
Fork of mbed RPC_serial to fix build errors. mbed RPC_Serial for LabVIEW interface: http://mbed.org/cookbook/Interfacing-with-LabVIEW http://mbed.org/cookbook/Interfacing-Using-RPC Steps: *Compile RPC_Serial main *Copy bit file to mbed flash and reset *Run examples in LabVIEW
Fork of RPC_Serial by
main.cpp
00001 #include "mbed.h" 00002 #include "mbed_rpc.h" 00003 00004 //using namespace mbed; 00005 00006 Serial pc(USBTX, USBRX); 00007 00008 int main() { 00009 // setup the classes that can be created dynamically 00010 00011 //These exist for all platforms 00012 RPC::add_rpc_class<RpcDigitalIn>(); 00013 RPC::add_rpc_class<RpcDigitalOut>(); 00014 RPC::add_rpc_class<RpcDigitalInOut>(); 00015 RPC::add_rpc_class<RpcTimer>(); 00016 00017 //Others are conditional by platform 00018 00019 //Unknown how RPC would work if serial was not present? 00020 #if DEVICE_SERIAL 00021 RPC::add_rpc_class<RpcSerial>(); 00022 #else 00023 #warning "No Serial function available in this configuration." 00024 #endif 00025 00026 #if DEVICE_ANALOGIN 00027 RPC::add_rpc_class<RpcAnalogIn>(); 00028 #else 00029 #warning "No AnalogIn function available in this configuration." 00030 #endif 00031 00032 #if DEVICE_ANALOGOUT 00033 RPC::add_rpc_class<RpcAnalogOut>(); 00034 #else 00035 #warning "No AnalogOut function available in this configuration." 00036 #endif 00037 00038 #if DEVICE_PWMOUT 00039 RPC::add_rpc_class<RpcPwmOut>(); 00040 #else 00041 #warning "No PWMOut function available in this configuration." 00042 #endif 00043 00044 #if DEVICE_SPI 00045 RPC::add_rpc_class<RpcSPI>(); 00046 #else 00047 #warning "No SPI function available in this configuration." 00048 #endif 00049 00050 //These appear to not be implemented 00051 //RPC::add_rpc_class<BusOut>(); 00052 //RPC::add_rpc_class<RpcBusIn>(); 00053 //RPC::add_rpc_class<RpcBusInOut>(); 00054 00055 // receive commands, and send back the responses 00056 char buf[RPC_MAX_STRING], outbuf[RPC_MAX_STRING]; 00057 while(1) { 00058 pc.gets(buf, RPC_MAX_STRING); 00059 RPC::call(buf, outbuf); 00060 pc.printf("%s\n", outbuf); 00061 } 00062 00063 }
Generated on Sun Jul 17 2022 00:23:17 by
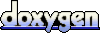