
Control a robot via a web browser, by routing the control signals via a web server.
Dependencies: HTTPClient Motordriver WiflyInterface mbed
main.cpp
00001 #include "mbed.h" 00002 #include "WiflyInterface.h" 00003 #include "motordriver.h" 00004 #include "HTTPClient.h" 00005 Serial pc(USBTX, USBRX); 00006 WiflyInterface wifly(p9, p10, p25, p26, "mbed", "password", WPA); 00007 HTTPClient http; 00008 00009 /* wifly object where: 00010 * - p9 and p10 are for the serial communication 00011 * - p25 is for the reset pin 00012 * - p26 is for the connection status 00013 * - "mbed" is the ssid of the network 00014 * - "password" is the password 00015 * - WPA is the security 00016 */ 00017 DigitalOut f(LED1); 00018 DigitalOut b(LED2); 00019 DigitalOut l(LED3); 00020 DigitalOut r(LED4); 00021 Motor A(p22, p6, p5, 1); // pwm, fwd, rev, can brake 00022 Motor B(p21, p7, p8, 1); // pwm, fwd, rev, can brake 00023 int main() { 00024 wifly.init(); // use DHCP 00025 while (!wifly.connect()); 00026 char buffer[2]; 00027 buffer[1]='\0'; 00028 char a; 00029 while(1) 00030 { 00031 00032 http.get("http://develop.jissjohn.com/netbot/command.txt", buffer,2); 00033 00034 printf("\n%s\n\r", buffer); 00035 a=buffer[0]; 00036 if(a=='1')// Forward direction 00037 {f=1; 00038 A.speed(0.4); 00039 B.speed(0.4); 00040 wait(0.02); 00041 } 00042 else if(a=='5')//Reverse Direction 00043 {b=1; 00044 f=0; 00045 l=0; 00046 r=0; 00047 A.speed(-0.4); 00048 B.speed(-0.4); 00049 wait(0.02); 00050 } 00051 else if(a=='7')//Turn Left Direction 00052 { l=1; 00053 f=0; 00054 r=0; 00055 b=0; 00056 A.speed(-0.5); 00057 B.speed(0.5); 00058 wait(0.02); 00059 } 00060 else if(a=='3')//Turn Right Direction 00061 {r=1; 00062 f=0; 00063 l=0; 00064 b=0; 00065 A.speed(0.5); 00066 B.speed(-0.5); 00067 wait(0.02); 00068 } 00069 else 00070 { 00071 00072 A.stop(1); 00073 B.stop(1); 00074 00075 wait(1); 00076 A.coast(); 00077 B.coast(); 00078 00079 } 00080 00081 } 00082 wifly.disconnect(); 00083 } 00084 00085
Generated on Mon Aug 1 2022 00:12:15 by
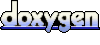