
test public
Dependencies: HttpServer_snapshot_mbed-os
ESP32Interface.cpp
00001 /* ESP32 implementation of NetworkInterfaceAPI 00002 * Copyright (c) 2017 Renesas Electronics Corporation 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include <string.h> 00018 #include "ESP32Interface.h" 00019 00020 // ESP32Interface implementation 00021 ESP32Interface::ESP32Interface() : 00022 ESP32Stack(MBED_CONF_ESP32_WIFI_EN, MBED_CONF_ESP32_WIFI_IO0, MBED_CONF_ESP32_WIFI_TX, MBED_CONF_ESP32_WIFI_RX, MBED_CONF_ESP32_WIFI_DEBUG, 00023 MBED_CONF_ESP32_WIFI_RTS, MBED_CONF_ESP32_WIFI_CTS, MBED_CONF_ESP32_WIFI_BAUDRATE, 0), 00024 _dhcp(true), 00025 _ap_ssid(), 00026 _ap_pass(), 00027 _ap_sec(NSAPI_SECURITY_NONE), 00028 _ip_address(), 00029 _netmask(), 00030 _gateway(), 00031 _connection_status(NSAPI_STATUS_DISCONNECTED), 00032 _connection_status_cb(NULL) 00033 { 00034 memset(_ap_ssid, 0, sizeof(_ap_ssid)); 00035 _esp->attach_wifi_status(callback(this, &ESP32Interface::wifi_status_cb)); 00036 } 00037 00038 ESP32Interface::ESP32Interface(PinName en, PinName io0, PinName tx, PinName rx, bool debug, 00039 PinName rts, PinName cts, int baudrate) : 00040 ESP32Stack(en, io0, tx, rx, debug, rts, cts, baudrate, 0), 00041 _dhcp(true), 00042 _ap_ssid(), 00043 _ap_pass(), 00044 _ap_sec(NSAPI_SECURITY_NONE), 00045 _ip_address(), 00046 _netmask(), 00047 _gateway(), 00048 _connection_status(NSAPI_STATUS_DISCONNECTED), 00049 _connection_status_cb(NULL) 00050 { 00051 memset(_ap_ssid, 0, sizeof(_ap_ssid)); 00052 _esp->attach_wifi_status(callback(this, &ESP32Interface::wifi_status_cb)); 00053 } 00054 00055 ESP32Interface::ESP32Interface(PinName tx, PinName rx, bool debug) : 00056 ESP32Stack(NC, NC, tx, rx, debug, NC, NC, 230400, 0), 00057 _dhcp(true), 00058 _ap_ssid(), 00059 _ap_pass(), 00060 _ap_sec(NSAPI_SECURITY_NONE), 00061 _ip_address(), 00062 _netmask(), 00063 _gateway(), 00064 _connection_status(NSAPI_STATUS_DISCONNECTED), 00065 _connection_status_cb(NULL) 00066 { 00067 memset(_ap_ssid, 0, sizeof(_ap_ssid)); 00068 _esp->attach_wifi_status(callback(this, &ESP32Interface::wifi_status_cb)); 00069 } 00070 00071 nsapi_error_t ESP32Interface::set_network(const char *ip_address, const char *netmask, const char *gateway) 00072 { 00073 _dhcp = false; 00074 00075 strncpy(_ip_address, ip_address ? ip_address : "", sizeof(_ip_address)); 00076 _ip_address[sizeof(_ip_address) - 1] = '\0'; 00077 strncpy(_netmask, netmask ? netmask : "", sizeof(_netmask)); 00078 _netmask[sizeof(_netmask) - 1] = '\0'; 00079 strncpy(_gateway, gateway ? gateway : "", sizeof(_gateway)); 00080 _gateway[sizeof(_gateway) - 1] = '\0'; 00081 00082 return NSAPI_ERROR_OK; 00083 } 00084 00085 nsapi_error_t ESP32Interface::set_dhcp(bool dhcp) 00086 { 00087 _dhcp = dhcp; 00088 00089 return NSAPI_ERROR_OK; 00090 } 00091 00092 int ESP32Interface::connect(const char *ssid, const char *pass, nsapi_security_t security, 00093 uint8_t channel) 00094 { 00095 if (channel != 0) { 00096 return NSAPI_ERROR_UNSUPPORTED; 00097 } 00098 00099 int ret = set_credentials(ssid, pass, security); 00100 if (ret != NSAPI_ERROR_OK) { 00101 return ret; 00102 } 00103 return connect(); 00104 } 00105 00106 int ESP32Interface::connect() 00107 { 00108 if (_ap_ssid[0] == 0) { 00109 return NSAPI_ERROR_NO_SSID; 00110 } 00111 00112 if (!_esp->dhcp(_dhcp, 1)) { 00113 return NSAPI_ERROR_DHCP_FAILURE; 00114 } 00115 00116 if (!_dhcp) { 00117 if (!_esp->set_network(_ip_address, _netmask, _gateway)) { 00118 return NSAPI_ERROR_DEVICE_ERROR; 00119 } 00120 } 00121 00122 set_connection_status(NSAPI_STATUS_CONNECTING); 00123 if (!