 00006 #include "myBoardInit.h" 00007 #include "cc3100_socket.h" 00008 #include "cc3100_driver.h" 00009 #include "cc3100_nonos.h" 00010 #include "mbed.h" 00011 #include "rtos.h" 00012 //#include "EthernetInterface.h" 00013 00014 using namespace mbed_cc3100; 00015 00016 #define SL_STOP_TIMEOUT 0xFF 00017 00018 /* IP addressed of server side socket. Should be in long format, 00019 * E.g: 0xc0a8010a == 192.168.1.10 */ 00020 //#define IP_ADDR 0xc0a82b69 //192.168.43.105 00021 #define PORT_NUM 50001 /* Port number to be used */ 00022 00023 #define BUF_SIZE 1400 00024 #define NO_OF_PACKETS 1000 00025 00026 cc3100 _cc3100(p9, p10, p8, SPI(p11, p12, p13));//LPC1768 irq, nHib, cs, mosi, miso, sck 00027 Serial pc(USBTX, USBRX);//lpc1768 00028 DigitalOut myled1(LED1); 00029 DigitalOut myled2(LED2); 00030 DigitalOut myled3(LED3); 00031 DigitalOut myled4(LED4); 00032 00033 00034 //GLOBAL VARIABLES -- Start 00035 int32_t demo = 0; 00036 //GLOBAL VARIABLES -- End 00037 00038 00039 static void displayBanner() 00040 { 00041 printf("\n\r\n\r"); 00042 printf("***********Getting started with station application***********"); 00043 printf("\n\r*******************************************************************************\n\r"); 00044 } 00045 00046 00047 //******************************STATION_MODE*********************************************** 00048 //***************************************************************************************** 00049 void station_app() 00050 { 00051 int32_t retVal = -1; 00052 00053 /* Connecting to WLAN AP */ 00054 retVal = _cc3100.establishConnectionWithAP(); 00055 //printf("retVal 000: %d\n\r",retVal); 00056 if(retVal < 0) 00057 { 00058 printf(" Failed to establish connection w/ an AP \n\r"); 00059 LOOP_FOREVER(); 00060 } 00061 printf(" Connection established w/ AP and IP is acquired \n\r"); 00062 00063 printf(" Pinging...! \n\r"); 00064 retVal = _cc3100.checkLanConnection(); 00065 //printf("retVal 001: %d\n\r",retVal); 00066 if(retVal < 0) 00067 { 00068 printf(" Device couldn't connect to LAN \n\r"); 00069 LOOP_FOREVER(); 00070 } 00071 printf(" Device successfully connected to the LAN\n\r"); 00072 /* 00073 retVal = _cc3100.checkInternetConnection(); 00074 if(retVal < 0) 00075 { 00076 printf(" Device couldn't connect to the internet \n\r"); 00077 LOOP_FOREVER(); 00078 } 00079 00080 printf(" Device successfully connected to the internet \n\r");*/ 00081 } 00082 //****************************END_STATION_MODE********************************************* 00083 //***************************************************************************************** 00084 void led(int result){ 00085 00086 if (result > 0) 00087 { 00088 myled1 = 1; 00089 wait(0.05); 00090 myled1 = 0; 00091 wait(0.05); 00092 myled2 = 1; 00093 wait(0.05); 00094 myled2 = 0; 00095 wait(0.05); 00096 myled3 = 1; 00097 wait(0.05); 00098 myled3 = 0; 00099 wait(0.05); 00100 myled4 = 1; 00101 wait(0.05); 00102 myled4 = 0; 00103 wait(0.05); 00104 } 00105 else 00106 { myled1 = 0; 00107 myled2 = 0; 00108 myled3 = 0; 00109 myled4 = 0; 00110 } 00111 00112 } 00113 00114 //osThreadDef(led, osPriorityNormal, DEFAULT_STACK_SIZE); 00115 static int32_t BsdTcpServer(uint16_t Port) 00116 { 00117 00118 SlSockAddrIn_t Addr; 00119 SlSockAddrIn_t LocalAddr; 00120 char stringa[100]; 00121 //int16_t idx = 0; 00122 int16_t AddrSize = 0; 00123 int16_t SockID = 0; 00124 int32_t Status = 0; 00125 int16_t newSockID = 0; 00126 int16_t LoopCount = 0; 00127 //int16_t SlSockAddrIn_t = 0; 00128 int16_t recvSize = 0; 00129 //Thread thread; 00130 00131 /* 00132 for (idx=0 ; idx<BUF_SIZE ; idx++) 00133 { 00134 uBuf.BsdBuf[idx] = (_u8)(idx % 10); 00135 } 00136 */ 00137 00138 LocalAddr.sin_family = SL_AF_INET; 00139 LocalAddr.sin_port = _cc3100._socket.sl_Htons(PORT_NUM); 00140 LocalAddr.sin_addr.s_addr = 0; 00141 //int port = 1; 00142 //osThreadCreate(osThread(led), (void *) port); 00143 //thread.start(led(1)); 00144 00145 SockID = _cc3100._socket.sl_Socket(SL_AF_INET,SL_SOCK_STREAM, 0); 00146 if( SockID < 0 ) 00147 { 00148 printf(" \n[TCP Server] Create socket Error \n\r"); 00149 ASSERT_ON_ERROR(SockID); 00150 } 00151 else 00152 printf("\nCreate socket \n\r"); 00153 00154 00155 AddrSize = sizeof(SlSockAddrIn_t); 00156 Status = _cc3100._socket.sl_Bind(SockID, (SlSockAddr_t *)&LocalAddr, AddrSize); 00157 if( Status < 0 ) 00158 { 00159 _cc3100._socket.sl_Close(SockID); 00160 printf(" \n[TCP Server] Socket address assignment Error \n\r"); 00161 ASSERT_ON_ERROR(Status); 00162 } 00163 else 00164 printf("\nSocket address assignment \n\r"); 00165 00166 Status = _cc3100._socket.sl_Listen(SockID, 0); 00167 if( Status < 0 ) 00168 { 00169 _cc3100._socket.sl_Close(SockID); 00170 printf("\n [TCP Server] Listen Error \n\r"); 00171 ASSERT_ON_ERROR(Status); 00172 } 00173 else 00174 printf("\nListen for a Connection \n\r"); 00175 00176 newSockID = _cc3100._socket.sl_Accept(SockID, (SlSockAddr_t *)&Addr,(SlSocklen_t*)&AddrSize); 00177 if( newSockID < 0 ) 00178 { 00179 _cc3100._socket.sl_Close(SockID); 00180 printf("\n [TCP Server] Accept connection Error \n\r"); 00181 ASSERT_ON_ERROR(newSockID); 00182 } 00183 else 00184 printf("\nAccept connection \n\r"); 00185 00186 while (LoopCount < NO_OF_PACKETS) 00187 { 00188 //TODO : sarebbe da chiamare la funzione led() con un altro thread così da farla girare in background 00189 led(1); 00190 recvSize = BUF_SIZE; 00191 //printf("RecvSize = %d \n\r",recvSize); 00192 do 00193 { 00194 Status = _cc3100._socket.sl_Recv(newSockID, &(stringa), strlen(stringa), 0); 00195 if( Status <= 0 ) 00196 { 00197 _cc3100._socket.sl_Close(newSockID); 00198 _cc3100._socket.sl_Close(SockID); 00199 led(1); 00200 printf(" \n[TCP Server] Data recv Error. Close socket %d \n\n\r",SockID); 00201 //ASSERT_ON_ERROR(TCP_RECV_ERROR); 00202 00203 /*-----Accept new connection----------*/ 00204 SockID = _cc3100._socket.sl_Socket(SL_AF_INET,SL_SOCK_STREAM, 0); 00205 printf("----------Create new socket---------- \n\n\r"); 00206 AddrSize = sizeof(SlSockAddrIn_t); 00207 Status = _cc3100._socket.sl_Bind(SockID, (SlSockAddr_t *)&LocalAddr, AddrSize); 00208 printf("----------Socket address assignment---------- \n\n\r"); 