This fork captures the mbed lib v125 for ease of integration into older projects.
Fork of mbed-dev by
SerialBase.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef MBED_SERIALBASE_H 00017 #define MBED_SERIALBASE_H 00018 00019 #include "platform.h" 00020 00021 #if DEVICE_SERIAL 00022 00023 #include "Stream.h" 00024 #include "Callback.h" 00025 #include "serial_api.h" 00026 00027 #if DEVICE_SERIAL_ASYNCH 00028 #include "CThunk.h" 00029 #include "dma_api.h" 00030 #endif 00031 00032 namespace mbed { 00033 00034 /** A base class for serial port implementations 00035 * Can't be instantiated directly (use Serial or RawSerial) 00036 * 00037 * @Note Synchronization level: Set by subclass 00038 */ 00039 class SerialBase { 00040 00041 public: 00042 /** Set the baud rate of the serial port 00043 * 00044 * @param baudrate The baudrate of the serial port (default = 9600). 00045 */ 00046 void baud(int baudrate); 00047 00048 enum Parity { 00049 None = 0, 00050 Odd, 00051 Even, 00052 Forced1, 00053 Forced0 00054 }; 00055 00056 enum IrqType { 00057 RxIrq = 0, 00058 TxIrq 00059 }; 00060 00061 enum Flow { 00062 Disabled = 0, 00063 RTS, 00064 CTS, 00065 RTSCTS 00066 }; 00067 00068 /** Set the transmission format used by the serial port 00069 * 00070 * @param bits The number of bits in a word (5-8; default = 8) 00071 * @param parity The parity used (SerialBase::None, SerialBase::Odd, SerialBase::Even, SerialBase::Forced1, SerialBase::Forced0; default = SerialBase::None) 00072 * @param stop The number of stop bits (1 or 2; default = 1) 00073 */ 00074 void format(int bits=8, Parity parity=SerialBase::None, int stop_bits=1); 00075 00076 /** Determine if there is a character available to read 00077 * 00078 * @returns 00079 * 1 if there is a character available to read, 00080 * 0 otherwise 00081 */ 00082 int readable(); 00083 00084 /** Determine if there is space available to write a character 00085 * 00086 * @returns 00087 * 1 if there is space to write a character, 00088 * 0 otherwise 00089 */ 00090 int writeable(); 00091 00092 /** Attach a function to call whenever a serial interrupt is generated 00093 * 00094 * @param func A pointer to a void function, or 0 to set as none 00095 * @param type Which serial interrupt to attach the member function to (Seriall::RxIrq for receive, TxIrq for transmit buffer empty) 00096 */ 00097 void attach(Callback<void()> func, IrqType type=RxIrq); 00098 00099 /** Attach a member function to call whenever a serial interrupt is generated 00100 * 00101 * @param obj pointer to the object to call the member function on 00102 * @param method pointer to the member function to be called 00103 * @param type Which serial interrupt to attach the member function to (Seriall::RxIrq for receive, TxIrq for transmit buffer empty) 00104 */ 00105 template<typename T> 00106 void attach(T *obj, void (T::*method)(), IrqType type=RxIrq) { 00107 attach(Callback<void()>(obj, method), type); 00108 } 00109 00110 /** Attach a member function to call whenever a serial interrupt is generated 00111 * 00112 * @param obj pointer to the object to call the member function on 00113 * @param method pointer to the member function to be called 00114 * @param type Which serial interrupt to attach the member function to (Seriall::RxIrq for receive, TxIrq for transmit buffer empty) 00115 */ 00116 template<typename T> 00117 void attach(T *obj, void (*method)(T*), IrqType type=RxIrq) { 00118 attach(Callback<void()>(obj, method), type); 00119 } 00120 00121 /** Generate a break condition on the serial line 00122 */ 00123 void send_break(); 00124 00125 protected: 00126 00127 /** Acquire exclusive access to this serial port 00128 */ 00129 virtual void lock(void); 00130 00131 /** Release exclusive access to this serial port 00132 */ 00133 virtual void unlock(void); 00134 00135 public: 00136 00137 #if DEVICE_SERIAL_FC 00138 /** Set the flow control type on the serial port 00139 * 00140 * @param type the flow control type (Disabled, RTS, CTS, RTSCTS) 00141 * @param flow1 the first flow control pin (RTS for RTS or