
test public
Dependencies: HttpServer_snapshot_mbed-os
EasyDecoder.h
00001 /* mbed EasyDecoder Library 00002 * Copyright (C) 2017 dkato 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef __EASY_DECODER_H__ 00018 #define __EASY_DECODER_H__ 00019 00020 #include "mbed.h" 00021 00022 class EasyDecoder { 00023 public: 00024 00025 virtual ~EasyDecoder(){} 00026 00027 /** analyze header 00028 * 00029 * @param p_title title tag buffer 00030 * @param p_artist artist tag buffer 00031 * @param p_album album tag buffer 00032 * @param tag_size tag buffer size 00033 * @param fp file pointer 00034 * @return true = success, false = failure 00035 */ 00036 virtual bool AnalyzeHeder(char* p_title, char* p_artist, char* p_album, uint16_t tag_size, FILE* fp) = 0; 00037 00038 /** get next data 00039 * 00040 * @param buf data buffer address 00041 * @param len data buffer length 00042 * @return get data size 00043 */ 00044 virtual size_t GetNextData(void *buf, size_t len) = 0; 00045 00046 /** get channel 00047 * 00048 * @return channel 00049 */ 00050 virtual uint16_t GetChannel() = 0; 00051 00052 /** get block size 00053 * 00054 * @return block size 00055 */ 00056 virtual uint16_t GetBlockSize() = 0; 00057 00058 /** get sampling rate 00059 * 00060 * @return sampling rate 00061 */ 00062 virtual uint32_t GetSamplingRate() = 0; 00063 00064 }; 00065 00066 #endif
Generated on Wed Jul 13 2022 05:33:36 by
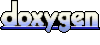