add "LE Device Address" 0x1B to advertising data types
Fork of BLE_API by
Gap.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef __GAP_H__ 00018 #define __GAP_H__ 00019 00020 #include "ble/BLEProtocol.h" 00021 #include "GapAdvertisingData.h" 00022 #include "GapAdvertisingParams.h" 00023 #include "GapScanningParams.h" 00024 #include "GapEvents.h" 00025 #include "CallChainOfFunctionPointersWithContext.h" 00026 #include "FunctionPointerWithContext.h" 00027 #include "deprecate.h" 00028 00029 /* Forward declarations for classes that will only be used for pointers or references in the following. */ 00030 class GapAdvertisingParams; 00031 class GapScanningParams; 00032 class GapAdvertisingData; 00033 00034 class Gap { 00035 /* 00036 * DEPRECATION ALERT: all of the APIs in this `public` block are deprecated. 00037 * They have been relocated to the class BLEProtocol. 00038 */ 00039 public: 00040 /** 00041 * Address-type for BLEProtocol addresses. 00042 * 00043 * @note: deprecated. Use BLEProtocol::AddressType_t instead. 00044 */ 00045 typedef BLEProtocol::AddressType_t AddressType_t; 00046 00047 /** 00048 * Address-type for BLEProtocol addresses. 00049 * @note: deprecated. Use BLEProtocol::AddressType_t instead. 00050 */ 00051 typedef BLEProtocol::AddressType_t addr_type_t; 00052 00053 /** 00054 * Address-type for BLEProtocol addresses. 00055 * \deprecated: Use BLEProtocol::AddressType_t instead. 00056 * 00057 * DEPRECATION ALERT: The following constants have been left in their 00058 * deprecated state to transparenly support existing applications which may 00059 * have used Gap::ADDR_TYPE_*. 00060 */ 00061 enum DeprecatedAddressType_t { 00062 ADDR_TYPE_PUBLIC = BLEProtocol::AddressType::PUBLIC, 00063 ADDR_TYPE_RANDOM_STATIC = BLEProtocol::AddressType::RANDOM_STATIC, 00064 ADDR_TYPE_RANDOM_PRIVATE_RESOLVABLE = BLEProtocol::AddressType::RANDOM_PRIVATE_RESOLVABLE, 00065 ADDR_TYPE_RANDOM_PRIVATE_NON_RESOLVABLE = BLEProtocol::AddressType::RANDOM_PRIVATE_NON_RESOLVABLE 00066 }; 00067 00068 static const unsigned ADDR_LEN = BLEProtocol::ADDR_LEN; /**< Length (in octets) of the BLE MAC address. */ 00069 typedef BLEProtocol::AddressBytes_t Address_t; /**< 48-bit address, LSB format. @Note: Deprecated. Use BLEProtocol::AddressBytes_t instead. */ 00070 typedef BLEProtocol::AddressBytes_t address_t; /**< 48-bit address, LSB format. @Note: Deprecated. Use BLEProtocol::AddressBytes_t instead. */ 00071 00072 public: 00073 enum TimeoutSource_t { 00074 TIMEOUT_SRC_ADVERTISING = 0x00, /**< Advertising timeout. */ 00075 TIMEOUT_SRC_SECURITY_REQUEST = 0x01, /**< Security request timeout. */ 00076 TIMEOUT_SRC_SCAN = 0x02, /**< Scanning timeout. */ 00077 TIMEOUT_SRC_CONN = 0x03, /**< Connection timeout. */ 00078 }; 00079 00080 /** 00081 * Enumeration for disconnection reasons. The values for these reasons are 00082 * derived from Nordic's implementation, but the reasons are meant to be 00083 * independent of the transport. If you are returned a reason that is not 00084 * covered by this enumeration, please refer to the underlying 00085 * transport library. 00086 */ 00087 enum DisconnectionReason_t { 00088 CONNECTION_TIMEOUT = 0x08, 00089 REMOTE_USER_TERMINATED_CONNECTION = 0x13, 00090 REMOTE_DEV_TERMINATION_DUE_TO_LOW_RESOURCES = 0x14, /**< Remote device terminated connection due to low resources.*/ 00091 REMOTE_DEV_TERMINATION_DUE_TO_POWER_OFF = 0x15, /**< Remote device terminated connection due to power off. */ 00092 LOCAL_HOST_TERMINATED_CONNECTION = 0x16, 00093 CONN_INTERVAL_UNACCEPTABLE = 0x3B, 00094 }; 00095 00096 /** 00097 * Enumeration for whitelist advertising policy filter modes. The possible 00098 * filter modes were obtained from the Bluetooth Core Specification 00099 * 4.2 (Vol. 6), Part B, Section 4.3.2. 00100 * 00101 * @experimental 00102 */ 00103 enum AdvertisingPolicyMode_t { 00104 ADV_POLICY_IGNORE_WHITELIST = 0, 00105 ADV_POLICY_FILTER_SCAN_REQS = 1, 00106 ADV_POLICY_FILTER_CONN_REQS = 2, 00107 ADV_POLICY_FILTER_ALL_REQS = 3, 00108 }; 00109 00110 /** 00111 * Enumeration for whitelist scanning policy filter modes. The possible 00112 * filter modes were obtained from the Bluetooth Core Specification 00113 * 4.2 (Vol. 6), Part B, Section 4.3.3. 