
test public
Dependencies: HttpServer_snapshot_mbed-os
PwmOutSpeaker.h
00001 /* mbed PwmOutSpeaker Library 00002 * Copyright (C) 2016 dkato 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef PWMOUT_SPEAKER_H 00018 #define PWMOUT_SPEAKER_H 00019 00020 #include "mbed.h" 00021 #include "AUDIO_RBSP.h" 00022 00023 /** PwmOutSpeaker class 00024 * 00025 */ 00026 class PwmOutSpeaker : public AUDIO_RBSP { 00027 public: 00028 /** Create a PwmOutSpeaker 00029 * 00030 * @param pwm_l 00031 * @param pwm_r 00032 */ 00033 PwmOutSpeaker(PinName pwm_l, PinName pwm_r); 00034 00035 virtual ~PwmOutSpeaker(); 00036 00037 virtual void power(bool type = true) { 00038 return; 00039 } 00040 00041 /** Set I2S interface bit length and mode 00042 * 00043 * @param length Set bit length to 8 or 16 bits 00044 * @return true = success, false = failure 00045 */ 00046 virtual bool format(char length); 00047 00048 /** Set sample frequency 00049 * 00050 * @param frequency Sample frequency of data in Hz 00051 * @return true = success, false = failure 00052 * 00053 * Supports the following frequencies: 8kHz, 8.021kHz, 32kHz, 44.1kHz, 48kHz 00054 * Default is 44.1kHz 00055 */ 00056 virtual bool frequency(int hz); 00057 00058 /** Enqueue asynchronous write request 00059 * 00060 * @param p_data Location of the data 00061 * @param data_size Number of bytes to write 00062 * @return Number of bytes written on success. negative number on error. 00063 */ 00064 virtual int write(void * const p_data, uint32_t data_size, const rbsp_data_conf_t * const p_data_conf = NULL); 00065 00066 virtual int read(void * const p_data, uint32_t data_size, const rbsp_data_conf_t * const p_data_conf = NULL) { 00067 return -1; 00068 } 00069 00070 /** Volume control 00071 * 00072 * @param volume Speaker volume 00073 * @return Returns "true" for success, "false" if parameters are out of range 00074 * Parameters accept a value, where 0.0 <= parameter <= 1.0 (1.0 = default) 00075 */ 00076 virtual bool outputVolume(float leftVolumeOut, float rightVolumeOut); 00077 00078 virtual bool micVolume(float VolumeIn) { 00079 return false; 00080 } 00081 00082 private: 00083 #define WRITE_BUFF_SIZE (1024 * 4) 00084 #define MSK_RING_BUFF (WRITE_BUFF_SIZE - 1) 00085 00086 PwmOut *_speaker_l; 00087 PwmOut *_speaker_r; 00088 Ticker _timer; 00089 int _length; 00090 int _hz_multi; 00091 int _data_cnt; 00092 bool _playing; 00093 volatile uint32_t _bottom; 00094 volatile uint32_t _top; 00095 float _speaker_vol_l; 00096 float _speaker_vol_r; 00097 float _pwm_duty_buf[WRITE_BUFF_SIZE]; 00098 Thread _audioThread; 00099 Semaphore _sound_out_req; 00100 00101 void sound_out(void); 00102 void audio_process(); 00103 }; 00104 00105 #endif // PWMOUT_SPEAKER_H
Generated on Wed Jul 13 2022 05:33:36 by
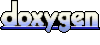