_esp->connect(_ap_ssid, _ap_pass)) { 00124 set_connection_status(NSAPI_STATUS_DISCONNECTED); 00125 return NSAPI_ERROR_NO_CONNECTION; 00126 } 00127 00128 return NSAPI_ERROR_OK; 00129 } 00130 00131 int ESP32Interface::set_credentials(const char *ssid, const char *pass, nsapi_security_t security) 00132 { 00133 int ret = NSAPI_ERROR_OK; 00134 size_t pass_len; 00135 00136 if ((ssid == NULL) || (ssid[0] == 0)) { 00137 return NSAPI_ERROR_PARAMETER; 00138 } 00139 00140 if ((pass == NULL) || (pass[0] == 0)) { 00141 pass_len = 0; 00142 } else { 00143 pass_len = strlen(pass); 00144 } 00145 00146 switch (security) { 00147 case NSAPI_SECURITY_NONE: 00148 if (pass_len != 0) { 00149 ret = NSAPI_ERROR_PARAMETER; 00150 } 00151 break; 00152 case NSAPI_SECURITY_WEP: 00153 if ((pass_len < 5) || (pass_len > 26)) { 00154 ret = NSAPI_ERROR_PARAMETER; 00155 } 00156 break; 00157 case NSAPI_SECURITY_WPA: 00158 case NSAPI_SECURITY_WPA2: 00159 case NSAPI_SECURITY_WPA_WPA2: 00160 if ((pass_len < 8) || (pass_len > 63)) { 00161 ret = NSAPI_ERROR_PARAMETER; 00162 } 00163 break; 00164 case NSAPI_SECURITY_UNKNOWN: 00165 // do nothing 00166 break; 00167 default: 00168 ret = NSAPI_ERROR_UNSUPPORTED; 00169 break; 00170 } 00171 00172 if (ret != NSAPI_ERROR_OK) { 00173 return ret; 00174 } 00175 00176 memset(_ap_ssid, 0, sizeof(_ap_ssid)); 00177 strncpy(_ap_ssid, ssid, sizeof(_ap_ssid)); 00178 00179 memset(_ap_pass, 0, sizeof(_ap_pass)); 00180 if (pass_len != 0) { 00181 strncpy(_ap_pass, pass, pass_len); 00182 } 00183 00184 _ap_sec = security; 00185 00186 return NSAPI_ERROR_OK; 00187 } 00188 00189 int ESP32Interface::set_channel(uint8_t channel) 00190 { 00191 return NSAPI_ERROR_UNSUPPORTED; 00192 } 00193 00194 int ESP32Interface::disconnect() 00195 { 00196 if (_connection_status == NSAPI_STATUS_DISCONNECTED) { 00197 return NSAPI_ERROR_NO_CONNECTION; 00198 } 00199 00200 if (!_esp->disconnect()) { 00201 return NSAPI_ERROR_DEVICE_ERROR; 00202 } 00203 00204 return NSAPI_ERROR_OK; 00205 } 00206 00207 const char *ESP32Interface::get_ip_address() 00208 { 00209 return _esp->getIPAddress(); 00210 } 00211 00212 const char *ESP32Interface::get_mac_address() 00213 { 00214 return _esp->getMACAddress(); 00215 } 00216 00217 const char *ESP32Interface::get_gateway() 00218 { 00219 return _esp->getGateway(); 00220 } 00221 00222 const char *ESP32Interface::get_netmask() 00223 { 00224 return _esp->getNetmask(); 00225 } 00226 00227 int8_t ESP32Interface::get_rssi() 00228 { 00229 return _esp->getRSSI(); 00230 } 00231 00232 int ESP32Interface::scan(WiFiAccessPoint *res, unsigned count) 00233 { 00234 return _esp->scan(res, count); 00235 } 00236 00237 void ESP32Interface::attach(mbed::Callback<void(nsapi_event_t, intptr_t)> status_cb) 00238 { 00239 _connection_status_cb = status_cb; 00240 } 00241 00242 nsapi_connection_status_t ESP32Interface::get_connection_status() const 00243 { 00244 return _connection_status; 00245 } 00246 00247 void ESP32Interface::set_connection_status(nsapi_connection_status_t connection_status) 00248 { 00249 if (_connection_status != connection_status) { 00250 _connection_status = connection_status; 00251 if (_connection_status_cb) { 00252 _connection_status_cb(NSAPI_EVENT_CONNECTION_STATUS_CHANGE, _connection_status); 00253 } 00254 } 00255 } 00256 00257 void ESP32Interface::wifi_status_cb(int8_t wifi_status) 00258 { 00259 switch (wifi_status) { 00260 case ESP32::STATUS_DISCONNECTED: 00261 set_connection_status(NSAPI_STATUS_DISCONNECTED); 00262 break; 00263 case ESP32::STATUS_GOT_IP: 00264 set_connection_status(NSAPI_STATUS_GLOBAL_UP); 00265 break; 00266 case ESP32::STATUS_CONNECTED: 00267 default: 00268 // do nothing 00269 break; 00270 } 00271 } 00272 00273 #if MBED_CONF_ESP32_PROVIDE_DEFAULT 00274 00275 WiFiInterface *WiFiInterface::get_default_instance() { 00276 static ESP32Interface esp32; 00277 return &esp32; 00278 } 00279 00280 #endif 00281
Generated on Wed Jul 13 2022 05:33:36 by
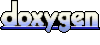