
test public
Dependencies: HttpServer_snapshot_mbed-os
USBHostHub.h
00001 /* mbed USBHost Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef USBHOSTHUB_H 00018 #define USBHOSTHUB_H 00019 00020 #include "USBHostConf.h" 00021 00022 #if MAX_HUB_NB 00023 00024 #include "USBHostTypes.h" 00025 #include "IUSBEnumerator.h" 00026 00027 class USBHost; 00028 class USBDeviceConnected; 00029 class USBEndpoint; 00030 00031 /** 00032 * A class to use a USB Hub 00033 */ 00034 class USBHostHub : public IUSBEnumerator { 00035 public: 00036 /** 00037 * Constructor 00038 */ 00039 USBHostHub(); 00040 #if defined(TARGET_RZ_A2XX) 00041 ~USBHostHub(); 00042 #endif 00043 00044 /** 00045 * Check if a USB Hub is connected 00046 * 00047 * @return true if a serial device is connected 00048 */ 00049 bool connected(); 00050 00051 /** 00052 * Try to connect device 00053 * 00054 * @param dev device to connect 00055 * @return true if connection was successful 00056 */ 00057 bool connect(USBDeviceConnected * dev); 00058 00059 /** 00060 * Automatically called by USBHost when a device 00061 * has been enumerated by usb_thread 00062 * 00063 * @param dev device connected 00064 */ 00065 void deviceConnected(USBDeviceConnected * dev); 00066 00067 /** 00068 * Automatically called by USBHost when a device 00069 * has been disconnected from this hub 00070 * 00071 * @param dev device disconnected 00072 */ 00073 void deviceDisconnected(USBDeviceConnected * dev); 00074 00075 /** 00076 * Rest a specific port 00077 * 00078 * @param port port number 00079 */ 00080 void portReset(uint8_t port); 00081 00082 /* 00083 * Called by USBHost to set the instance of USBHost 00084 * 00085 * @param host host instance 00086 */ 00087 void setHost(USBHost * host); 00088 00089 /** 00090 * Called by USBhost when a hub has been disconnected 00091 */ 00092 void hubDisconnected(); 00093 00094 protected: 00095 //From IUSBEnumerator 00096 virtual void setVidPid(uint16_t vid, uint16_t pid); 00097 virtual bool parseInterface(uint8_t intf_nb, uint8_t intf_class, uint8_t intf_subclass, uint8_t intf_protocol); //Must return true if the interface should be parsed 00098 virtual bool useEndpoint(uint8_t intf_nb, ENDPOINT_TYPE type, ENDPOINT_DIRECTION dir); //Must return true if the endpoint will be used 00099 00100 private: 00101 USBHost * host; 00102 USBDeviceConnected * dev; 00103 bool dev_connected; 00104 USBEndpoint * int_in; 00105 uint8_t nb_port; 00106 uint8_t hub_characteristics; 00107 00108 void rxHandler(); 00109 00110 #if defined(TARGET_RZ_A2XX) 00111 uint8_t * buf; 00112 uint32_t * p_st; 00113 #else 00114 uint8_t buf[sizeof(HubDescriptor)]; 00115 #endif 00116 00117 int hub_intf; 00118 bool hub_device_found; 00119 00120 void setPortFeature(uint32_t feature, uint8_t port); 00121 void clearPortFeature(uint32_t feature, uint8_t port); 00122 uint32_t getPortStatus(uint8_t port); 00123 00124 USBDeviceConnected * device_children[MAX_HUB_PORT]; 00125 00126 void init(); 00127 void disconnect(); 00128 00129 }; 00130 00131 #endif 00132 00133 #endif
Generated on Wed Jul 13 2022 05:33:37 by
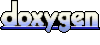