00209 Status = _cc3100._socket.sl_Listen(SockID, 0); 00210 printf("----------Listen for a new Connection---------- \n\n\r"); 00211 newSockID = _cc3100._socket.sl_Accept(SockID, (SlSockAddr_t *)&Addr,(SlSocklen_t*)&AddrSize); 00212 printf("----------Accept new connection---------- \n\n\r"); 00213 led(1); 00214 } 00215 00216 //printf("Status = %d \n\r",Status); 00217 recvSize -= Status; 00218 //printf("RecvSize = %d \n\r",recvSize); 00219 printf("%s",stringa); 00220 led(1); 00221 char thanks [] = "Data received"; 00222 _cc3100._socket.sl_Send(SockID, &(thanks), strlen(thanks), 0); 00223 00224 } 00225 while(recvSize > 0); 00226 00227 LoopCount++; 00228 } 00229 00230 Status = _cc3100._socket.sl_Close(newSockID); 00231 ASSERT_ON_ERROR(Status); 00232 00233 Status = _cc3100._socket.sl_Close(SockID); 00234 ASSERT_ON_ERROR(Status); 00235 00236 return SUCCESS; 00237 00238 } 00239 00240 00241 00242 //**************************_START_APPLICATION_********************************* 00243 int main(void) 00244 { 00245 pc.baud(115200); 00246 00247 00248 00249 int32_t retVal = -1; 00250 00251 retVal = _cc3100.initializeAppVariables(); 00252 00253 displayBanner(); 00254 00255 _cc3100.CLR_STATUS_BIT(g_Status, STATUS_BIT_PING_DONE); 00256 g_PingPacketsRecv = 0; 00257 //printf("\n\r FUNZIONE CLR_STATUS_BIT ESEGUITA CON SUCCESSO\n\r"); 00258 00259 //retVal = _cc3100.configureSimpleLinkToDefaultState(); 00260 00261 //printf("\n\rRetval 2: %d \n\r ",retVal); 00262 00263 if(retVal < 0) { 00264 if (DEVICE_NOT_IN_STATION_MODE == retVal) 00265 printf(" Failed to configure the device to its default state \n\r"); 00266 00267 LOOP_FOREVER(); 00268 } 00269 00270 printf("\n\rDevice is configured in it's default state \n\r"); 00271 /*printf("\n\r******************************************************************************************************\n\r"); 00272 printf("\n\r*****_DA_QUI_IN_POI_SCELTA_TIPO_FUNZIONE_IN_BASE_AL_VALORE_demo_*****\n\r"); 00273 printf("\n\r******************************************************************************************************\n\r");*/ 00274 00275 /* 00276 * Assumption is that the device is configured in station mode already 00277 * and it is in its default state 00278 */ 00279 /* Initializing the CC3100 device */ 00280 00281 retVal = _cc3100.sl_Start(0, 0, 0); 00282 00283 if ((retVal < 0) || (ROLE_STA != retVal) ) 00284 { 00285 printf(" Failed to start the device \n\r"); 00286 LOOP_FOREVER(); 00287 } 00288 00289 printf("\n\r Device started as STATION \n\r"); 00290 00291 00292 station_app(); 00293 00294 printf("Establishing connection with TCP server \n\r"); 00295 /*Before proceeding, please make sure to have a server waiting on PORT_NUM*/ 00296 retVal = BsdTcpServer(PORT_NUM); 00297 if(retVal < 0) 00298 printf(" Failed to establishing connection with TCP server \n\r"); 00299 else 00300 printf(" Connection with TCP server established successfully \n\r"); 00301 00302 return 0; 00303 00304 00305 00306 00307 } 00308
Generated on Thu Jul 28 2022 21:30:48 by
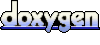