
test public
Dependencies: HttpServer_snapshot_mbed-os
R_BSP_Spdif.h
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer* 00021 * Copyright (C) 2018 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 /**************************************************************************//** 00024 * @file R_BSP_Spdif.h 00025 * @brief SPDIF API 00026 ******************************************************************************/ 00027 00028 00029 #ifndef R_BSP_SPDIF_H 00030 #define R_BSP_SPDIF_H 00031 00032 #if defined(TARGET_RZ_A2XX) 00033 #define R_BSP_SPDIF_ENABLE 1 00034 00035 #include <stdint.h> 00036 #include "R_BSP_SerialFamily.h" 00037 #include "R_BSP_SpdifDef.h" 00038 #include "rtos.h" 00039 #include "pinmap.h" 00040 00041 00042 /** A class to communicate a R_BSP_Spdif 00043 * 00044 */ 00045 class R_BSP_Spdif : public R_BSP_SerialFamily { 00046 00047 public: 00048 00049 /** Constructor 00050 * 00051 * @param audio_clk audio clock 00052 * @param tx SPDIF serial data output 00053 * @param rx SPDIF serial data input 00054 */ 00055 R_BSP_Spdif(PinName audio_clk, PinName tx, PinName rx); 00056 00057 /** Destructor 00058 * 00059 */ 00060 virtual ~R_BSP_Spdif(); 00061 00062 /** Initialization 00063 * 00064 * @param p_ch_cfg SPDIF channel configuration parameter 00065 * @param max_write_num The upper limit of write buffer (SPDIF) 00066 * @param max_read_num The upper limit of read buffer (SPDIF) 00067 * @return true = success, false = failure 00068 */ 00069 void init(const spdif_channel_cfg_t* const p_ch_cfg, int32_t max_write_num, int32_t max_read_num); 00070 00071 /** Get a value of SPDIF channel number 00072 * 00073 * @return SPDIF channel number 00074 */ 00075 int32_t GetSsifChNo(void) { 00076 return spdif_ch; 00077 }; 00078 00079 /** Save configuration to the SPDIF driver 00080 * 00081 * @param p_ch_cfg SPDIF channel configuration parameter 00082 * @return true = success, false = failure 00083 */ 00084 bool ConfigChannel(const spdif_channel_cfg_t* const p_ch_cfg); 00085 00086 bool SetChannelStatus(uint32_t status_Lch, uint32_t status_Rch); 00087 bool GetChannelStatus(uint32_t * p_status_Lch, uint32_t * p_status_Rch); 00088 bool SetTransAudioBit(int direction, char length); 00089 00090 private: 00091 int32_t spdif_ch; 00092 }; 00093 #endif /* TARGET_RZ_A2XX */ 00094 00095 #endif 00096
Generated on Wed Jul 13 2022 05:33:36 by
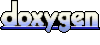