This fork captures the mbed lib v125 for ease of integration into older projects.
Fork of mbed-dev by
i2c_api.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2015 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef MBED_I2C_API_H 00017 #define MBED_I2C_API_H 00018 00019 #include "device.h" 00020 #include "buffer.h" 00021 00022 #if DEVICE_I2C_ASYNCH 00023 #include "dma_api.h" 00024 #endif 00025 00026 #if DEVICE_I2C 00027 00028 /** 00029 * @defgroup hal_I2CEvents I2C Events Macros 00030 * 00031 * @{ 00032 */ 00033 #define I2C_EVENT_ERROR (1 << 1) 00034 #define I2C_EVENT_ERROR_NO_SLAVE (1 << 2) 00035 #define I2C_EVENT_TRANSFER_COMPLETE (1 << 3) 00036 #define I2C_EVENT_TRANSFER_EARLY_NACK (1 << 4) 00037 #define I2C_EVENT_ALL (I2C_EVENT_ERROR | I2C_EVENT_TRANSFER_COMPLETE | I2C_EVENT_ERROR_NO_SLAVE | I2C_EVENT_TRANSFER_EARLY_NACK) 00038 00039 /**@}*/ 00040 00041 #if DEVICE_I2C_ASYNCH 00042 /** Asynch I2C HAL structure 00043 */ 00044 typedef struct { 00045 struct i2c_s i2c; /**< Target specific I2C structure */ 00046 struct buffer_s tx_buff; /**< Tx buffer */ 00047 struct buffer_s rx_buff; /**< Rx buffer */ 00048 } i2c_t; 00049 00050 #else 00051 /** Non-asynch I2C HAL structure 00052 */ 00053 typedef struct i2c_s i2c_t; 00054 00055 #endif 00056 00057 enum { 00058 I2C_ERROR_NO_SLAVE = -1, 00059 I2C_ERROR_BUS_BUSY = -2 00060 }; 00061 00062 #ifdef __cplusplus 00063 extern "C" { 00064 #endif 00065 00066 /** 00067 * \defgroup hal_GeneralI2C I2C Configuration Functions 00068 * @{ 00069 */ 00070 00071 /** Initialize the I2C peripheral. It sets the default parameters for I2C 00072 * peripheral, and configures its specifieds pins. 00073 * 00074 * @param obj The I2C object 00075 * @param sda The sda pin 00076 * @param scl The scl pin 00077 */ 00078 void i2c_init(i2c_t *obj, PinName sda, PinName scl); 00079 00080 /** Configure the I2C frequency 00081 * 00082 * @param obj The I2C object 00083 * @param hz Frequency in Hz 00084 */ 00085 void i2c_frequency(i2c_t *obj, int hz); 00086 00087 /** Send START command 00088 * 00089 * @param obj The I2C object 00090 */ 00091 int i2c_start(i2c_t *obj); 00092 00093 /** Send STOP command 00094 * 00095 * @param obj The I2C object 00096 */ 00097 int i2c_stop(i2c_t *obj); 00098 00099 /** Blocking reading data 00100 * 00101 * @param obj The I2C object 00102 * @param address 7-bit address (last bit is 1) 00103 * @param data The buffer for receiving 00104 * @param length Number of bytes to read 00105 * @param stop Stop to be generated after the transfer is done 00106 * @return Number of read bytes 00107 */ 00108 int i2c_read(i2c_t *obj, int address, char *data, int length, int stop); 00109 00110 /** Blocking sending data 00111 * 00112 * @param obj The I2C object 00113 * @param address 7-bit address (last bit is 0) 00114 * @param data The buffer for sending 00115 * @param length Number of bytes to write 00116 * @param stop Stop to be generated after the transfer is done 00117 * @return Number of written bytes 00118 */ 00119 int i2c_write(i2c_t *obj, int address, const char *data, int length, int stop); 00120 00121 /** Reset I2C peripheral. TODO: The action here. Most of the implementation sends stop() 00122 * 00123 * @param obj The I2C object 00124 */ 00125 void i2c_reset(i2c_t *obj); 00126 00127 /** Read one byte 00128 * 00129 * @param obj The I2C object 00130 * @param last Acknoledge 00131 * @return The read byte 00132 */ 00133 int i2c_byte_read(i2c_t *obj, int last); 00134 00135 /** Write one byte 00136 * 00137 * @param obj The I2C object 00138 * @param data Byte to be written 00139 * @return 0 if NAK was received, 1 if ACK was received, 2 for timeout. 