
test public
Dependencies: HttpServer_snapshot_mbed-os
EasyPlayback.h
00001 /* mbed EasyPlayback Library 00002 * Copyright (C) 2017 dkato 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef __EASY_PLAYBACK_H__ 00018 #define __EASY_PLAYBACK_H__ 00019 00020 #include <string> 00021 #include <map> 00022 #include "EasyDecoder.h" 00023 #include "AUDIO_GRBoard.h" 00024 #include "PwmOutSpeaker.h" 00025 #include "SPDIF_GRBoard.h" 00026 #include "SoundlessSpeaker.h" 00027 #include "NullSpeaker.h" 00028 #include "FATFileSystem.h" 00029 00030 class EasyPlayback 00031 { 00032 public: 00033 typedef enum { 00034 AUDIO_TPYE_SSIF, 00035 AUDIO_TPYE_PWM, 00036 AUDIO_TPYE_SPDIF, 00037 AUDIO_TPYE_SOUNDLESS, 00038 AUDIO_TPYE_NULL 00039 } audio_type_t; 00040 00041 EasyPlayback(audio_type_t type = AUDIO_TPYE_SSIF, PinName pin1 = NC, PinName pin2 = NC); 00042 ~EasyPlayback(); 00043 bool get_tag(const char* filename, char* p_title, char* p_artist, char* p_album, uint16_t tag_size); 00044 bool play(const char* filename); 00045 bool is_paused(void); 00046 void pause(void); 00047 void pause(bool type); 00048 void skip(void); 00049 bool outputVolume(float VolumeOut); 00050 00051 template<typename T> 00052 void add_decoder(const string& extension) { 00053 m_lpDecoders[extension] = &T::inst; 00054 } 00055 00056 private: 00057 AUDIO_GRBoard * _audio_ssif; 00058 PwmOutSpeaker * _audio_pwm; 00059 #if (R_BSP_SPDIF_ENABLE == 1) 00060 SPDIF_GRBoard * _audio_spdif; 00061 #endif 00062 SoundlessSpeaker * _audio_soundless; 00063 NullSpeaker * _audio_null; 00064 AUDIO_RBSP * _audio; 00065 int _buff_index; 00066 audio_type_t _type; 00067 bool _skip; 00068 bool _pause; 00069 bool _init_end; 00070 uint32_t _audio_write_buff_num; 00071 uint32_t _audio_buff_size; 00072 uint8_t *_heap_buf; 00073 uint8_t *_audio_buf; 00074 std::map<std::string, EasyDecoder*(*)()> m_lpDecoders; 00075 00076 EasyDecoder * create_decoer_class(const char* filename); 00077 }; 00078 00079 #endif
Generated on Wed Jul 13 2022 05:33:36 by
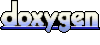