
test public
Dependencies: HttpServer_snapshot_mbed-os
R_BSP_SpdifDef.h
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer 00021 * Copyright (C) 2018 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 /**************************************************************************//** 00024 * @file R_BSP_SpdifDef.h 00025 * @brief SPDIF defines 00026 ******************************************************************************/ 00027 00028 #ifndef R_BSP_SPDIF_DEF_H 00029 #define R_BSP_SPDIF_DEF_H 00030 00031 /****************************************************************************** 00032 Includes <System Includes> , "Project Includes" 00033 ******************************************************************************/ 00034 00035 #ifdef __cplusplus 00036 extern "C" 00037 { 00038 #endif /* __cplusplus */ 00039 00040 /****************************************************************************** 00041 Defines 00042 *****************************************************************************/ 00043 00044 /****************************************************************************** 00045 Constant Macros 00046 *****************************************************************************/ 00047 #define SPDIF_NUM_CHANS (1u) /**< Number of SPDIF channels */ 00048 00049 /* ==== SPDIF Buffer ==== */ 00050 #define SPDIF_NUMOF_FRM (192) 00051 #define SPDIF_NUMOF_CH (2) 00052 #define SPDIF_AUDIO_BUFFSIZE (SPDIF_NUMOF_FRM * SPDIF_NUMOF_CH) 00053 #define SPDIF_USER_BUFFSIZE ((SPDIF_NUMOF_FRM * SPDIF_NUMOF_CH) / 32) 00054 00055 /****************************************************************************** 00056 Function Macros 00057 *****************************************************************************/ 00058 00059 /****************************************************************************** 00060 Enumerated Types 00061 *****************************************************************************/ 00062 /** CTRL:CKS(Clock source for oversampling) */ 00063 typedef enum 00064 { 00065 SPDIF_CFG_CKS_AUDIO_X1 = 0, /**< select AUDIO_X1 */ 00066 SPDIF_CFG_CKS_AUDIO_CLK = 1 /**< select AUIDIO_CLK */ 00067 } spdif_chcfg_cks_t; 00068 00069 /** CTRL:RASS,TASS(Audio bit length) */ 00070 typedef enum 00071 { 00072 SPDIF_CFG_AUDIO_BIT_16 = 0, /**< Audio bit length 16 */ 00073 SPDIF_CFG_AUDIO_BIT_20 = 1, /**< Audio bit length 20 */ 00074 SPDIF_CFG_AUDIO_BIT_24 = 2 /**< Audio bit length 24 */ 00075 } spdif_chcfg_audio_bit_t; 00076 00077 /****************************************************************************** 00078 Structures 00079 *****************************************************************************/ 00080 00081 /** This structure contains the configuration settings */ 00082 typedef struct 00083 { 00084 bool enabled; /**< The enable flag for the channel */ 00085 uint8_t int_level; /**< Interrupt priority for the channel */ 00086 spdif_chcfg_cks_t clk_select; /**< CTRL-CKS : Audio clock select */ 00087 spdif_chcfg_audio_bit_t audio_bit_tx; /**< CTRL-TASS : Audio bit length */ 00088 spdif_chcfg_audio_bit_t audio_bit_rx; /**< CTRL-RASS : Audio bit length */ 00089 bool tx_u_data_enabled; /**< CTRL-RASS : Audio bit length */ 00090 } spdif_channel_cfg_t; 00091 00092 typedef struct 00093 { 00094 uint32_t Lch; /**< Channel Status bit Lch */ 00095 uint32_t Rch; /**< Channel Status bit Rch */ 00096 } spdif_channel_status_t; 00097 00098 typedef struct 00099 { 00100 uint32_t a_buff[SPDIF_AUDIO_BUFFSIZE]; /**< Audio Buffer */ 00101 uint32_t u_buff[SPDIF_USER_BUFFSIZE]; /**< User Buffer */ 00102 uint32_t s_buff[SPDIF_NUMOF_CH]; /**< Channel Status Buffer */ 00103 } spdif_t; 00104 00105 /****************************************************************************** 00106 IOCTLS 00107 *****************************************************************************/ 00108 00109 #define SPDIF_CONFIG_CHANNEL (0) 00110 #define SPDIF_SET_CHANNEL_STATUS (1) 00111 #define SPDIF_GET_CHANNEL_STATUS (2) 00112 #define SPDIF_SET_TRANS_AUDIO_BIT (3) 00113 #define SPDIF_SET_RECV_AUDIO_BIT (4) 00114 00115 #ifdef __cplusplus 00116 } 00117 #endif /* __cplusplus */ 00118 00119 #endif /* R_BSP_SPDIF_DEF_H */
Generated on Wed Jul 13 2022 05:33:36 by
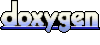