
test public
Dependencies: HttpServer_snapshot_mbed-os
DisplayApp.cpp
00001 /* Copyright (c) 2016 dkato 00002 * SPDX-License-Identifier: Apache-2.0 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #include "mbed.h" 00017 #include "DisplayApp.h" 00018 00019 void DisplayApp::display_app_process() { 00020 int data; 00021 int pos_x_wk; 00022 int pos_wk; 00023 00024 PcApp.connect(); 00025 00026 while (!PcApp.configured()) { 00027 ThisThread::sleep_for(100); 00028 } 00029 00030 while (1) { 00031 if (PcApp.readable()) { 00032 data = PcApp.getc(); 00033 if (data == '{') { 00034 pos_seq = POS_SEQ_START; 00035 } else if (data == 'X') { 00036 if (pos_seq == POS_SEQ_START) { 00037 pos_seq = POS_SEQ_X; 00038 } else { 00039 pos_seq = POS_SEQ_INIT; 00040 } 00041 } else if (data == 'Y') { 00042 if (pos_seq == POS_SEQ_C) { 00043 pos_seq = POS_SEQ_Y; 00044 } else { 00045 pos_seq = POS_SEQ_INIT; 00046 } 00047 } else if (data == '=') { 00048 if (pos_seq == POS_SEQ_X) { 00049 pos_seq = POS_SEQ_X_POS; 00050 pos_wk = 0; 00051 } else if (pos_seq == POS_SEQ_Y) { 00052 pos_seq = POS_SEQ_Y_POS; 00053 pos_wk = 0; 00054 } else { 00055 pos_seq = POS_SEQ_INIT; 00056 } 00057 } else if (data == '-') { 00058 if (pos_seq == POS_SEQ_X_POS) { 00059 pos_seq = POS_SEQ_X_M; 00060 } else if (pos_seq == POS_SEQ_Y_POS) { 00061 pos_seq = POS_SEQ_Y_M; 00062 } else { 00063 pos_seq = POS_SEQ_INIT; 00064 } 00065 } else if ((data >= '0') && (data <= '9')) { 00066 if ((pos_seq == POS_SEQ_X_POS) || (pos_seq == POS_SEQ_Y_POS)) { 00067 pos_wk = (pos_wk * 10) + (data - '0'); 00068 } else if ((pos_seq == POS_SEQ_X_M) && (data == '1')) { 00069 pos_wk = -1; 00070 } else if ((pos_seq == POS_SEQ_Y_M) && (data == '1')) { 00071 pos_wk = -1; 00072 } else { 00073 pos_seq = POS_SEQ_INIT; 00074 } 00075 } else if (data == ',') { 00076 if ((pos_seq == POS_SEQ_X_POS) || (pos_seq == POS_SEQ_X_M)) { 00077 pos_x_wk = pos_wk; 00078 pos_seq = POS_SEQ_C; 00079 } else { 00080 pos_seq = POS_SEQ_INIT; 00081 } 00082 } else if (data == '}') { 00083 if ((pos_seq == POS_SEQ_Y_POS) || (pos_seq == POS_SEQ_Y_M)) { 00084 pos_seq = POS_SEQ_END; 00085 if ((pos_x != pos_x_wk) || (pos_y != pos_wk)) { 00086 pos_x = pos_x_wk; 00087 pos_y = pos_wk; 00088 if (event) { 00089 event.call(); 00090 } 00091 } 00092 } else { 00093 pos_seq = POS_SEQ_INIT; 00094 } 00095 } else { 00096 pos_seq = POS_SEQ_INIT; 00097 } 00098 } else { 00099 ThisThread::sleep_for(10); 00100 } 00101 } 00102 } 00103 00104 DisplayApp::DisplayApp(osPriority tsk_pri, uint32_t stack_size) : 00105 PcApp(false), displayThread(tsk_pri, stack_size) { 00106 displayThread.start(callback(this, &DisplayApp::display_app_process)); 00107 } 00108 00109 void DisplayApp::SendHeader(uint32_t size) { 00110 uint8_t