
test public
Dependencies: HttpServer_snapshot_mbed-os
SPDIF_RBSP.cpp
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer* 00021 * Copyright (C) 2018 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 00024 #include "mbed.h" 00025 #include "SPDIF_RBSP.h" 00026 #include "pinmap.h" 00027 00028 #if (R_BSP_SPDIF_ENABLE == 1) 00029 SPDIF_RBSP::SPDIF_RBSP(PinName audio_clk, PinName tx, PinName rx, bool tx_udata_enable, 00030 uint8_t int_level, int32_t max_write_num, int32_t max_read_num) 00031 : mSpdif_(audio_clk, tx, rx) { 00032 00033 spdif_cfg.enabled = true; 00034 spdif_cfg.int_level = int_level; 00035 if ((int32_t)audio_clk == NC) { 00036 spdif_cfg.clk_select = SPDIF_CFG_CKS_AUDIO_X1; 00037 } else { 00038 spdif_cfg.clk_select = SPDIF_CFG_CKS_AUDIO_CLK; 00039 } 00040 spdif_cfg.audio_bit_tx = SPDIF_CFG_AUDIO_BIT_24; 00041 spdif_cfg.audio_bit_rx = SPDIF_CFG_AUDIO_BIT_24; 00042 spdif_cfg.tx_u_data_enabled = tx_udata_enable; 00043 mSpdif_.init(&spdif_cfg, max_write_num, max_read_num); 00044 00045 frequency(44100); // Default sample frequency is 44.1kHz 00046 } 00047 00048 // Public Functions 00049 bool SPDIF_RBSP::outputVolume(float leftVolumeOut, float rightVolumeOut) { 00050 // do nothing 00051 return false; 00052 } 00053 00054 bool SPDIF_RBSP::micVolume(float VolumeIn) { 00055 // do nothing 00056 return false; 00057 } 00058 00059 void SPDIF_RBSP::power(bool type) { 00060 // do nothing 00061 } 00062 00063 bool SPDIF_RBSP::format(char length) { 00064 return mSpdif_.SetTransAudioBit(0, length); 00065 } 00066 00067 bool SPDIF_RBSP::frequency(int hz) { 00068 uint32_t channel_status_Lch; 00069 uint32_t channel_status_Rch; 00070 00071 /* Status Register 00072 b31:b30 Reserved - Read only bit - 00: 00073 b29:b28 CLAC - Clock Accuracy - 01: Level 1 (50 ppm) 00074 b27:b24 FS - Sample Frequency - 0000: 44.1kHz 00075 b23:b20 CHNO - Channel Number - 0001: Left (0010: Right) 00076 b19:b16 SRCNO - Source Number - 0000: 00077 b15:b8 CATCD - Category Code - 00000000: General 2-channel format 00078 b7:b6 Reserved - Read only bit - 00: 00079 b5:b1 CTL - Control - 0: 2 channel Audio, 00080 00: Disable preemphasis 00081 0: Disable copy 00082 0: LPCM 00083 b0 Reserved - Read only bit - 0: */ 00084 00085 switch (hz) { 00086 case 48000: 00087 channel_status_Lch = 0x12100000uL; 00088 channel_status_Rch = 0x12200000uL; 00089 break; 00090 case 44100: 00091 channel_status_Lch = 0x10100000uL; 00092 channel_status_Rch = 0x10200000uL; 00093 break; 00094 case 32000: 00095 channel_status_Lch = 0x13100000uL; 00096 channel_status_Rch = 0x13200000uL; 00097 break; 00098 default: 00099 return false; 00100 } 00101 00102 return mSpdif_.SetChannelStatus(channel_status_Lch, channel_status_Rch); 00103 } 00104 00105 int SPDIF_RBSP::write(void * const p_data, uint32_t data_size, const rbsp_data_conf_t * const p_data_conf) { 00106 return mSpdif_.write(p_data, data_size, p_data_conf); 00107 } 00108 00109 int SPDIF_RBSP::write_s(spdif_t * const p_spdif_data, const rbsp_data_conf_t * const p_data_conf) { 00110 return mSpdif_.write((void *)p_spdif_data, 0, p_data_conf); 00111 } 00112 00113 int SPDIF_RBSP::read(void * const p_data, uint32_t data_size, const rbsp_data_conf_t * const p_data_conf) { 00114 return mSpdif_.read(p_data, data_size, p_data_conf); 00115 } 00116 00117 int SPDIF_RBSP::read_s(spdif_t * const p_spdif_data, const rbsp_data_conf_t * const p_data_conf) { 00118 return mSpdif_.read((void *)p_spdif_data, 0, p_data_conf); 00119 } 00120 00121 #endif /* R_BSP_SPDIF_ENABLE */
Generated on Wed Jul 13 2022 05:33:37 by
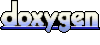