00140 */ 00141 int i2c_byte_write(i2c_t *obj, int data); 00142 00143 /**@}*/ 00144 00145 #if DEVICE_I2CSLAVE 00146 00147 /** 00148 * \defgroup SynchI2C Synchronous I2C Hardware Abstraction Layer for slave 00149 * @{ 00150 */ 00151 00152 /** Configure I2C as slave or master. 00153 * @param obj The I2C object 00154 * @return non-zero if a value is available 00155 */ 00156 void i2c_slave_mode(i2c_t *obj, int enable_slave); 00157 00158 /** Check to see if the I2C slave has been addressed. 00159 * @param obj The I2C object 00160 * @return The status - 1 - read addresses, 2 - write to all slaves, 00161 * 3 write addressed, 0 - the slave has not been addressed 00162 */ 00163 int i2c_slave_receive(i2c_t *obj); 00164 00165 /** Configure I2C as slave or master. 00166 * @param obj The I2C object 00167 * @return non-zero if a value is available 00168 */ 00169 int i2c_slave_read(i2c_t *obj, char *data, int length); 00170 00171 /** Configure I2C as slave or master. 00172 * @param obj The I2C object 00173 * @return non-zero if a value is available 00174 */ 00175 int i2c_slave_write(i2c_t *obj, const char *data, int length); 00176 00177 /** Configure I2C address. 00178 * @param obj The I2C object 00179 * @param idx Currently not used 00180 * @param address The address to be set 00181 * @param mask Currently not used 00182 */ 00183 void i2c_slave_address(i2c_t *obj, int idx, uint32_t address, uint32_t mask); 00184 00185 #endif 00186 00187 /**@}*/ 00188 00189 #if DEVICE_I2C_ASYNCH 00190 00191 /** 00192 * \defgroup hal_AsynchI2C Asynchronous I2C Hardware Abstraction Layer 00193 * @{ 00194 */ 00195 00196 /** Start I2C asynchronous transfer 00197 * 00198 * @param obj The I2C object 00199 * @param tx The transmit buffer 00200 * @param tx_length The number of bytes to transmit 00201 * @param rx The receive buffer 00202 * @param rx_length The number of bytes to receive 00203 * @param address The address to be set - 7bit or 9bit 00204 * @param stop If true, stop will be generated after the transfer is done 00205 * @param handler The I2C IRQ handler to be set 00206 * @param hint DMA hint usage 00207 */ 00208 void i2c_transfer_asynch(i2c_t *obj, const void *tx, size_t tx_length, void *rx, size_t rx_length, uint32_t address, uint32_t stop, uint32_t handler, uint32_t event, DMAUsage hint); 00209 00210 /** The asynchronous IRQ handler 00211 * 00212 * @param obj The I2C object which holds the transfer information 00213 * @return Event flags if a transfer termination condition was met, otherwise return 0. 00214 */ 00215 uint32_t i2c_irq_handler_asynch(i2c_t *obj); 00216 00217 /** Attempts to determine if the I2C peripheral is already in use 00218 * 00219 * @param obj The I2C object 00220 * @return Non-zero if the I2C module is active or zero if it is not 00221 */ 00222 uint8_t i2c_active(i2c_t *obj); 00223 00224 /** Abort asynchronous transfer 00225 * 00226 * This function does not perform any check - that should happen in upper layers. 00227 * @param obj The I2C object 00228 */ 00229 void i2c_abort_asynch(i2c_t *obj); 00230 00231 #endif 00232 00233 /**@}*/ 00234 00235 #ifdef __cplusplus 00236 } 00237 #endif 00238 00239 #endif 00240 00241 #endif
Generated on Tue Jul 12 2022 19:06:15 by
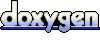