
BLE GATT Button on Board Example Nucleo IDB0XA1
Dependencies: BLE_API X_NUCLEO_IDB0XA1 mbed
Fork of BLE_GAP_Example by
main.cpp
00001 #include "mbed.h" 00002 #include "ble/BLE.h" 00003 #include "ble/Gap.h" 00004 #include "ble/services/BatteryService.h" 00005 #include "ble/services/DeviceInformationService.h" 00006 00007 #define BUTTON_SERVICE_UUID_0 0x0000 // Custom SERVICE_UUID 00008 #define BUTTON_SERVICE_UUID_1 0x0000 // Custom SERVICE_UUID 00009 #define BUTTON_SERVICE_UUID_2 0x0000 // Custom SERVICE_UUID 00010 #define BUTTON_SERVICE_UUID_3 0x0000 // Custom SERVICE_UUID 00011 #define BUTTON_SERVICE_UUID_4 0x0000 // Custom SERVICE_UUID 00012 #define BUTTON_SERVICE_UUID_5 0x0000 // Custom SERVICE_UUID 00013 #define BUTTON_SERVICE_UUID_6 0x0000 // Custom SERVICE_UUID 00014 #define BUTTON_SERVICE_UUID_7 0xFFF0 // Custom SERVICE_UUID 00015 00016 #define BUTTON_STATE_CHARACTERISTIC_UUID_0 0x0000 // Custom CHARACTERISTIC_UUID 00017 #define BUTTON_STATE_CHARACTERISTIC_UUID_1 0x0000 // Custom CHARACTERISTIC_UUID 00018 #define BUTTON_STATE_CHARACTERISTIC_UUID_2 0x0000 // Custom CHARACTERISTIC_UUID 00019 #define BUTTON_STATE_CHARACTERISTIC_UUID_3 0x0000 // Custom CHARACTERISTIC_UUID 00020 #define BUTTON_STATE_CHARACTERISTIC_UUID_4 0x0000 // Custom CHARACTERISTIC_UUID 00021 #define BUTTON_STATE_CHARACTERISTIC_UUID_5 0x0000 // Custom CHARACTERISTIC_UUID 00022 #define BUTTON_STATE_CHARACTERISTIC_UUID_6 0x0000 // Custom CHARACTERISTIC_UUID 00023 #define BUTTON_STATE_CHARACTERISTIC_UUID_7 0xFFF1 // Custom CHARACTERISTIC_UUID 00024 00025 BLE ble; 00026 00027 DigitalOut led1(LED1); 00028 InterruptIn int_external(PC_13); 00029 DigitalIn Push_Button(PC_13,PullNone); 00030 00031 static const char DEVICE_NAME[] = "CESA BLE 4.0 Button"; 00032 //static const uint16_t service_uuid16_list[] = {BUTTON_SERVICE_UUID_7}; 00033 static const uint16_t service_uuid128_list[] = {BUTTON_SERVICE_UUID_7}; 00034 static const uint16_t characteristic_uuid128_list[] = {BUTTON_STATE_CHARACTERISTIC_UUID_7}; 00035 00036 bool buttonPressed = false; 00037 00038 //buttonState is accessible to button callbacks(CHARACTERISTIC_UUID) 00039 ReadOnlyGattCharacteristic<bool> buttonState((uint8_t *)characteristic_uuid128_list,&buttonPressed,GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ); 00040 00041 00042 void buttonPressedCallback(void) 00043 { 00044 buttonPressed = true; 00045 ble.updateCharacteristicValue(buttonState.getValueHandle(),(uint8_t *)&buttonPressed, sizeof(bool)); 00046 } 00047 00048 void buttonReleasedCallback(void) 00049 { 00050 buttonPressed = false; 00051 ble.updateCharacteristicValue(buttonState.getValueHandle(),(uint8_t *)&buttonPressed, sizeof(bool)); 00052 } 00053 00054 void disconnectionCallback(const Gap::DisconnectionCallbackParams_t *params) 00055 { 00056 ble.startAdvertising(); 00057 } 00058 00059 void periodicCallback(void) 00060 { 00061 //led1 = !led1; /* Do blinky on LED1 to indicate system aliveness. */ 00062 } 00063 00064 int main(void) 00065 { 00066 led1 = 1; 00067 Ticker ticker; 00068 ticker.attach(periodicCallback, 1); 00069 int_external.fall(&buttonPressedCallback); 00070 int_external.rise(&buttonReleasedCallback); 00071 00072 ble.init(); 00073 ble.onDisconnection(disconnectionCallback); 00074 00075 GattCharacteristic *charTable[] = {&buttonState}; 00076 GattService buttonService((uint8_t *)service_uuid128_list, charTable, sizeof(charTable) / sizeof(GattCharacteristic *)); 00077 ble.addService(buttonService); 00078 00079 /* setup advertising */ 00080 ble.accumulateAdvertisingPayload(GapAdvertisingData::BREDR_NOT_SUPPORTED | GapAdvertisingData::LE_GENERAL_DISCOVERABLE); 00081 //ble.accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LIST_16BIT_SERVICE_IDS,(uint8_t *)service_uuid16_list, sizeof(service_uuid16_list)); 00082 ble.accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LIST_128BIT_SERVICE_IDS,(uint8_t *)service_uuid128_list, sizeof(service_uuid128_list)); 00083 ble.accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LOCAL_NAME,(uint8_t *)DEVICE_NAME, sizeof(DEVICE_NAME)); 00084 ble.setAdvertisingType(GapAdvertisingParams::ADV_CONNECTABLE_UNDIRECTED); 00085 00086 ble.setAdvertisingInterval(100); /* 100 ms. */ 00087 ble.startAdvertising(); 00088 00089 while (true) 00090 { 00091 ble.waitForEvent(); 00092 } 00093 }
Generated on Wed Jul 13 2022 08:46:18 by
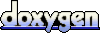