00114 * 00115 * @experimental 00116 */ 00117 enum ScanningPolicyMode_t { 00118 SCAN_POLICY_IGNORE_WHITELIST = 0, 00119 SCAN_POLICY_FILTER_ALL_ADV = 1, 00120 }; 00121 00122 /** 00123 * Enumeration for the whitelist initiator policy fiter modes. The possible 00124 * filter modes were obtained from the Bluetooth Core Specification 00125 * 4.2 (vol. 6), Part B, Section 4.4.4. 00126 * 00127 * @experimental 00128 */ 00129 enum InitiatorPolicyMode_t { 00130 INIT_POLICY_IGNORE_WHITELIST = 0, 00131 INIT_POLICY_FILTER_ALL_ADV = 1, 00132 }; 00133 00134 /** 00135 * Representation of a Bluetooth Low Enery Whitelist containing addresses. 00136 * 00137 * @experimental 00138 */ 00139 struct Whitelist_t { 00140 BLEProtocol::Address_t *addresses; 00141 uint8_t size; 00142 uint8_t capacity; 00143 }; 00144 00145 00146 /* Describes the current state of the device (more than one bit can be set). */ 00147 struct GapState_t { 00148 unsigned advertising : 1; /**< Peripheral is currently advertising. */ 00149 unsigned connected : 1; /**< Peripheral is connected to a central. */ 00150 }; 00151 00152 typedef uint16_t Handle_t; /* Type for connection handle. */ 00153 00154 typedef struct { 00155 uint16_t minConnectionInterval; /**< Minimum Connection Interval in 1.25 ms units, see @ref BLE_GAP_CP_LIMITS.*/ 00156 uint16_t maxConnectionInterval; /**< Maximum Connection Interval in 1.25 ms units, see @ref BLE_GAP_CP_LIMITS.*/ 00157 uint16_t slaveLatency; /**< Slave Latency in number of connection events, see @ref BLE_GAP_CP_LIMITS.*/ 00158 uint16_t connectionSupervisionTimeout; /**< Connection Supervision Timeout in 10 ms units, see @ref BLE_GAP_CP_LIMITS.*/ 00159 } ConnectionParams_t; 00160 00161 enum Role_t { 00162 PERIPHERAL = 0x1, /**< Peripheral Role. */ 00163 CENTRAL = 0x2, /**< Central Role. */ 00164 }; 00165 00166 struct AdvertisementCallbackParams_t { 00167 BLEProtocol::AddressBytes_t peerAddr; 00168 int8_t rssi; 00169 bool isScanResponse; 00170 GapAdvertisingParams::AdvertisingType_t type; 00171 uint8_t advertisingDataLen; 00172 const uint8_t *advertisingData; 00173 }; 00174 typedef FunctionPointerWithContext<const AdvertisementCallbackParams_t *> AdvertisementReportCallback_t; 00175 00176 struct ConnectionCallbackParams_t { 00177 Handle_t handle; 00178 Role_t role; 00179 BLEProtocol::AddressType_t peerAddrType; 00180 BLEProtocol::AddressBytes_t peerAddr; 00181 BLEProtocol::AddressType_t ownAddrType; 00182 BLEProtocol::AddressBytes_t ownAddr; 00183 const ConnectionParams_t *connectionParams; 00184 00185 ConnectionCallbackParams_t(Handle_t handleIn, 00186 Role_t roleIn, 00187 BLEProtocol::AddressType_t peerAddrTypeIn, 00188 const uint8_t *peerAddrIn, 00189 BLEProtocol::AddressType_t ownAddrTypeIn, 00190 const uint8_t *ownAddrIn, 00191 const ConnectionParams_t *connectionParamsIn) : 00192 handle(handleIn), 00193 role(roleIn), 00194 peerAddrType(peerAddrTypeIn), 00195 peerAddr(), 00196 ownAddrType(ownAddrTypeIn), 00197 ownAddr(), 00198 connectionParams(connectionParamsIn) { 00199 memcpy(peerAddr, peerAddrIn, ADDR_LEN); 00200 memcpy(ownAddr, ownAddrIn, ADDR_LEN); 00201 } 00202 }; 00203 00204 struct DisconnectionCallbackParams_t { 00205 Handle_t handle; 00206 DisconnectionReason_t reason; 00207 00208 DisconnectionCallbackParams_t(Handle_t handleIn, 00209 DisconnectionReason_t reasonIn) : 00210 handle(handleIn), 00211 reason(reasonIn) 00212 {} 00213 }; 00214 00215 static const uint16_t UNIT_1_25_MS = 1250; /**< Number of microseconds in 1.25 milliseconds. */ 00216 static uint16_t MSEC_TO_GAP_DURATION_UNITS(uint32_t durationInMillis) { 00217 return (durationInMillis * 1000) / UNIT_1_25_MS; 00218 } 00219 00220 typedef FunctionPointerWithContext<TimeoutSource_t> TimeoutEventCallback_t; 00221 typedef CallChainOfFunctionPointersWithContext<TimeoutSource_t> TimeoutEventCallbackChain_t; 00222 00223 typedef FunctionPointerWithContext<const ConnectionCallbackParams_t *> ConnectionEventCallback_t; 00224 typedef CallChainOfFunctionPointersWithContext<const ConnectionCallbackParams_t *> ConnectionEventCallbackChain_t; 00225 00226 typedef FunctionPointerWithContext<const DisconnectionCallbackParams_t*> DisconnectionEventCallback_t; 00227 typedef CallChainOfFunctionPointersWithContext<const DisconnectionCallbackParams_t*> DisconnectionEventCallbackChain_t; 00228 00229 typedef FunctionPointerWithContext<bool> RadioNotificationEventCallback_t; 00230 00231 typedef FunctionPointerWithContext<const Gap *> GapShutdownCallback_t; 00232 typedef CallChainOfFunctionPointersWithContext<const Gap *> GapShutdownCallbackChain_t; 00233 00234 /* 00235 * The following functions are meant to be overridden in the platform-specific sub-class. 