
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 00003 PwmOut myled(LED1); 00004 00005 bool back = false; 00006 float drive = 0; 00007 00008 Timer timer; 00009 00010 int main() 00011 { 00012 int start = 0; 00013 int current = 0; 00014 00015 timer.start(); 00016 00017 myled = 1.0; 00018 00019 while(1) 00020 { 00021 current = timer.read_ms(); 00022 00023 if((current - start) >= 10) 00024 { 00025 if(back) 00026 { 00027 drive -= 0.01; 00028 00029 if(drive <= 0) 00030 { 00031 drive = 0; 00032 back = false; 00033 } 00034 } 00035 else 00036 { 00037 drive += 0.01; 00038 00039 if(drive >= 1) 00040 { 00041 drive = 0.999; 00042 back = true; 00043 } 00044 } 00045 00046 myled = drive; 00047 start = timer.read_ms(); 00048 } 00049 } 00050 }
Generated on Sat Jul 23 2022 21:23:52 by
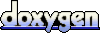