RTSCTS, CTS for CTS) 00142 * @param flow2 the second flow control pin (CTS for RTSCTS) 00143 */ 00144 void set_flow_control(Flow type, PinName flow1=NC, PinName flow2=NC); 00145 #endif 00146 00147 static void _irq_handler(uint32_t id, SerialIrq irq_type); 00148 00149 #if DEVICE_SERIAL_ASYNCH 00150 00151 /** Begin asynchronous write using 8bit buffer. The completition invokes registered TX event callback 00152 * 00153 * @param buffer The buffer where received data will be stored 00154 * @param length The buffer length in bytes 00155 * @param callback The event callback function 00156 * @param event The logical OR of TX events 00157 */ 00158 int write(const uint8_t *buffer, int length, const event_callback_t& callback, int event = SERIAL_EVENT_TX_COMPLETE); 00159 00160 /** Begin asynchronous write using 16bit buffer. The completition invokes registered TX event callback 00161 * 00162 * @param buffer The buffer where received data will be stored 00163 * @param length The buffer length in bytes 00164 * @param callback The event callback function 00165 * @param event The logical OR of TX events 00166 */ 00167 int write(const uint16_t *buffer, int length, const event_callback_t& callback, int event = SERIAL_EVENT_TX_COMPLETE); 00168 00169 /** Abort the on-going write transfer 00170 */ 00171 void abort_write(); 00172 00173 /** Begin asynchronous reading using 8bit buffer. The completition invokes registred RX event callback. 00174 * 00175 * @param buffer The buffer where received data will be stored 00176 * @param length The buffer length in bytes 00177 * @param callback The event callback function 00178 * @param event The logical OR of RX events 00179 * @param char_match The matching character 00180 */ 00181 int read(uint8_t *buffer, int length, const event_callback_t& callback, int event = SERIAL_EVENT_RX_COMPLETE, unsigned char char_match = SERIAL_RESERVED_CHAR_MATCH); 00182 00183 /** Begin asynchronous reading using 16bit buffer. The completition invokes registred RX event callback. 00184 * 00185 * @param buffer The buffer where received data will be stored 00186 * @param length The buffer length in bytes 00187 * @param callback The event callback function 00188 * @param event The logical OR of RX events 00189 * @param char_match The matching character 00190 */ 00191 int read(uint16_t *buffer, int length, const event_callback_t& callback, int event = SERIAL_EVENT_RX_COMPLETE, unsigned char char_match = SERIAL_RESERVED_CHAR_MATCH); 00192 00193 /** Abort the on-going read transfer 00194 */ 00195 void abort_read(); 00196 00197 /** Configure DMA usage suggestion for non-blocking TX transfers 00198 * 00199 * @param usage The usage DMA hint for peripheral 00200 * @return Zero if the usage was set, -1 if a transaction is on-going 00201 */ 00202 int set_dma_usage_tx(DMAUsage usage); 00203 00204 /** Configure DMA usage suggestion for non-blocking RX transfers 00205 * 00206 * @param usage The usage DMA hint for peripheral 00207 * @return Zero if the usage was set, -1 if a transaction is on-going 00208 */ 00209 int set_dma_usage_rx(DMAUsage usage); 00210 00211 protected: 00212 void start_read(void *buffer, int buffer_size, char buffer_width, const event_callback_t& callback, int event, unsigned char char_match); 00213 void start_write(const void *buffer, int buffer_size, char buffer_width, const event_callback_t& callback, int event); 00214 void interrupt_handler_asynch(void); 00215 #endif 00216 00217 protected: 00218 SerialBase(PinName tx, PinName rx); 00219 virtual ~SerialBase() { 00220 } 00221 00222 int _base_getc(); 00223 int _base_putc(int c); 00224 00225 #if DEVICE_SERIAL_ASYNCH 00226 CThunk<SerialBase> _thunk_irq; 00227 event_callback_t _tx_callback; 00228 event_callback_t _rx_callback; 00229 DMAUsage _tx_usage; 00230 DMAUsage _rx_usage; 00231 #endif 00232 00233 serial_t _serial; 00234 Callback<void()> _irq[2]; 00235 int _baud; 00236 00237 }; 00238 00239 } // namespace mbed 00240 00241 #endif 00242 00243 #endif
Generated on Tue Jul 12 2022 19:06:16 by
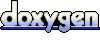