This fork captures the mbed lib v125 for ease of integration into older projects.
Fork of mbed-dev by
toolchain.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef MBED_TOOLCHAIN_H 00017 #define MBED_TOOLCHAIN_H 00018 00019 00020 // Warning for unsupported compilers 00021 #if !defined(__GNUC__) /* GCC */ \ 00022 && !defined(__CC_ARM) /* ARMCC */ \ 00023 && !defined(__clang__) /* LLVM/Clang */ \ 00024 && !defined(__ICCARM__) /* IAR */ 00025 #warning "This compiler is not yet supported." 00026 #endif 00027 00028 00029 // Attributes 00030 00031 /** MBED_PACKED 00032 * Pack a structure, preventing any padding from being added between fields. 00033 * 00034 * @code 00035 * #include "toolchain.h" 00036 * 00037 * MBED_PACKED(struct) foo { 00038 * char x; 00039 * int y; 00040 * }; 00041 * @endcode 00042 */ 00043 #ifndef MBED_PACKED 00044 #if defined(__ICCARM__) 00045 #define MBED_PACKED(struct) __packed struct 00046 #else 00047 #define MBED_PACKED(struct) struct __attribute__((packed)) 00048 #endif 00049 #endif 00050 00051 /** MBED_ALIGN(N) 00052 * Declare a variable to be aligned on an N-byte boundary. 00053 * 00054 * @note 00055 * IAR does not support alignment greater than word size on the stack 00056 * 00057 * @code 00058 * #include "toolchain.h" 00059 * 00060 * MBED_ALIGN(16) char a; 00061 * @endcode 00062 */ 00063 #ifndef MBED_ALIGN 00064 #if defined(__ICCARM__) 00065 #define _MBED_ALIGN(N) _Pragma(#N) 00066 #define MBED_ALIGN(N) _MBED_ALIGN(data_alignment=N) 00067 #else 00068 #define MBED_ALIGN(N) __attribute__((aligned(N))) 00069 #endif 00070 #endif 00071 00072 /** MBED_UNUSED 00073 * Declare a function argument to be unused, suppressing compiler warnings 00074 * 00075 * @code 00076 * #include "toolchain.h" 00077 * 00078 * void foo(MBED_UNUSED int arg) { 00079 * 00080 * } 00081 * @endcode 00082 */ 00083 #ifndef MBED_UNUSED 00084 #if defined(__GNUC__) || defined(__clang__) || defined(__CC_ARM) 00085 #define MBED_UNUSED __attribute__((__unused__)) 00086 #else 00087 #define MBED_UNUSED 00088 #endif 00089 #endif 00090 00091 /** MBED_WEAK 00092 * Mark a function as being weak. 00093 * 00094 * @note 00095 * weak functions are not friendly to making code re-usable, as they can only 00096 * be overridden once (and if they are multiply overridden the linker will emit 00097 * no warning). You should not normally use weak symbols as part of the API to 00098 * re-usable modules. 00099 * 00100 * @code 00101 * #include "toolchain.h" 00102 * 00103 * MBED_WEAK void foo() { 00104 * // a weak implementation of foo that can be overriden by a definition 00105 * // without __weak 00106 * } 00107 * @endcode 00108 */ 00109 #ifndef MBED_WEAK 00110 #if defined(__ICCARM__) 00111 #define MBED_WEAK __weak 00112 #else 00113 #define MBED_WEAK __attribute__((weak)) 00114 #endif 00115 #endif 00116 00117 /** MBED_PURE 00118 * Hint to the compiler that a function depends only on parameters 00119 * 00120 * @code 00121 * #include "toolchain.h" 00122 * 00123 * MBED_PURE int foo(int arg){ 00124 * // no access to global variables 00125 * } 00126 * @endcode 00127 */ 00128 #ifndef MBED_PURE 00129 #if defined(__GNUC__) || defined(__clang__) || defined(__CC_ARM) 00130 #define MBED_PURE __attribute__((const)) 00131 #else 00132 #define MBED_PURE 00133 #endif 00134 #endif 00135 00136 /** MBED_FORCEINLINE 00137 * Declare a function that must always be inlined. Failure to inline 00138 * such a function will result in an error. 