
test public
Dependencies: HttpServer_snapshot_mbed-os
MAX9867_RBSP.cpp
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer* 00021 * Copyright (C) 2017 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 00024 #include "mbed.h" 00025 #include "MAX9867_RBSP.h" 00026 #include "pinmap.h" 00027 00028 MAX9867_RBSP::MAX9867_RBSP(PinName sda, PinName scl, PinName sck, PinName ws, PinName tx, PinName rx, 00029 uint8_t int_level, int32_t max_write_num, int32_t max_read_num) 00030 : mI2c_(sda, scl), mI2s_(sck, ws, tx, rx) { 00031 mAddr = 0x30; 00032 00033 // I2S Mode 00034 ssif_cfg.enabled = true; 00035 ssif_cfg.int_level = int_level; 00036 ssif_cfg.slave_mode = true; 00037 ssif_cfg.sample_freq = 44100u; 00038 ssif_cfg.clk_select = SSIF_CFG_CKS_AUDIO_X1; 00039 ssif_cfg.multi_ch = SSIF_CFG_MULTI_CH_1; 00040 ssif_cfg.data_word = SSIF_CFG_DATA_WORD_16; 00041 ssif_cfg.system_word = SSIF_CFG_SYSTEM_WORD_32; 00042 ssif_cfg.bclk_pol = SSIF_CFG_FALLING; 00043 ssif_cfg.ws_pol = SSIF_CFG_WS_LOW; 00044 ssif_cfg.padding_pol = SSIF_CFG_PADDING_LOW; 00045 ssif_cfg.serial_alignment = SSIF_CFG_DATA_FIRST; 00046 ssif_cfg.parallel_alignment = SSIF_CFG_LEFT; 00047 ssif_cfg.ws_delay = SSIF_CFG_DELAY; 00048 ssif_cfg.noise_cancel = SSIF_CFG_ENABLE_NOISE_CANCEL; 00049 ssif_cfg.tdm_mode = SSIF_CFG_DISABLE_TDM; 00050 ssif_cfg.romdec_direct.mode = SSIF_CFG_DISABLE_ROMDEC_DIRECT; 00051 ssif_cfg.romdec_direct.p_cbfunc = NULL; 00052 mI2s_.init(&ssif_cfg, max_write_num, max_read_num); 00053 00054 mI2c_.frequency(150000); 00055 power(false); // Power off 00056 frequency(44100); // Default sample frequency is 44.1kHz 00057 activateDigitalInterface_(); // The digital part of the chip is active 00058 } 00059 00060 // Public Functions 00061 bool MAX9867_RBSP::outputVolume(float leftVolumeOut, float rightVolumeOut) { 00062 // check values are in range 00063 if ((leftVolumeOut < 0.0) || (leftVolumeOut > 1.0) 00064 || (rightVolumeOut < 0.0) || (rightVolumeOut > 1.0)) { 00065 return false; 00066 } 00067 00068 // convert float to encoded char 00069 char left = (char)(40 - (40 * leftVolumeOut)); 00070 char right = (char)(40 - (40 * rightVolumeOut)); 00071 00072 cmd[0] = 0x10; // Playback Volume Registers 00073 cmd[1] = left; 00074 cmd[2] = right; 00075 mI2c_.write(mAddr, cmd, 3); 00076 00077 return true; 00078 } 00079 00080 bool MAX9867_RBSP::micVolume(float VolumeIn) { 00081 // check values are in range 00082 if ((VolumeIn < 0.0) || (VolumeIn > 1.0)) { 00083 return false; 00084 } 00085 00086 // convert float to encoded char 00087 char vol = (char)(50 * VolumeIn); 00088 00089 cmd[0] = 0x12; // Microphone Input Registers 00090 if (vol == 0) { 00091 cmd[1] = 0x00; // PAREN=00,PGAMR=0x00 00092 } else if (vol <= 20) { 00093 cmd[1] = 0x20 + 20 - vol; // PAREN=01,PGAMR=20-vol 