00236 */ 00237 public: 00238 /** 00239 * Set the BTLE MAC address and type. Please note that the address format is 00240 * least significant byte first (LSB). Please refer to BLEProtocol::AddressBytes_t. 00241 * 00242 * @return BLE_ERROR_NONE on success. 00243 */ 00244 virtual ble_error_t setAddress(BLEProtocol::AddressType_t type, const BLEProtocol::AddressBytes_t address) { 00245 /* avoid compiler warnings about unused variables */ 00246 (void)type; 00247 (void)address; 00248 00249 return BLE_ERROR_NOT_IMPLEMENTED; /* Requesting action from porter(s): override this API if this capability is supported. */ 00250 } 00251 00252 /** 00253 * Fetch the BTLE MAC address and type. 00254 * 00255 * @return BLE_ERROR_NONE on success. 00256 */ 00257 virtual ble_error_t getAddress(BLEProtocol::AddressType_t *typeP, BLEProtocol::AddressBytes_t address) { 00258 /* Avoid compiler warnings about unused variables. */ 00259 (void)typeP; 00260 (void)address; 00261 00262 return BLE_ERROR_NOT_IMPLEMENTED; /* Requesting action from porter(s): override this API if this capability is supported. */ 00263 } 00264 00265 /** 00266 * @return Minimum Advertising interval in milliseconds for connectable 00267 * undirected and connectable directed event types. 00268 */ 00269 virtual uint16_t getMinAdvertisingInterval(void) const { 00270 return 0; /* Requesting action from porter(s): override this API if this capability is supported. */ 00271 } 00272 00273 /** 00274 * @return Minimum Advertising interval in milliseconds for scannable 00275 * undirected and non-connectable undirected event types. 00276 */ 00277 virtual uint16_t getMinNonConnectableAdvertisingInterval(void) const { 00278 return 0; /* Requesting action from porter(s): override this API if this capability is supported. */ 00279 } 00280 00281 /** 00282 * @return Maximum Advertising interval in milliseconds. 00283 */ 00284 virtual uint16_t getMaxAdvertisingInterval(void) const { 00285 return 0xFFFF; /* Requesting action from porter(s): override this API if this capability is supported. */ 00286 } 00287 00288 virtual ble_error_t stopAdvertising(void) { 00289 return BLE_ERROR_NOT_IMPLEMENTED; /* Requesting action from porter(s): override this API if this capability is supported. */ 00290 } 00291 00292 /** 00293 * Stop scanning. The current scanning parameters remain in effect. 00294 * 00295 * @retval BLE_ERROR_NONE if successfully stopped scanning procedure. 00296 */ 00297 virtual ble_error_t stopScan() { 00298 return BLE_ERROR_NOT_IMPLEMENTED; /* Requesting action from porter(s): override this API if this capability is supported. */ 00299 } 00300 00301 /** 00302 * Create a connection (GAP Link Establishment). 00303 * 00304 * @param peerAddr 00305 * 48-bit address, LSB format. 00306 * @param peerAddrType 00307 * Address type of the peer. 00308 * @param connectionParams 00309 * Connection parameters. 00310 * @param scanParams 00311 * Paramters to be used while scanning for the peer. 00312 * @return BLE_ERROR_NONE if connection establishment procedure is started 00313 * successfully. The connectionCallChain (if set) will be invoked upon 00314 * a connection event. 00315 */ 00316 virtual ble_error_t connect(const BLEProtocol::AddressBytes_t peerAddr, 00317 BLEProtocol::AddressType_t peerAddrType, 00318 const ConnectionParams_t *connectionParams, 00319 const GapScanningParams *scanParams) { 00320 /* Avoid compiler warnings about unused variables. */ 00321 (void)peerAddr; 00322 (void)peerAddrType; 00323 (void)connectionParams; 00324 (void)scanParams; 00325 00326 return BLE_ERROR_NOT_IMPLEMENTED; /* Requesting action from porter(s): override this API if this capability is supported. */ 00327 } 00328 00329 /** 00330 * Create a connection (GAP Link Establishment). 