headder_data[12] = {0xFF,0xFF,0xAA,0x55,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00}; 00111 00112 headder_data[8] = (uint8_t)((uint32_t)size >> 0); 00113 headder_data[9] = (uint8_t)((uint32_t)size >> 8); 00114 headder_data[10] = (uint8_t)((uint32_t)size >> 16); 00115 headder_data[11] = (uint8_t)((uint32_t)size >> 24); 00116 PcApp.send((uint8_t *)headder_data, sizeof(headder_data)); 00117 } 00118 00119 void DisplayApp::SendData(uint8_t * buf, uint32_t size) { 00120 PcApp.send(buf, size); 00121 } 00122 00123 int DisplayApp::SendRgb888(uint8_t * buf, uint32_t pic_width, uint32_t pic_height) { 00124 uint32_t offset_size = 54; 00125 uint32_t buf_stride = (((pic_width * 4u) + 31u) & ~31u); 00126 uint32_t pic_size = buf_stride * pic_height; 00127 uint32_t total_size = pic_size + offset_size; 00128 uint8_t wk_bitmap_buf[54]; 00129 int wk_idx = 0; 00130 00131 if (!PcApp.configured()) { 00132 return 0; 00133 } 00134 SendHeader(total_size); 00135 00136 /* BITMAPFILEHEADER */ 00137 wk_bitmap_buf[wk_idx++] = 'B'; 00138 wk_bitmap_buf[wk_idx++] = 'M'; 00139 wk_bitmap_buf[wk_idx++] = (uint8_t)(total_size >> 0); /* bfSize */ 00140 wk_bitmap_buf[wk_idx++] = (uint8_t)(total_size >> 8); /* bfSize */ 00141 wk_bitmap_buf[wk_idx++] = (uint8_t)(total_size >> 16); /* bfSize */ 00142 wk_bitmap_buf[wk_idx++] = (uint8_t)(total_size >> 24); /* bfSize */ 00143 wk_bitmap_buf[wk_idx++] = 0; /* bfReserved1 */ 00144 wk_bitmap_buf[wk_idx++] = 0; /* bfReserved1 */ 00145 wk_bitmap_buf[wk_idx++] = 0; /* bfReserved2 */ 00146 wk_bitmap_buf[wk_idx++] = 0; /* bfReserved2 */ 00147 wk_bitmap_buf[wk_idx++] = (uint8_t)(offset_size >> 0); /* bfOffBits */ 00148 wk_bitmap_buf[wk_idx++] = (uint8_t)(offset_size >> 8); /* bfOffBits */ 00149 wk_bitmap_buf[wk_idx++] = (uint8_t)(offset_size >> 16); /* bfOffBits */ 00150 wk_bitmap_buf[wk_idx++] = (uint8_t)(offset_size >> 24); /* bfOffBits */ 00151 00152 /* BITMAPINFOHEADER */ 00153 wk_bitmap_buf[wk_idx++] = 40; /* biSize */ 00154 wk_bitmap_buf[wk_idx++] = 0; /* biSize */ 00155 wk_bitmap_buf[wk_idx++] = 0; /* biSize */ 00156 wk_bitmap_buf[wk_idx++] = 0; /* biSize */ 00157 wk_bitmap_buf[wk_idx++] = (uint8_t)(pic_width >> 0); /* biWidth */ 00158 wk_bitmap_buf[wk_idx++] = (uint8_t)(pic_width >> 8); /* biWidth */ 00159 wk_bitmap_buf[wk_idx++] = (uint8_t)(pic_width >> 16); /* biWidth */ 00160 wk_bitmap_buf[wk_idx++] = (uint8_t)(pic_width >> 24); /* biWidth */ 00161 wk_bitmap_buf[wk_idx++] = (uint8_t)((-(long)pic_height) >> 0); /* biHeight */ 00162 wk_bitmap_buf[wk_idx++] = (uint8_t)((-(long)pic_height) >> 8); /* biHeight */ 00163 wk_bitmap_buf[wk_idx++] = (uint8_t)((-(long)pic_height) >> 16); /* biHeight */ 00164 wk_bitmap_buf[wk_idx++] = (uint8_t)((-(long)pic_height) >> 24); /* biHeight */ 00165 wk_bitmap_buf[wk_idx++] = 1; /* biPlanes */ 00166 