
test public
Dependencies: HttpServer_snapshot_mbed-os
TouchKey.h
00001 /* mbed Microcontroller Library 00002 * Copyright (C) 2016 Renesas Electronics Corporation. All rights reserved. 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 /**************************************************************************//** 00017 * @file TouchKey.h 00018 * @brief TouchKey API 00019 ******************************************************************************/ 00020 00021 #ifndef TOUCH_KEY_H 00022 #define TOUCH_KEY_H 00023 00024 #include "mbed.h" 00025 00026 /** 00027 * The class to acquire touch coordinates 00028 */ 00029 class TouchKey { 00030 00031 public: 00032 /** Touch position structure */ 00033 typedef struct { 00034 uint32_t x; /**< The position of the x-coordinate. */ 00035 uint32_t y; /**< The position of the y-coordinate. */ 00036 bool valid; /**< Whether a valid data.. */ 00037 } touch_pos_t; 00038 00039 00040 /** Create a TouchKey object 00041 * 00042 * @param tprst tprst pin 00043 * @param tpint tpint pin 00044 */ 00045 TouchKey(PinName tprst, PinName tpint) : touch_int(tpint) { 00046 if (tprst != NC) { 00047 p_touch_reset = new DigitalOut(tprst); 00048 } else { 00049 p_touch_reset = NULL; 00050 } 00051 } 00052 00053 ~TouchKey() { 00054 if (p_touch_reset != NULL) { 00055 delete p_touch_reset; 00056 } 00057 } 00058 00059 /** Initialization of touch panel IC 00060 * 00061 */ 00062 void Reset(void) { 00063 if (p_touch_reset != NULL) { 00064 *p_touch_reset = 0; 00065 wait_ms(1); 00066 *p_touch_reset = 1; 00067 } 00068 } 00069 00070 /** Attach a function to call when touch panel int 00071 * 00072 * @param fptr A pointer to a void function, or 0 to set as none 00073 */ 00074 void SetCallback(void (*fptr)(void)) { 00075 touch_int.fall(fptr); 00076 } 00077 00078 /** Attach a member function to call when touch panel int 00079 * 00080 * @param tptr pointer to the object to call the member function on 00081 * @param mptr pointer to the member function to be called 00082 */ 00083 template<typename T> 00084 void SetCallback(T* tptr, void (T::*mptr)(void)) { 00085 touch_int.fall(tptr, mptr); 00086 } 00087 00088 /** Get the maximum number of simultaneous touches 00089 * 00090 * @return The maximum number of simultaneous touches. 00091 */ 00092 virtual int GetMaxTouchNum(void) = 0; 00093 00094 /** Get the coordinates 00095 * 00096 * @param touch_buff_num The number of structure p_touch. 00097 * @param p_touch Touch position information. 00098 * @return The number of touch points. 00099 */ 00100 virtual int GetCoordinates(int touch_buff_num, touch_pos_t * p_touch) = 0; 00101 00102 private: 00103 DigitalOut * p_touch_reset; 00104 InterruptIn touch_int; 00105 }; 00106 00107 #endif
Generated on Wed Jul 13 2022 05:33:37 by
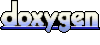