Error Handler/Logger construct for mbed applications
Embed:
(wiki syntax)
Show/hide line numbers
ErrorHandler.h
00001 /* Copyright C2014 ARM, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files the "Software", to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #ifndef _ERROR_HANDLER_H_ 00020 #define _ERROR_HANDLER_H_ 00021 00022 // our definitions 00023 #include "Definitions.h" 00024 00025 // biggest log message length 00026 #define MAX_LOG_MESSAGE_PREFIX 128 00027 #define MAX_LOG_MESSAGE (MAX_BUFFER_LENGTH+MAX_LOG_MESSAGE_PREFIX+1) 00028 00029 // Support for varargs 00030 #include <stdarg.h> 00031 00032 // LCD Support 00033 #if _UBLOX_PLATFORM 00034 #include "C12832.h" 00035 #define LCDCLASS C12832 00036 #endif 00037 00038 #if _NXP_PLATFORM 00039 #include "C12832_lcd.h" 00040 #define LCDCLASS C12832_LCD 00041 #endif 00042 00043 #if _K64F_PLATFORM 00044 #include "C12832.h" 00045 #define LCDCLASS C12832 // not used 00046 #endif 00047 00048 /** 00049 ErrorHandler 00050 Error handling class for mbed endpoints 00051 */ 00052 class ErrorHandler { 00053 private: 00054 RawSerial *m_pc; 00055 LCDCLASS *m_lcd; 00056 char m_message[MAX_LOG_MESSAGE+1]; 00057 00058 public: 00059 /** 00060 Default constructor 00061 @param pc RawSerial instance 00062 @param lcd LCDCLASS instance (or NULL) 00063 */ 00064 ErrorHandler(RawSerial *pc,LCDCLASS *lcd); 00065 00066 /** 00067 Default destructor 00068 */ 00069 virtual ~ErrorHandler(); 00070 00071 /** 00072 log message to the serial console and LCD (if enabled) 00073 @param format variable argument format 00074 */ 00075 void log(const char *format, ...); 00076 00077 /** 00078 log message to the serial console only 00079 @param format variable argument format 00080 */ 00081 void logConsole(const char *format, ...); 00082 00083 /** 00084 turn the multi-colored LED red 00085 */ 00086 void turnLEDRed(); 00087 00088 /** 00089 turn the multi-colored LED green 00090 */ 00091 void turnLEDGreen(); 00092 00093 /** 00094 turn the multi-colored LED blue 00095 */ 00096 void turnLEDBlue(); 00097 00098 /** 00099 turn the multi-colored LED purple 00100 */ 00101 void turnLEDPurple(); 00102 00103 /** 00104 turn the multi-colored LED black 00105 */ 00106 void turnLEDBlack(); 00107 00108 /** 00109 turn the multi-colored LED yellow 00110 */ 00111 void turnLEDYellow(); 00112 00113 /** 00114 turn the multi-colored LED orange 00115 */ 00116 void turnLEDOrange(); 00117 00118 /** 00119 blink the transmit LED 00120 */ 00121 void blinkTransportTxLED(); 00122 00123 /** 00124 blink the receiver LED 00125 */ 00126 void blinkTransportRxLED(); 00127 00128 private: 00129 void setRGBLED(float H, float S, float V); 00130 void blinkLED(DigitalOut led); 00131 }; 00132 00133 #endif // _ERROR_HANDLER_H_
Generated on Mon Jul 18 2022 12:20:31 by
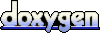