
mbedConnectorInterface sample endpoint utilizing 3g cellular radio as the underlying connection transport.
Dependencies: LM75B mbed mbedConnectorInterface mbedEndpointNetwork_Ublox
main.cpp
00001 /** 00002 * @file main.cpp 00003 * @brief mbed Connected Home Endpoint main entry point 00004 * @author Doug Anson 00005 * @version 1.0 00006 * @see 00007 * 00008 * Copyright (c) 2014 00009 * 00010 * Licensed under the Apache License, Version 2.0 (the "License"); 00011 * you may not use this file except in compliance with the License. 00012 * You may obtain a copy of the License at 00013 * 00014 * http://www.apache.org/licenses/LICENSE-2.0 00015 * 00016 * Unless required by applicable law or agreed to in writing, software 00017 * distributed under the License is distributed on an "AS IS" BASIS, 00018 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00019 * See the License for the specific language governing permissions and 00020 * limitations under the License. 00021 */ 00022 00023 // mbed Connector Interface (configuration) 00024 #include "mbedConnectorInterface.h" 00025 00026 // mbed Endpoint Network 00027 #include "mbedEndpointNetwork.h" 00028 00029 // Static Resources 00030 #include "StaticResource.h" 00031 StaticResource mfg(&logger,"dev/mfg","u-blox"); 00032 StaticResource model(&logger,"dev/mdl","mbed cellular endpoint"); 00033 00034 // 00035 // Dynamic Resource Note: 00036 // 00037 // mbedConnectorInterface supports up to IPT_MAX_ENTRIES 00038 // (currently 5) independent dynamic resources. 00039 // 00040 // You can increase this (at the cost of memory) 00041 // in mbedConnectorinterface.h 00042 // 00043 00044 // Sample Dynamic Resource 00045 #include "SampleDynamicResource.h" 00046 SampleDynamicResource sample(&logger,"999/999/9999"); 00047 00048 // Light Resource 00049 #include "LightResource.h" 00050 LightResource light(&logger,"311/0/5850"); 00051 00052 // Temperature Resource 00053 #include "TemperatureResource.h" 00054 TemperatureResource temperature(&logger,"303/0/5700",true); 00055 00056 // Location Resource 00057 #include "LocationResource.h" 00058 LocationResource location(&logger,"999/0/1234",true); 00059 00060 // Set our own unique endpoint name 00061 #define MY_ENDPOINT_NAME "mbed-3g-sample" 00062 00063 // My NSP Domain 00064 #define MY_NSP_DOMAIN "domain" 00065 00066 // Customization Example: My custom NSP IPv4 address and NSP CoAP port 00067 uint8_t my_nsp_address[NSP_IP_ADDRESS_LENGTH] = {23,99,29,171}; // connector (api.connector.mbed.org) 00068 int my_nsp_coap_port = 5683; 00069 00070 // called from the Endpoint::start() below to create resources and the endpoint internals... 00071 Connector::Options *configure_endpoint(Connector::OptionsBuilder &config) 00072 { 00073 // Build the endpoint configuration parameters 00074 logger.log("configure_endpoint: building endpoint configuration..."); 00075 return config.setEndpointNodename(MY_ENDPOINT_NAME) // custom endpoint name 00076 .setNSPAddress(my_nsp_address) // custom NSP address 00077 .setDomain(MY_NSP_DOMAIN) // custom NSP domain 00078 .setNSPPortNumber(my_nsp_coap_port) // custom NSP CoAP port 00079 00080 // enable or disable(default) immediate observationing control 00081 .setImmedateObservationEnabled(true) 00082 00083 // enable or disable(default) GET-based observation control 00084 .setEnableGETObservationControl(false) 00085 00086 // add the static resource representing this endpoint 00087 .addResource(&mfg) 00088 .addResource(&model) 00089 00090 // add a Sample Dynamic Resource 00091 .addResource(&sample) 00092 00093 // Add my specific physical dynamic resources... 00094 .addResource(&light) 00095 .addResource(&temperature,10000) // observe every 10 seconds 00096 .addResource(&location,30000) // observe every 30 seconds 00097 00098 // finalize the configuration... 00099 .build(); 00100 } 00101 00102 // main entry point... 00103 int main() 00104 { 00105 // Announce 00106 logger.log("\r\n\r\nmbed mDS Sample Endpoint v1.0 (3G Cellular)"); 00107 00108 // we have to plumb our network first 00109 Connector::Endpoint::plumbNetwork(); 00110 00111 // starts the endpoint by finalizing its configuration (configure_endpoint() above called),creating a Thread and reading NSP events... 00112 logger.log("Start the endpoint to finish setup and enter the main loop..."); 00113 Connector::Endpoint::start(); 00114 }
Generated on Sat Jul 30 2022 21:39:30 by
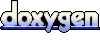