00094 } else if (vol <= 40) { 00095 cmd[1] = 0x40 + 40 - vol; // PAREN=10,PGAMR=40-vol 00096 } else { 00097 cmd[1] = 0x60 + 50 - vol; // PAREN=11,PGAMR=50-vol 00098 } 00099 cmd[2] = 0x00; // Right Microphone Gain PAREN=00,PGAMR=0x00 00100 mI2c_.write(mAddr, cmd, 3); 00101 00102 return true; 00103 } 00104 00105 void MAX9867_RBSP::power(bool type) { 00106 cmd[0] = 0x17; // Power-Management Register 00107 if (type) { 00108 cmd[1] = 0x8E; // SHDN=1,LNLEN=0,LNREN=0,DALEN=1,DAREN=1,ADLEN=1,ADREN=0 00109 } else { 00110 cmd[1] = 0x80; // SHDN=1,LNLEN=0,LNREN=0,DALEN=0,DAREN=0,ADLEN=0,ADREN=0 00111 } 00112 mI2c_.write(mAddr, cmd, 2); 00113 } 00114 00115 bool MAX9867_RBSP::format(char length) { 00116 if (length != 16) { 00117 return false; 00118 } 00119 return true; 00120 } 00121 00122 bool MAX9867_RBSP::frequency(int hz) { 00123 uint16_t lrclk; 00124 00125 switch (hz) { 00126 case 48000: 00127 lrclk = 0x624E; 00128 break; 00129 case 44100: 00130 lrclk = 0x5A51; 00131 break; 00132 case 32000: 00133 lrclk = 0x4189; 00134 break; 00135 case 24000: 00136 lrclk = 0x3127; 00137 break; 00138 case 16000: 00139 lrclk = 0x20C5; 00140 break; 00141 case 8000: 00142 lrclk = 0x1062; 00143 break; 00144 default: 00145 return false; 00146 } 00147 00148 cmd[0] = 0x05; // Clock Control Registers 00149 cmd[1] = 0x10; // PSCLK = 00 FREQ = 0x0 00150 cmd[2] = (uint8_t)(lrclk >> 8); 00151 cmd[3] = (uint8_t)(lrclk); 00152 mI2c_.write(mAddr, cmd, 4); 00153 00154 return true; 00155 } 00156 00157 // Private Functions 00158 void MAX9867_RBSP::activateDigitalInterface_(void) { 00159 cmd[0] = 0x04; // Interrupt Enable 00160 cmd[1] = 0x00; // ICLE=0,ISLD=0,IULK=0,SDODLY=0,IJDET=0 00161 mI2c_.write(mAddr, cmd, 2); 00162 00163 cmd[0] = 0x08; // Interface Mode 00164 cmd[1] = 0x90; // MAS=1,WCI=0,BCI=0,DLY=1,HIZOFF=0,TDM=0 00165 cmd[2] = 0x02; // LVOLFIX=0,DMONO=0,BSEL=010 00166 mI2c_.write(mAddr, cmd, 3); 00167 00168 cmd[0] = 0x0B; // Digital Gain Control 00169 cmd[1] = 0x00; // DSTS=0,DVST=0 00170 mI2c_.write(mAddr, cmd, 2); 00171 00172 cmd[0] = 0x0E; // Line Input Registers 00173 cmd[1] = 0x40; // LILM=1,LIGL=0x00 00174 cmd[2] = 0x40; // LIRM=1,LIGR=0x00 00175 mI2c_.write(mAddr, cmd, 3); 00176 00177 cmd[0] = 0x12; // Microphone Input Registers 00178 cmd[1] = 0x00; // Left Microphone Gain PAREN=00,PGAMR=0x00 00179 cmd[2] = 0x00; // Right Microphone Gain PAREN=00,PGAMR=0x00 00180 mI2c_.write(mAddr, cmd, 3); 00181 00182 cmd[0] = 0x14; // CONFIGURATION 00183 cmd[1] = 0x40; // MXINL=01,MXINR=00,AUXCAP=0,AUXGAIN=0,AUXCAL=0,AUXEN=0 00184 cmd[2] = 0x00; // MICCLK=0,DIGMICL=0,DIGMICR=0 00185 cmd[3] = 0x02; // DSLEW=0,VSEN=0,ZDEN=0,JDETEN=0,HPMODE=000 00186 mI2c_.write(mAddr, cmd, 4); 00187 }
Generated on Wed Jul 13 2022 05:33:36 by
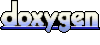