00331 * 00332 * \deprecated: This funtion overloads Gap::connect(const BLEProtocol::Address_t peerAddr, 00333 BLEProtocol::AddressType_t peerAddrType, 00334 const ConnectionParams_t *connectionParams, 00335 const GapScanningParams *scanParams) 00336 * to maintain backward compatibility for change from Gap::AddressType_t to BLEProtocol::AddressType_t 00337 */ 00338 ble_error_t connect(const BLEProtocol::AddressBytes_t peerAddr, 00339 DeprecatedAddressType_t peerAddrType, 00340 const ConnectionParams_t *connectionParams, 00341 const GapScanningParams *scanParams) 00342 __deprecated_message("Gap::DeprecatedAddressType_t is deprecated, use BLEProtocol::AddressType_t instead") { 00343 return connect(peerAddr, (BLEProtocol::AddressType_t) peerAddrType, connectionParams, scanParams); 00344 } 00345 00346 /** 00347 * This call initiates the disconnection procedure, and its completion will 00348 * be communicated to the application with an invocation of the 00349 * disconnectionCallback. 00350 * 00351 * @param reason 00352 * The reason for disconnection; to be sent back to the peer. 00353 */ 00354 virtual ble_error_t disconnect(Handle_t connectionHandle, DisconnectionReason_t reason) { 00355 /* avoid compiler warnings about unused variables */ 00356 (void)connectionHandle; 00357 (void)reason; 00358 00359 return BLE_ERROR_NOT_IMPLEMENTED; /* Requesting action from porter(s): override this API if this capability is supported. */ 00360 } 00361 00362 /** 00363 * This call initiates the disconnection procedure, and its completion will 00364 * be communicated to the application with an invocation of the 00365 * disconnectionCallback. 00366 * 00367 * @param reason 00368 * The reason for disconnection; to be sent back to the peer. 00369 * 00370 * @note: This version of disconnect() doesn't take a connection handle. It 00371 * works reliably only for stacks that are limited to a single 00372 * connection. This API should be considered *deprecated* in favour of the 00373 * alternative, which takes a connection handle. It will be dropped in the future. 00374 */ 00375 virtual ble_error_t disconnect(DisconnectionReason_t reason) { 00376 /* Avoid compiler warnings about unused variables. */ 00377 (void)reason; 00378 00379 return BLE_ERROR_NOT_IMPLEMENTED; /* Requesting action from porter(s): override this API if this capability is supported. */ 00380 } 00381 00382 /** 00383 * Get the GAP peripheral preferred connection parameters. These are the 00384 * defaults that the peripheral would like to have in a connection. The 00385 * choice of the connection parameters is eventually up to the central. 00386 * 00387 * @param[out] params 00388 * The structure where the parameters will be stored. Memory 00389 * for this is owned by the caller. 00390 * 00391 * @return BLE_ERROR_NONE if the parameters were successfully filled into 00392 * the given structure pointed to by params. 00393 */ 00394 virtual ble_error_t getPreferredConnectionParams(ConnectionParams_t *params) { 00395 /* Avoid compiler warnings about unused variables. */ 00396 (void)params; 00397 00398 return BLE_ERROR_NOT_IMPLEMENTED; /* Requesting action from porter(s): override this API if this capability is supported. */ 00399 } 00400 00401 /** 00402 * Set the GAP peripheral preferred connection parameters. These are the 00403 * defaults that the peripheral would like to have in a connection. The 00404 * choice of the connection parameters is eventually up to the central. 00405 * 00406 * @param[in] params 00407 * The structure containing the desired parameters. 00408 */ 00409 virtual ble_error_t setPreferredConnectionParams(const ConnectionParams_t *params) { 00410 /* Avoid compiler warnings about unused variables. */ 00411 (void)params; 00412 00413 return BLE_ERROR_NOT_IMPLEMENTED; /* Requesting action from porter(s): override this API if this capability is supported. */ 00414 } 00415 00416 /** 00417 * Update connection parameters. 00418 * In the central role this will initiate a Link Layer connection parameter update procedure. 00419 * In the peripheral role, this will send the corresponding L2CAP request and wait for 00420 * the central to perform the procedure. 00421 * 00422 * @param[in] handle 00423 * Connection Handle. 