This fork captures the mbed lib v125 for ease of integration into older projects.
Fork of mbed-dev by
DigitalOut.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef MBED_DIGITALOUT_H 00017 #define MBED_DIGITALOUT_H 00018 00019 #include "platform.h" 00020 #include "gpio_api.h" 00021 #include "critical.h" 00022 00023 namespace mbed { 00024 00025 /** A digital output, used for setting the state of a pin 00026 * 00027 * @Note Synchronization level: Interrupt safe 00028 * 00029 * Example: 00030 * @code 00031 * // Toggle a LED 00032 * #include "mbed.h" 00033 * 00034 * DigitalOut led(LED1); 00035 * 00036 * int main() { 00037 * while(1) { 00038 * led = !led; 00039 * wait(0.2); 00040 * } 00041 * } 00042 * @endcode 00043 */ 00044 class DigitalOut { 00045 00046 public: 00047 /** Create a DigitalOut connected to the specified pin 00048 * 00049 * @param pin DigitalOut pin to connect to 00050 */ 00051 DigitalOut(PinName pin) : gpio() { 00052 // No lock needed in the constructor 00053 gpio_init_out(&gpio, pin); 00054 } 00055 00056 /** Create a DigitalOut connected to the specified pin 00057 * 00058 * @param pin DigitalOut pin to connect to 00059 * @param value the initial pin value 00060 */ 00061 DigitalOut(PinName pin, int value) : gpio() { 00062 // No lock needed in the constructor 00063 gpio_init_out_ex(&gpio, pin, value); 00064 } 00065 00066 /** Set the output, specified as 0 or 1 (int) 00067 * 00068 * @param value An integer specifying the pin output value, 00069 * 0 for logical 0, 1 (or any other non-zero value) for logical 1 00070 */ 00071 void write(int value) { 00072 // Thread safe / atomic HAL call 00073 gpio_write(&gpio, value); 00074 } 00075 00076 /** Return the output setting, represented as 0 or 1 (int) 00077 * 00078 * @returns 00079 * an integer representing the output setting of the pin, 00080 * 0 for logical 0, 1 for logical 1 00081 */ 00082 int read() { 00083 // Thread safe / atomic HAL call 00084 return gpio_read(&gpio); 00085 } 00086 00087 /** Return the output setting, represented as 0 or 1 (int) 00088 * 00089 * @returns 00090 * Non zero value if pin is connected to uc GPIO 00091 * 0 if gpio object was initialized with NC 00092 */ 00093 int is_connected() { 00094 // Thread safe / atomic HAL call 00095 return gpio_is_connected(&gpio); 00096 } 00097 00098 /** A shorthand for write() 00099 */ 00100 DigitalOut& operator= (int value) { 00101 // Underlying write is thread safe 00102 write(value); 00103 return *this; 00104 } 00105 00106 DigitalOut& operator= (DigitalOut& rhs) { 00107 core_util_critical_section_enter(); 00108 write(rhs.read()); 00109 core_util_critical_section_exit(); 00110 return *this; 00111 } 00112 00113 /** A shorthand for read() 00114 */ 00115 operator int() { 00116 // Underlying call is thread safe 00117 return read(); 00118 } 00119 00120 protected: 00121 gpio_t gpio; 00122 }; 00123 00124 } // namespace mbed 00125 00126 #endif
Generated on Tue Jul 12 2022 19:06:15 by
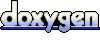