wk_bitmap_buf[wk_idx++] = 0; /* biPlanes */ 00167 wk_bitmap_buf[wk_idx++] = 32; /* biBitCount */ 00168 wk_bitmap_buf[wk_idx++] = 0; /* biBitCount */ 00169 00170 wk_bitmap_buf[wk_idx++] = 0; /* biCopmression */ 00171 wk_bitmap_buf[wk_idx++] = 0; /* biCopmression */ 00172 wk_bitmap_buf[wk_idx++] = 0; /* biCopmression */ 00173 wk_bitmap_buf[wk_idx++] = 0; /* biCopmression */ 00174 wk_bitmap_buf[wk_idx++] = (uint8_t)(pic_size >> 0); /* biSizeImage */ 00175 wk_bitmap_buf[wk_idx++] = (uint8_t)(pic_size >> 8); /* biSizeImage */ 00176 wk_bitmap_buf[wk_idx++] = (uint8_t)(pic_size >> 16); /* biSizeImage */ 00177 wk_bitmap_buf[wk_idx++] = (uint8_t)(pic_size >> 24); /* biSizeImage */ 00178 wk_bitmap_buf[wk_idx++] = 0; /* biXPixPerMeter */ 00179 wk_bitmap_buf[wk_idx++] = 0; /* biXPixPerMeter */ 00180 wk_bitmap_buf[wk_idx++] = 0; /* biXPixPerMeter */ 00181 wk_bitmap_buf[wk_idx++] = 0; /* biXPixPerMeter */ 00182 wk_bitmap_buf[wk_idx++] = 0; /* biYPixPerMeter */ 00183 wk_bitmap_buf[wk_idx++] = 0; /* biYPixPerMeter */ 00184 wk_bitmap_buf[wk_idx++] = 0; /* biYPixPerMeter */ 00185 wk_bitmap_buf[wk_idx++] = 0; /* biYPixPerMeter */ 00186 00187 wk_bitmap_buf[wk_idx++] = 0; /* biClrUsed */ 00188 wk_bitmap_buf[wk_idx++] = 0; /* biClrUsed */ 00189 wk_bitmap_buf[wk_idx++] = 0; /* biClrUsed */ 00190 wk_bitmap_buf[wk_idx++] = 0; /* biClrUsed */ 00191 wk_bitmap_buf[wk_idx++] = 0; /* biCirImportant */ 00192 wk_bitmap_buf[wk_idx++] = 0; /* biCirImportant */ 00193 wk_bitmap_buf[wk_idx++] = 0; /* biCirImportant */ 00194 wk_bitmap_buf[wk_idx++] = 0; /* biCirImportant */ 00195 PcApp.send(wk_bitmap_buf, wk_idx); 00196 00197 SendData(buf, pic_size); 00198 wk_idx += pic_size; 00199 00200 return wk_idx; 00201 }; 00202 00203 void DisplayApp::SetCallback(Callback<void()> func) { 00204 event = func; 00205 } 00206 00207 int DisplayApp::SendJpeg(uint8_t * buf, uint32_t size) { 00208 if (!PcApp.configured()) { 00209 return 0; 00210 } 00211 SendHeader(size); 00212 SendData(buf, size); 00213 00214 return size; 00215 } 00216 00217 int DisplayApp::GetMaxTouchNum(void) { 00218 return 1; 00219 } 00220 00221 int DisplayApp::GetCoordinates(int touch_buff_num, touch_pos_t * p_touch) { 00222 touch_pos_t * wk_touch; 00223 int count = 0; 00224 int x = pos_x; 00225 int y = pos_y; 00226 00227 if (touch_buff_num > 0) { 00228 count = 0; 00229 wk_touch = &p_touch[0]; 00230 wk_touch->valid = false; 00231 wk_touch->x = 0; 00232 wk_touch->y = 0; 00233 if (x >= 0) { 00234 count = 1; 00235 wk_touch->valid = true; 00236 wk_touch->x = (uint32_t)x; 00237 } 00238 if (y >= 0) { 00239 count = 1; 00240 wk_touch->valid = true; 00241 wk_touch->y = (uint32_t)y; 00242 } 00243 } 00244 00245 return count; 00246 }
Generated on Wed Jul 13 2022 05:33:36 by
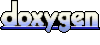