
test public
Dependencies: HttpServer_snapshot_mbed-os
EasyDec_Mov.h
00001 /* mbed EasyDec_Mov Library 00002 * Copyright (C) 2018 dkato 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 /**************************************************************************//** 00018 * @file EasyDec_Mov.h 00019 * @brief mov 00020 ******************************************************************************/ 00021 #ifndef __EASY_DEC_MOV_H__ 00022 #define __EASY_DEC_MOV_H__ 00023 00024 #include "EasyDecoder.h" 00025 00026 /** A class to communicate a EasyDec_Mov 00027 * 00028 */ 00029 class EasyDec_Mov : public EasyDecoder { 00030 public: 00031 00032 static inline EasyDecoder* inst() { return new EasyDec_Mov; } 00033 00034 /** Attach a function to be called when acquiring an image 00035 * 00036 * @param func pointer to the function to be called 00037 * @param video_buf video buffer address 00038 * @param video_buf_size video buffer size 00039 */ 00040 static void attach(Callback<void()> func, uint8_t * video_buf, uint32_t video_buf_size); 00041 00042 /** analyze header 00043 * 00044 * @param p_title title tag buffer 00045 * @param p_artist artist tag buffer 00046 * @param p_album album tag buffer 00047 * @param tag_size tag buffer size 00048 * @param fp file pointer 00049 * @return true = success, false = failure 00050 */ 00051 virtual bool AnalyzeHeder(char* p_title, char* p_artist, char* p_album, uint16_t tag_size, FILE* fp); 00052 00053 /** get next data 00054 * 00055 * @param buf data buffer address 00056 * @param len data buffer length 00057 * @return get data size 00058 */ 00059 virtual size_t GetNextData(void *buf, size_t len); 00060 00061 /** get channel 00062 * 00063 * @return channel 00064 */ 00065 virtual uint16_t GetChannel(); 00066 00067 /** get block size 00068 * 00069 * @return block size 00070 */ 00071 virtual uint16_t GetBlockSize(); 00072 00073 /** get sampling rate 00074 * 00075 * @return sampling rate 00076 */ 00077 virtual uint32_t GetSamplingRate(); 00078 00079 private: 00080 union __attribute__((packed)) Buffer { 00081 uint8_t array[4]; 00082 uint32_t value; 00083 }; 00084 static uint8_t * _videoBuf; 00085 static uint32_t _videoBufSize; 00086 static Callback<void()> _function; /**< Callback. */ 00087 00088 static const int bufSize = 32; 00089 uint32_t frameSizes[bufSize]; 00090 uint32_t audioSizes[bufSize]; 00091 FILE * mov_fp; 00092 uint32_t numOfFrames; 00093 uint32_t *frameSizesP; 00094 uint32_t *audioSizesP; 00095 uint32_t stszAddress; 00096 uint32_t stcoAddress; 00097 uint32_t lastFrameAddress; 00098 int availableCount; 00099 bool _video_flg; 00100 00101 void search(uint32_t pattern); 00102 void fillCaches(); 00103 }; 00104 00105 #endif 00106
Generated on Wed Jul 13 2022 05:33:36 by
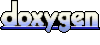