00139 * 00140 * @code 00141 * #include "toolchain.h" 00142 * 00143 * MBED_FORCEINLINE void foo() { 00144 * 00145 * } 00146 * @endcode 00147 */ 00148 #ifndef MBED_FORCEINLINE 00149 #if defined(__GNUC__) || defined(__clang__) || defined(__CC_ARM) 00150 #define MBED_FORCEINLINE static inline __attribute__((always_inline)) 00151 #elif defined(__ICCARM__) 00152 #define MBED_FORCEINLINE _Pragma("inline=forced") static 00153 #else 00154 #define MBED_FORCEINLINE static inline 00155 #endif 00156 #endif 00157 00158 /** MBED_NORETURN 00159 * Declare a function that will never return. 00160 * 00161 * @code 00162 * #include "toolchain.h" 00163 * 00164 * MBED_NORETURN void foo() { 00165 * // must never return 00166 * while (1) {} 00167 * } 00168 * @endcode 00169 */ 00170 #ifndef MBED_NORETURN 00171 #if defined(__GNUC__) || defined(__clang__) || defined(__CC_ARM) 00172 #define MBED_NORETURN __attribute__((noreturn)) 00173 #elif defined(__ICCARM__) 00174 #define MBED_NORETURN __noreturn 00175 #else 00176 #define MBED_NORETURN 00177 #endif 00178 #endif 00179 00180 /** MBED_UNREACHABLE 00181 * An unreachable statement. If the statement is reached, 00182 * behaviour is undefined. Useful in situations where the compiler 00183 * cannot deduce the unreachability of code. 00184 * 00185 * @code 00186 * #include "toolchain.h" 00187 * 00188 * void foo(int arg) { 00189 * switch (arg) { 00190 * case 1: return 1; 00191 * case 2: return 2; 00192 * ... 00193 * } 00194 * MBED_UNREACHABLE; 00195 * } 00196 * @endcode 00197 */ 00198 #ifndef MBED_UNREACHABLE 00199 #if (defined(__GNUC__) || defined(__clang__)) && !defined(__CC_ARM) 00200 #define MBED_UNREACHABLE __builtin_unreachable() 00201 #else 00202 #define MBED_UNREACHABLE while (1) 00203 #endif 00204 #endif 00205 00206 /** MBED_DEPRECATED("message string") 00207 * Mark a function declaration as deprecated, if it used then a warning will be 00208 * issued by the compiler possibly including the provided message. Note that not 00209 * all compilers are able to display the message. 00210 * 00211 * @code 00212 * #include "toolchain.h" 00213 * 00214 * MBED_DEPRECATED("don't foo any more, bar instead") 00215 * void foo(int arg); 00216 * @endcode 00217 */ 00218 #ifndef MBED_DEPRECATED 00219 #if defined(__GNUC__) || defined(__clang__) 00220 #define MBED_DEPRECATED(M) __attribute__((deprecated(M))) 00221 #elif defined(__CC_ARM) 00222 #define MBED_DEPRECATED(M) __attribute__((deprecated)) 00223 #else 00224 #define MBED_DEPRECATED(M) 00225 #endif 00226 #endif 00227 00228 /** MBED_DEPRECATED_SINCE("version", "message string") 00229 * Mark a function declaration as deprecated, noting that the declaration was 00230 * deprecated on the specified version. If the function is used then a warning 00231 * will be issued by the compiler possibly including the provided message. 00232 * Note that not all compilers are able to display this message. 00233 * 00234 * @code 00235 * #include "toolchain.h" 00236 * 00237 * MBED_DEPRECATED_SINCE("mbed-os-5.1", "don't foo any more, bar instead") 00238 * void foo(int arg); 00239 * @endcode 00240 */ 00241 #define MBED_DEPRECATED_SINCE(D, M) MBED_DEPRECATED(M " [since " D "]") 00242 00243 00244 // FILEHANDLE declaration 00245 #if defined(TOOLCHAIN_ARM) 00246 #include <rt_sys.h> 00247 #endif 00248 00249 #ifndef FILEHANDLE 00250 typedef int FILEHANDLE; 00251 #endif 00252 00253 // Backwards compatibility 00254 #ifndef WEAK 00255 #define WEAK MBED_WEAK 00256 #endif 00257 00258 #ifndef PACKED 00259 #define PACKED MBED_PACKED() 00260 #endif 00261 00262 #ifndef EXTERN 00263 #define EXTERN extern 00264 #endif 00265 00266 #endif
Generated on Tue Jul 12 2022 19:06:16 by
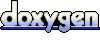