
test public
Dependencies: HttpServer_snapshot_mbed-os
TouchKey_7_1inch.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (C) 2016 Renesas Electronics Corporation. All rights reserved. 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "TouchKey_7_1inch.h" 00018 00019 TouchKey_7_1inch::TouchKey_7_1inch(PinName tprst, PinName tpint, PinName sda, PinName scl) : 00020 TouchKey(tprst, tpint), i2c(sda, scl) { 00021 } 00022 00023 int TouchKey_7_1inch::GetMaxTouchNum(void) { 00024 return 2; 00025 } 00026 00027 int TouchKey_7_1inch::GetCoordinates(int touch_buff_num, touch_pos_t * p_touch) { 00028 char buf[10]; 00029 stx_report_data_t *pdata; 00030 touch_pos_t * wk_touch; 00031 int count = 0; 00032 int i; 00033 int read_size; 00034 00035 if (touch_buff_num > GetMaxTouchNum()) { 00036 touch_buff_num = GetMaxTouchNum(); 00037 } 00038 if (touch_buff_num < 2) { 00039 read_size = 6; 00040 } else { 00041 read_size = 10; 00042 } 00043 00044 if (p_touch != NULL) { 00045 for (i = 0; i < touch_buff_num; i++) { 00046 wk_touch = &p_touch[i]; 00047 wk_touch->x = 0; 00048 wk_touch->y = 0; 00049 wk_touch->valid = false; 00050 } 00051 if (i2c.read((0x55 << 1), buf, read_size) == 0) { 00052 pdata = (stx_report_data_t *)buf; 00053 if (pdata->fingers) { 00054 for (i = 0; i < touch_buff_num; i++) { 00055 if (pdata->xyz_data[i].valid) { 00056 wk_touch = &p_touch[i]; 00057 wk_touch->x = (pdata->xyz_data[i].x_h << 8) | pdata->xyz_data[i].x_l; 00058 wk_touch->y = (pdata->xyz_data[i].y_h << 8) | pdata->xyz_data[i].y_l; 00059 wk_touch->valid = true; 00060 count++; 00061 } 00062 } 00063 } 00064 } 00065 } 00066 00067 return count; 00068 } 00069 00070
Generated on Wed Jul 13 2022 05:33:37 by
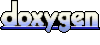