00424 * @param[in] params 00425 * Pointer to desired connection parameters. If NULL is provided on a peripheral role, 00426 * the parameters in the PPCP characteristic of the GAP service will be used instead. 00427 */ 00428 virtual ble_error_t updateConnectionParams(Handle_t handle, const ConnectionParams_t *params) { 00429 /* avoid compiler warnings about unused variables */ 00430 (void)handle; 00431 (void)params; 00432 00433 return BLE_ERROR_NOT_IMPLEMENTED; /* Requesting action from porter(s): override this API if this capability is supported. */ 00434 } 00435 00436 /** 00437 * Set the device name characteristic in the GAP service. 00438 * @param[in] deviceName 00439 * The new value for the device-name. This is a UTF-8 encoded, <b>NULL-terminated</b> string. 00440 */ 00441 virtual ble_error_t setDeviceName(const uint8_t *deviceName) { 00442 /* Avoid compiler warnings about unused variables. */ 00443 (void)deviceName; 00444 00445 return BLE_ERROR_NOT_IMPLEMENTED; /* Requesting action from porter(s): override this API if this capability is supported. */ 00446 } 00447 00448 /** 00449 * Get the value of the device name characteristic in the GAP service. 00450 * @param[out] deviceName 00451 * Pointer to an empty buffer where the UTF-8 *non NULL- 00452 * terminated* string will be placed. Set this 00453 * value to NULL in order to obtain the deviceName-length 00454 * from the 'length' parameter. 00455 * 00456 * @param[in/out] lengthP 00457 * (on input) Length of the buffer pointed to by deviceName; 00458 * (on output) the complete device name length (without the 00459 * null terminator). 00460 * 00461 * @note If the device name is longer than the size of the supplied buffer, 00462 * length will return the complete device name length, and not the 00463 * number of bytes actually returned in deviceName. The application may 00464 * use this information to retry with a suitable buffer size. 00465 */ 00466 virtual ble_error_t getDeviceName(uint8_t *deviceName, unsigned *lengthP) { 00467 /* avoid compiler warnings about unused variables */ 00468 (void)deviceName; 00469 (void)lengthP; 00470 00471 return BLE_ERROR_NOT_IMPLEMENTED; /* Requesting action from porter(s): override this API if this capability is supported. */ 00472 } 00473 00474 /** 00475 * Set the appearance characteristic in the GAP service. 00476 * @param[in] appearance 00477 * The new value for the device-appearance. 00478 */ 00479 virtual ble_error_t setAppearance(GapAdvertisingData::Appearance appearance) { 00480 /* Avoid compiler warnings about unused variables. */ 00481 (void)appearance; 00482 00483 return BLE_ERROR_NOT_IMPLEMENTED; /* Requesting action from porter(s): override this API if this capability is supported. */ 00484 } 00485 00486 /** 00487 * Get the appearance characteristic in the GAP service. 00488 * @param[out] appearance 00489 * The new value for the device-appearance. 00490 */ 00491 virtual ble_error_t getAppearance(GapAdvertisingData::Appearance *appearanceP) { 00492 /* Avoid compiler warnings about unused variables. */ 00493 (void)appearanceP; 00494 00495 return BLE_ERROR_NOT_IMPLEMENTED; /* Requesting action from porter(s): override this API if this capability is supported. */ 00496 } 00497 00498 /** 00499 * Set the radio's transmit power. 00500 * @param[in] txPower Radio transmit power in dBm. 00501 */ 00502 virtual ble_error_t setTxPower(int8_t txPower) { 00503 /* Avoid compiler warnings about unused variables. */ 00504 (void)txPower; 00505 00506 return BLE_ERROR_NOT_IMPLEMENTED; /* Requesting action from porter(s): override this API if this capability is supported. */ 00507 } 00508 00509 /** 00510 * Query the underlying stack for permitted arguments for setTxPower(). 00511 * 00512 * @param[out] valueArrayPP 00513 * Out parameter to receive the immutable array of Tx values. 00514 * @param[out] countP 00515 * Out parameter to receive the array's size. 00516 */ 00517 virtual void getPermittedTxPowerValues(const int8_t **valueArrayPP, size_t *countP) { 00518 /* Avoid compiler warnings about unused variables. */ 00519 (void)valueArrayPP; 00520 (void)countP; 00521 00522 *countP = 0; /* Requesting action from porter(s): override this API if this capability is supported. */ 00523 } 00524 00525 /** 00526 * @return Maximum size of the whitelist. 