This fork captures the mbed lib v125 for ease of integration into older projects.
Fork of mbed-dev by
PortInOut.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef MBED_PORTINOUT_H 00017 #define MBED_PORTINOUT_H 00018 00019 #include "platform.h" 00020 00021 #if DEVICE_PORTINOUT 00022 00023 #include "port_api.h" 00024 #include "critical.h" 00025 00026 namespace mbed { 00027 00028 /** A multiple pin digital in/out used to set/read multiple bi-directional pins 00029 * 00030 * @Note Synchronization level: Interrupt safe 00031 */ 00032 class PortInOut { 00033 public: 00034 00035 /** Create an PortInOut, connected to the specified port 00036 * 00037 * @param port Port to connect to (Port0-Port5) 00038 * @param mask A bitmask to identify which bits in the port should be included (0 - ignore) 00039 */ 00040 PortInOut(PortName port, int mask = 0xFFFFFFFF) { 00041 core_util_critical_section_enter(); 00042 port_init(&_port, port, mask, PIN_INPUT); 00043 core_util_critical_section_exit(); 00044 } 00045 00046 /** Write the value to the output port 00047 * 00048 * @param value An integer specifying a bit to write for every corresponding port pin 00049 */ 00050 void write(int value) { 00051 port_write(&_port, value); 00052 } 00053 00054 /** Read the value currently output on the port 00055 * 00056 * @returns 00057 * An integer with each bit corresponding to associated port pin setting 00058 */ 00059 int read() { 00060 return port_read(&_port); 00061 } 00062 00063 /** Set as an output 00064 */ 00065 void output() { 00066 core_util_critical_section_enter(); 00067 port_dir(&_port, PIN_OUTPUT); 00068 core_util_critical_section_exit(); 00069 } 00070 00071 /** Set as an input 00072 */ 00073 void input() { 00074 core_util_critical_section_enter(); 00075 port_dir(&_port, PIN_INPUT); 00076 core_util_critical_section_exit(); 00077 } 00078 00079 /** Set the input pin mode 00080 * 00081 * @param mode PullUp, PullDown, PullNone, OpenDrain 00082 */ 00083 void mode(PinMode mode) { 00084 core_util_critical_section_enter(); 00085 port_mode(&_port, mode); 00086 core_util_critical_section_exit(); 00087 } 00088 00089 /** A shorthand for write() 00090 */ 00091 PortInOut& operator= (int value) { 00092 write(value); 00093 return *this; 00094 } 00095 00096 PortInOut& operator= (PortInOut& rhs) { 00097 write(rhs.read()); 00098 return *this; 00099 } 00100 00101 /** A shorthand for read() 00102 */ 00103 operator int() { 00104 return read(); 00105 } 00106 00107 private: 00108 port_t _port; 00109 }; 00110 00111 } // namespace mbed 00112 00113 #endif 00114 00115 #endif
Generated on Tue Jul 12 2022 19:06:16 by
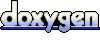