This fork captures the mbed lib v125 for ease of integration into older projects.
Fork of mbed-dev by
gpio_api.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef MBED_GPIO_API_H 00017 #define MBED_GPIO_API_H 00018 00019 #include <stdint.h> 00020 #include "device.h" 00021 00022 #ifdef __cplusplus 00023 extern "C" { 00024 #endif 00025 00026 /** 00027 * \defgroup hal_gpio GPIO HAL functions 00028 * @{ 00029 */ 00030 00031 /** Set the given pin as GPIO 00032 * 00033 * @param pin The pin to be set as GPIO 00034 * @return The GPIO port mask for this pin 00035 **/ 00036 uint32_t gpio_set(PinName pin); 00037 /* Checks if gpio object is connected (pin was not initialized with NC) 00038 * @param pin The pin to be set as GPIO 00039 * @return 0 if port is initialized with NC 00040 **/ 00041 int gpio_is_connected(const gpio_t *obj); 00042 00043 /** Initialize the GPIO pin 00044 * 00045 * @param obj The GPIO object to initialize 00046 * @param pin The GPIO pin to initialize 00047 */ 00048 void gpio_init(gpio_t *obj, PinName pin); 00049 00050 /** Set the input pin mode 00051 * 00052 * @param obj The GPIO object 00053 * @param mode The pin mode to be set 00054 */ 00055 void gpio_mode(gpio_t *obj, PinMode mode); 00056 00057 /** Set the pin direction 00058 * 00059 * @param obj The GPIO object 00060 * @param direction The pin direction to be set 00061 */ 00062 void gpio_dir(gpio_t *obj, PinDirection direction); 00063 00064 /** Set the output value 00065 * 00066 * @param obj The GPIO object 00067 * @param value The value to be set 00068 */ 00069 void gpio_write(gpio_t *obj, int value); 00070 00071 /** Read the input value 00072 * 00073 * @param obj The GPIO object 00074 * @return An integer value 1 or 0 00075 */ 00076 int gpio_read(gpio_t *obj); 00077 00078 // the following functions are generic and implemented in the common gpio.c file 00079 // TODO: fix, will be moved to the common gpio header file 00080 00081 /** Init the input pin and set mode to PullDefault 00082 * 00083 * @param obj The GPIO object 00084 * @param pin The pin name 00085 */ 00086 void gpio_init_in(gpio_t* gpio, PinName pin); 00087 00088 /** Init the input pin and set the mode 00089 * 00090 * @param obj The GPIO object 00091 * @param pin The pin name 00092 * @param mode The pin mode to be set 00093 */ 00094 void gpio_init_in_ex(gpio_t* gpio, PinName pin, PinMode mode); 00095 00096 /** Init the output pin as an output, with predefined output value 0 00097 * 00098 * @param obj The GPIO object 00099 * @param pin The pin name 00100 * @return An integer value 1 or 0 00101 */ 00102 void gpio_init_out(gpio_t* gpio, PinName pin); 00103 00104 /** Init the pin as an output and set the output value 00105 * 00106 * @param obj The GPIO object 00107 * @param pin The pin name 00108 * @param value The value to be set 00109 */ 00110 void gpio_init_out_ex(gpio_t* gpio, PinName pin, int value); 00111 00112 /** Init the pin to be in/out 00113 * 00114 * @param obj The GPIO object 00115 * @param pin The pin name 00116 * @param direction The pin direction to be set 00117 * @param mode The pin mode to be set 00118 * @param value The value to be set for an output pin 00119 */ 00120 void gpio_init_inout(gpio_t* gpio, PinName pin, PinDirection direction, PinMode mode, int value); 00121 00122 /**@}*/ 00123 00124 #ifdef __cplusplus 00125 } 00126 #endif 00127 00128 #endif
Generated on Tue Jul 12 2022 19:06:15 by
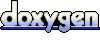