00527 * 00528 * @experimental 00529 */ 00530 virtual uint8_t getMaxWhitelistSize(void) const 00531 { 00532 return 0; 00533 } 00534 00535 /** 00536 * Get the internal whitelist to be used by the Link Layer when scanning, 00537 * advertising or initiating a connection depending on the filter policies. 00538 * 00539 * @param[in/out] whitelist 00540 * (on input) whitelist.capacity contains the maximum number 00541 * of addresses to be returned. 00542 * (on output) The populated whitelist with copies of the 00543 * addresses in the implementation's whitelist. 00544 * 00545 * @return BLE_ERROR_NONE if the implementation's whitelist was successfully 00546 * copied into the supplied reference. 00547 * 00548 * @experimental 00549 */ 00550 virtual ble_error_t getWhitelist(Whitelist_t &whitelist) const 00551 { 00552 (void) whitelist; 00553 return BLE_ERROR_NOT_IMPLEMENTED; 00554 } 00555 00556 /** 00557 * Set the internal whitelist to be used by the Link Layer when scanning, 00558 * advertising or initiating a connection depending on the filter policies. 00559 * 00560 * @param[in] whitelist 00561 * A reference to a whitelist containing the addresses to 00562 * be added to the internal whitelist. 00563 * 00564 * @return BLE_ERROR_NONE if the implementation's whitelist was successfully 00565 * populated with the addresses in the given whitelist. 00566 * 00567 * @note The whitelist must not contain addresses of type @ref 00568 * BLEProtocol::AddressType_t::RANDOM_PRIVATE_NON_RESOLVABLE, this 00569 * this will result in a @ref BLE_ERROR_INVALID_PARAM since the 00570 * remote peer might change its private address at any time and it 00571 * is not possible to resolve it. 00572 * @note If the input whitelist is larger than @ref getMaxWhitelistSize() 00573 * the @ref BLE_ERROR_PARAM_OUT_OF_RANGE is returned. 00574 * 00575 * @experimental 00576 */ 00577 virtual ble_error_t setWhitelist(const Whitelist_t &whitelist) 00578 { 00579 (void) whitelist; 00580 return BLE_ERROR_NOT_IMPLEMENTED; 00581 } 00582 00583 /** 00584 * Set the advertising policy filter mode to be used in the next call 00585 * to startAdvertising(). 00586 * 00587 * @param[in] mode 00588 * The new advertising policy filter mode. 00589 * 00590 * @return BLE_ERROR_NONE if the specified policy filter mode was set 00591 * successfully. 00592 * 00593 * @experimental 00594 */ 00595 virtual ble_error_t setAdvertisingPolicyMode(AdvertisingPolicyMode_t mode) 00596 { 00597 (void) mode; 00598 return BLE_ERROR_NOT_IMPLEMENTED; 00599 } 00600 00601 /** 00602 * Set the scan policy filter mode to be used in the next call 00603 * to startScan(). 00604 * 00605 * @param[in] mode 00606 * The new scan policy filter mode. 00607 * 00608 * @return BLE_ERROR_NONE if the specified policy filter mode was set 00609 * successfully. 00610 * 00611 * @experimental 00612 */ 00613 virtual ble_error_t setScanningPolicyMode(ScanningPolicyMode_t mode) 00614 { 00615 (void) mode; 00616 return BLE_ERROR_NOT_IMPLEMENTED; 00617 } 00618 00619 /** 00620 * Set the initiator policy filter mode to be used. 00621 * 00622 * @param[in] mode 00623 * The new initiator policy filter mode. 00624 * 00625 * @return BLE_ERROR_NONE if the specified policy filter mode was set 00626 * successfully. 00627 * 00628 * @experimental 00629 */ 00630 virtual ble_error_t setInitiatorPolicyMode(InitiatorPolicyMode_t mode) 00631 { 00632 (void) mode; 00633 return BLE_ERROR_NOT_IMPLEMENTED; 00634 } 00635 00636 /** 00637 * Get the advertising policy filter mode that will be used in the next 00638 * call to startAdvertising(). 00639 * 00640 * @return The set advertising policy filter mode. 00641 * 00642 * @experimental 00643 */ 00644 virtual AdvertisingPolicyMode_t getAdvertisingPolicyMode(void) const 00645 { 00646 return ADV_POLICY_IGNORE_WHITELIST; 00647 } 00648 00649 /** 00650 * Get the scan policy filter mode that will be used in the next 00651 * call to startScan(). 