This fork captures the mbed lib v125 for ease of integration into older projects.
Fork of mbed-dev by
Ticker.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef MBED_TICKER_H 00017 #define MBED_TICKER_H 00018 00019 #include "TimerEvent.h" 00020 #include "Callback.h" 00021 00022 namespace mbed { 00023 00024 /** A Ticker is used to call a function at a recurring interval 00025 * 00026 * You can use as many seperate Ticker objects as you require. 00027 * 00028 * @Note Synchronization level: Interrupt safe 00029 * 00030 * Example: 00031 * @code 00032 * // Toggle the blinking led after 5 seconds 00033 * 00034 * #include "mbed.h" 00035 * 00036 * Ticker timer; 00037 * DigitalOut led1(LED1); 00038 * DigitalOut led2(LED2); 00039 * 00040 * int flip = 0; 00041 * 00042 * void attime() { 00043 * flip = !flip; 00044 * } 00045 * 00046 * int main() { 00047 * timer.attach(&attime, 5); 00048 * while(1) { 00049 * if(flip == 0) { 00050 * led1 = !led1; 00051 * } else { 00052 * led2 = !led2; 00053 * } 00054 * wait(0.2); 00055 * } 00056 * } 00057 * @endcode 00058 */ 00059 class Ticker : public TimerEvent { 00060 00061 public: 00062 Ticker() : TimerEvent() { 00063 } 00064 00065 Ticker(const ticker_data_t *data) : TimerEvent(data) { 00066 data->interface->init(); 00067 } 00068 00069 /** Attach a function to be called by the Ticker, specifiying the interval in seconds 00070 * 00071 * @param func pointer to the function to be called 00072 * @param t the time between calls in seconds 00073 */ 00074 void attach(Callback<void()> func, float t) { 00075 attach_us(func, t * 1000000.0f); 00076 } 00077 00078 /** Attach a member function to be called by the Ticker, specifiying the interval in seconds 00079 * 00080 * @param obj pointer to the object to call the member function on 00081 * @param method pointer to the member function to be called 00082 * @param t the time between calls in seconds 00083 */ 00084 template<typename T, typename M> 00085 void attach(T *obj, M method, float t) { 00086 attach(Callback<void()>(obj, method), t); 00087 } 00088 00089 /** Attach a function to be called by the Ticker, specifiying the interval in micro-seconds 00090 * 00091 * @param fptr pointer to the function to be called 00092 * @param t the time between calls in micro-seconds 00093 */ 00094 void attach_us(Callback<void()> func, timestamp_t t) { 00095 _function.attach(func); 00096 setup(t); 00097 } 00098 00099 /** Attach a member function to be called by the Ticker, specifiying the interval in micro-seconds 00100 * 00101 * @param tptr pointer to the object to call the member function on 00102 * @param mptr pointer to the member function to be called 00103 * @param t the time between calls in micro-seconds 00104 */ 00105 template<typename T, typename M> 00106 void attach_us(T *obj, M method, timestamp_t t) { 00107 attach_us(Callback<void()>(obj, method), t); 00108 } 00109 00110 virtual ~Ticker() { 00111 detach(); 00112 } 00113 00114 /** Detach the function 00115 */ 00116 void detach(); 00117 00118 protected: 00119 void setup(timestamp_t t); 00120 virtual void handler(); 00121 00122 protected: 00123 timestamp_t _delay; /**< Time delay (in microseconds) for re-setting the multi-shot callback. */ 00124 Callback<void()> _function; /**< Callback. */ 00125 }; 00126 00127 } // namespace mbed 00128 00129 #endif
Generated on Tue Jul 12 2022 19:06:16 by
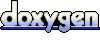