
Bluetooth enabled control of a BLDC via the Allegro MicroSystems A4960.
Dependencies: BLE_API mbed nRF51822
Fork of BLE_ModularRobot by
spi_master.cpp
00001 /* 00002 00003 Copyright (c) 2012-2014 RedBearLab 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00006 and associated documentation files (the "Software"), to deal in the Software without restriction, 00007 including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, 00009 subject to the following conditions: 00010 The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. 00011 00012 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, 00013 INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR 00014 PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE 00015 FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00016 ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 00018 */ 00019 00020 #include "spi_master.h" 00021 00022 /********************************************************************** 00023 name : 00024 function : 00025 **********************************************************************/ 00026 SPIClass::SPIClass(NRF_SPI_Type *_spi) : spi(_spi) 00027 { 00028 //do nothing 00029 } 00030 00031 /********************************************************************** 00032 name : 00033 function : 00034 **********************************************************************/ 00035 void SPIClass::setCPOL( bool active_low) 00036 { 00037 if(active_low) 00038 { 00039 spi->CONFIG |= (SPI_CONFIG_CPOL_ActiveLow << SPI_CONFIG_CPOL_Pos); 00040 } 00041 else 00042 { 00043 spi->CONFIG |= (SPI_CONFIG_CPOL_ActiveHigh << SPI_CONFIG_CPOL_Pos); 00044 } 00045 } 00046 00047 /********************************************************************** 00048 name : 00049 function : 00050 **********************************************************************/ 00051 void SPIClass::setCPHA( bool trailing) 00052 { 00053 if(trailing) 00054 { 00055 spi->CONFIG |= (SPI_CONFIG_CPHA_Trailing << SPI_CONFIG_CPHA_Pos); 00056 } 00057 else 00058 { 00059 spi->CONFIG |= (SPI_CONFIG_CPHA_Leading << SPI_CONFIG_CPHA_Pos); 00060 } 00061 00062 } 00063 00064 /********************************************************************** 00065 name : 00066 function : MSBFIRST, LSBFIRST 00067 **********************************************************************/ 00068 void SPIClass::setBitORDER( BitOrder bit) 00069 { 00070 if(bit == MSBFIRST) 00071 { 00072 spi->CONFIG |= (SPI_CONFIG_ORDER_MsbFirst << SPI_CONFIG_ORDER_Pos); 00073 } 00074 else 00075 { 00076 spi->CONFIG |= (SPI_CONFIG_ORDER_LsbFirst << SPI_CONFIG_ORDER_Pos); 00077 } 00078 } 00079 00080 /********************************************************************** 00081 name : 00082 function : 00083 **********************************************************************/ 00084 void SPIClass::setFrequency(uint8_t speed) 00085 { 00086 if (speed == 0) 00087 { 00088 spi->FREQUENCY = SPI_FREQUENCY_125K; 00089 } 00090 else if (speed == 1) 00091 { 00092 spi->FREQUENCY = SPI_FREQUENCY_250K; 00093 } 00094 else if (speed == 2) 00095 { 00096 spi->FREQUENCY = SPI_FREQUENCY_500K; 00097 } 00098 else if (speed == 3) 00099 { 00100 spi->FREQUENCY = SPI_FREQUENCY_1M; 00101 } 00102 else if (speed == 4) 00103 { 00104 spi->FREQUENCY = SPI_FREQUENCY_2M; 00105 } 00106 else if (speed == 5) 00107 { 00108 spi->FREQUENCY = SPI_FREQUENCY_4M; 00109 } 00110 else if (speed == 6) 00111 { 00112 spi->FREQUENCY = SPI_FREQUENCY_8M; 00113 } 00114 else 00115 { 00116 spi->FREQUENCY = SPI_FREQUENCY_4M; 00117 } 00118 } 00119 00120 /********************************************************************** 00121 name : 00122 function : 00123 **********************************************************************/ 00124 void SPIClass::setSPIMode( uint8_t mode ) 00125 { 00126 if (SPI_MODE0 == mode) 00127 { 00128 setCPOL(0); 00129 setCPHA(0); 00130 } 00131 else if (mode == SPI_MODE1) 00132 { 00133 setCPOL(0); 00134 setCPHA(1); 00135 } 00136 else if (mode == SPI_MODE2) 00137 { 00138 setCPOL(1); 00139 setCPHA(0); 00140 } 00141 else if (mode == SPI_MODE3) 00142 { 00143 setCPOL(1); 00144 setCPHA(1); 00145 } 00146 else 00147 { 00148 setCPOL(0); 00149 setCPHA(0); 00150 } 00151 } 00152 00153 /********************************************************************** 00154 name : 00155 function : 00156 **********************************************************************/ 00157 void SPIClass::begin(uint32_t sck, uint32_t mosi, uint32_t miso) 00158 { 00159 00160 SCK_Pin = sck; 00161 MOSI_Pin = mosi; 00162 MISO_Pin = miso; 00163 00164 NRF_GPIO->PIN_CNF[SCK_Pin] = (GPIO_PIN_CNF_SENSE_Disabled << GPIO_PIN_CNF_SENSE_Pos) 00165 | (GPIO_PIN_CNF_DRIVE_S0S1 << GPIO_PIN_CNF_DRIVE_Pos) 00166 | (GPIO_PIN_CNF_PULL_Disabled << GPIO_PIN_CNF_PULL_Pos) 00167 | (GPIO_PIN_CNF_INPUT_Connect << GPIO_PIN_CNF_INPUT_Pos) 00168 | (GPIO_PIN_CNF_DIR_Output << GPIO_PIN_CNF_DIR_Pos); 00169 00170 NRF_GPIO->PIN_CNF[MOSI_Pin] = (GPIO_PIN_CNF_SENSE_Disabled << GPIO_PIN_CNF_SENSE_Pos) 00171 | (GPIO_PIN_CNF_DRIVE_S0S1 << GPIO_PIN_CNF_DRIVE_Pos) 00172 | (GPIO_PIN_CNF_PULL_Disabled << GPIO_PIN_CNF_PULL_Pos) 00173 | (GPIO_PIN_CNF_INPUT_Connect << GPIO_PIN_CNF_INPUT_Pos) 00174 | (GPIO_PIN_CNF_DIR_Output << GPIO_PIN_CNF_DIR_Pos); 00175 00176 NRF_GPIO->PIN_CNF[MISO_Pin] = (GPIO_PIN_CNF_SENSE_Disabled << GPIO_PIN_CNF_SENSE_Pos) 00177 | (GPIO_PIN_CNF_DRIVE_S0S1 << GPIO_PIN_CNF_DRIVE_Pos) 00178 | (GPIO_PIN_CNF_PULL_Disabled << GPIO_PIN_CNF_PULL_Pos) 00179 | (GPIO_PIN_CNF_INPUT_Connect << GPIO_PIN_CNF_INPUT_Pos) 00180 | (GPIO_PIN_CNF_DIR_Input << GPIO_PIN_CNF_DIR_Pos); 00181 spi->PSELSCK = SCK_Pin; 00182 spi->PSELMOSI = MOSI_Pin; 00183 spi->PSELMISO = MISO_Pin; 00184 00185 setFrequency(SPI_1M); 00186 setSPIMode(SPI_MODE3); 00187 setBitORDER(MSBFIRST); 00188 00189 spi->EVENTS_READY = 0; 00190 spi->ENABLE = (SPI_ENABLE_ENABLE_Enabled << SPI_ENABLE_ENABLE_Pos); 00191 00192 } 00193 00194 /********************************************************************** 00195 name : 00196 function : 00197 **********************************************************************/ 00198 void SPIClass::begin() 00199 { 00200 begin(SCK, MOSI, MISO); 00201 } 00202 00203 /********************************************************************** 00204 name : 00205 function : 00206 **********************************************************************/ 00207 uint8_t SPIClass::transfer(uint8_t data) 00208 { 00209 while( spi->EVENTS_READY != 0U ); 00210 00211 spi->TXD = (uint32_t)data; 00212 00213 while(spi->EVENTS_READY == 0); 00214 00215 data = (uint8_t)spi->RXD; 00216 00217 spi->EVENTS_READY = 0; 00218 00219 return data; 00220 } 00221 00222 /********************************************************************** 00223 name : 00224 function : 00225 **********************************************************************/ 00226 void SPIClass::endTransfer() 00227 { 00228 spi->ENABLE = (SPI_ENABLE_ENABLE_Disabled << SPI_ENABLE_ENABLE_Pos); 00229 } 00230 00231 /********************************************************************** 00232 name : 00233 function : 00234 **********************************************************************/ 00235 00236 //SPIClass SPI(NRF_SPI1);
Generated on Tue Jul 19 2022 06:45:22 by
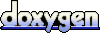