00652 * 00653 * @return The set scan policy filter mode. 00654 * 00655 * @experimental 00656 */ 00657 virtual ScanningPolicyMode_t getScanningPolicyMode(void) const 00658 { 00659 return SCAN_POLICY_IGNORE_WHITELIST; 00660 } 00661 00662 /** 00663 * Get the initiator policy filter mode that will be used. 00664 * 00665 * @return The set scan policy filter mode. 00666 * 00667 * @experimental 00668 */ 00669 virtual InitiatorPolicyMode_t getInitiatorPolicyMode(void) const 00670 { 00671 return INIT_POLICY_IGNORE_WHITELIST; 00672 } 00673 00674 00675 protected: 00676 /* Override the following in the underlying adaptation layer to provide the functionality of scanning. */ 00677 virtual ble_error_t startRadioScan(const GapScanningParams &scanningParams) { 00678 (void)scanningParams; 00679 return BLE_ERROR_NOT_IMPLEMENTED; /* Requesting action from porter(s): override this API if this capability is supported. */ 00680 } 00681 00682 /* 00683 * APIs with non-virtual implementations. 00684 */ 00685 public: 00686 /** 00687 * Returns the current GAP state of the device using a bitmask that 00688 * describes whether the device is advertising or connected. 00689 */ 00690 GapState_t getState(void) const { 00691 return state; 00692 } 00693 00694 /** 00695 * Set the GAP advertising mode to use for this device. 00696 */ 00697 void setAdvertisingType(GapAdvertisingParams::AdvertisingType_t advType) { 00698 _advParams.setAdvertisingType(advType); 00699 } 00700 00701 /** 00702 * @param[in] interval 00703 * Advertising interval in units of milliseconds. Advertising 00704 * is disabled if interval is 0. If interval is smaller than 00705 * the minimum supported value, then the minimum supported 00706 * value is used instead. This minimum value can be discovered 00707 * using getMinAdvertisingInterval(). 00708 * 00709 * This field must be set to 0 if connectionMode is equal 00710 * to ADV_CONNECTABLE_DIRECTED. 00711 * 00712 * @note: Decreasing this value will allow central devices to detect a 00713 * peripheral faster, at the expense of more power being used by the radio 00714 * due to the higher data transmit rate. 00715 * 00716 * @Note: [WARNING] This API previously used 0.625ms as the unit for its 00717 * 'interval' argument. That required an explicit conversion from 00718 * milliseconds using Gap::MSEC_TO_GAP_DURATION_UNITS(). This conversion is 00719 * no longer required as the new units are milliseconds. Any application 00720 * code depending on the old semantics needs to be updated accordingly. 00721 */ 00722 void setAdvertisingInterval(uint16_t interval) { 00723 if (interval == 0) { 00724 stopAdvertising(); 00725 } else if (interval < getMinAdvertisingInterval()) { 00726 interval = getMinAdvertisingInterval(); 00727 } 00728 _advParams.setInterval(interval); 00729 } 00730 00731 /** 00732 * @param[in] timeout 00733 * Advertising timeout (in seconds) between 0x1 and 0x3FFF (1 00734 * and 16383). Use 0 to disable the advertising timeout. 00735 */ 00736 void setAdvertisingTimeout(uint16_t timeout) { 00737 _advParams.setTimeout(timeout); 00738 } 00739 00740 /** 00741 * Start advertising. 00742 */ 00743 ble_error_t startAdvertising(void) { 00744 setAdvertisingData(); /* Update the underlying stack. */ 00745 return startAdvertising(_advParams); 00746 } 00747 00748 /** 00749 * Reset any advertising payload prepared from prior calls to 00750 * accumulateAdvertisingPayload(). This automatically propagates the re- 00751 * initialized advertising payload to the underlying stack. 00752 */ 00753 void clearAdvertisingPayload(void) { 00754 _advPayload.clear(); 00755 setAdvertisingData(); 00756 } 00757 00758 /** 00759 * Accumulate an AD structure in the advertising payload. Please note that 00760 * the payload is limited to 31 bytes. The SCAN_RESPONSE message may be used 00761 * as an additional 31 bytes if the advertising payload is too 00762 * small. 00763 * 00764 * @param[in] flags 00765 * The flags to be added. Please refer to 00766 * GapAdvertisingData::Flags for valid flags. Multiple 00767 * flags may be specified in combination. 00768 */ 00769 ble_error_t accumulateAdvertisingPayload(uint8_t flags) { 00770 ble_error_t rc; 00771 if ((rc = _advPayload.addFlags(flags)) != BLE_ERROR_NONE) { 00772 return rc; 00773 } 00774 00775 return setAdvertisingData(); 00776 } 00777 00778 /** 00779 * Accumulate an AD structure in the advertising payload. Please note that 00780 * the payload is limited to 31 bytes. The SCAN_RESPONSE message may be used 00781 * as an additional 31 bytes if the advertising payload is too 00782 * small. 