
A implementation of a simple bomberman game
Dependencies: 4DGL-uLCD-SE SDFileSystem mbed-rtos mbed wave_player
Fork of rtos_basic by
navSwitch.h
00001 class Nav_Switch 00002 { 00003 public: 00004 Nav_Switch(PinName up,PinName down,PinName left,PinName right,PinName fire); 00005 int read(); 00006 //boolean functions to test each switch 00007 bool up(); 00008 bool down(); 00009 bool left(); 00010 bool right(); 00011 bool fire(); 00012 //automatic read on RHS 00013 operator int (); 00014 //index to any switch array style 00015 bool operator[](int index) { 00016 return _pins[index]; 00017 }; 00018 private: 00019 BusIn _pins; 00020 }; 00021 00022 Nav_Switch::Nav_Switch (PinName up,PinName down,PinName left,PinName right,PinName fire): 00023 _pins(up, down, left, right, fire) 00024 { 00025 _pins.mode(PullUp); //needed if pullups not on board or a bare nav switch is used - delete otherwise 00026 wait(0.001); //delays just a bit for pullups to pull inputs high 00027 } 00028 inline bool Nav_Switch::up() 00029 { 00030 return !(_pins[0]); 00031 } 00032 inline bool Nav_Switch::down() 00033 { 00034 return !(_pins[1]); 00035 } 00036 inline bool Nav_Switch::left() 00037 { 00038 return !(_pins[2]); 00039 } 00040 inline bool Nav_Switch::right() 00041 { 00042 return !(_pins[3]); 00043 } 00044 inline bool Nav_Switch::fire() 00045 { 00046 return !(_pins[4]); 00047 } 00048 inline int Nav_Switch::read() 00049 { 00050 return _pins.read(); 00051 } 00052 inline Nav_Switch::operator int () 00053 { 00054 return _pins.read(); 00055 }
Generated on Thu Jul 14 2022 17:13:56 by
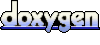