00783 * 00784 * @param app 00785 * The appearance of the peripheral. 00786 */ 00787 ble_error_t accumulateAdvertisingPayload(GapAdvertisingData::Appearance app) { 00788 setAppearance(app); 00789 00790 ble_error_t rc; 00791 if ((rc = _advPayload.addAppearance(app)) != BLE_ERROR_NONE) { 00792 return rc; 00793 } 00794 00795 return setAdvertisingData(); 00796 } 00797 00798 /** 00799 * Accumulate an AD structure in the advertising payload. Please note that 00800 * the payload is limited to 31 bytes. The SCAN_RESPONSE message may be used 00801 * as an additional 31 bytes if the advertising payload is too 00802 * small. 00803 * 00804 * @param power 00805 * The max transmit power to be used by the controller (in dBm). 00806 */ 00807 ble_error_t accumulateAdvertisingPayloadTxPower(int8_t power) { 00808 ble_error_t rc; 00809 if ((rc = _advPayload.addTxPower(power)) != BLE_ERROR_NONE) { 00810 return rc; 00811 } 00812 00813 return setAdvertisingData(); 00814 } 00815 00816 /** 00817 * Accumulate a variable length byte-stream as an AD structure in the 00818 * advertising payload. Please note that the payload is limited to 31 bytes. 00819 * The SCAN_RESPONSE message may be used as an additional 31 bytes if the 00820 * advertising payload is too small. 00821 * 00822 * @param type The type describing the variable length data. 00823 * @param data Data bytes. 00824 * @param len Length of data. 00825 * 00826 * @return BLE_ERROR_NONE if the advertisement payload was updated based on 00827 * matching AD type; otherwise, an appropriate error. 00828 * 00829 * @note When the specified AD type is INCOMPLETE_LIST_16BIT_SERVICE_IDS, 00830 * COMPLETE_LIST_16BIT_SERVICE_IDS, INCOMPLETE_LIST_32BIT_SERVICE_IDS, 00831 * COMPLETE_LIST_32BIT_SERVICE_IDS, INCOMPLETE_LIST_128BIT_SERVICE_IDS, 00832 * COMPLETE_LIST_128BIT_SERVICE_IDS or LIST_128BIT_SOLICITATION_IDS the 00833 * supplied value is appended to the values previously added to the 00834 * payload. 00835 */ 00836 ble_error_t accumulateAdvertisingPayload(GapAdvertisingData::DataType type, const uint8_t *data, uint8_t len) { 00837 if (type == GapAdvertisingData::COMPLETE_LOCAL_NAME) { 00838 setDeviceName(data); 00839 } 00840 00841 ble_error_t rc; 00842 if ((rc = _advPayload.addData(type, data, len)) != BLE_ERROR_NONE) { 00843 return rc; 00844 } 00845 00846 return setAdvertisingData(); 00847 } 00848 00849 /** 00850 * Update a particular ADV field in the advertising payload (based on 00851 * matching type). 00852 * 00853 * @param[in] type The ADV type field describing the variable length data. 00854 * @param[in] data Data bytes. 00855 * @param[in] len Length of data. 00856 * 00857 * @note: If advertisements are enabled, then the update will take effect immediately. 00858 * 00859 * @return BLE_ERROR_NONE if the advertisement payload was updated based on 00860 * matching AD type; otherwise, an appropriate error. 00861 */ 00862 ble_error_t updateAdvertisingPayload(GapAdvertisingData::DataType type, const uint8_t *data, uint8_t len) { 00863 if (type == GapAdvertisingData::COMPLETE_LOCAL_NAME) { 00864 setDeviceName(data); 00865 } 00866 00867 ble_error_t rc; 00868 if ((rc = _advPayload.updateData(type, data, len)) != BLE_ERROR_NONE) { 00869 return rc; 00870 } 00871 00872 return setAdvertisingData(); 00873 } 00874 00875 /** 00876 * Set up a particular, user-constructed advertisement payload for the 00877 * underlying stack. It would be uncommon for this API to be used directly; 00878 * there are other APIs to build an advertisement payload (see above). 00879 */ 00880 ble_error_t setAdvertisingPayload(const GapAdvertisingData &payload) { 00881 _advPayload = payload; 00882 return setAdvertisingData(); 00883 } 00884 00885 /** 00886 * @return Read back advertising data. Useful for storing and 00887 * restoring payload. 00888 */ 00889 const GapAdvertisingData &getAdvertisingPayload(void) const { 00890 return _advPayload; 00891 } 00892 00893 /** 00894 * Accumulate a variable length byte-stream as an AD structure in the 00895 * scanResponse payload. 00896 * 00897 * @param[in] type The type describing the variable length data. 00898 * @param[in] data Data bytes. 00899 * @param[in] len Length of data. 00900 */ 00901 ble_error_t accumulateScanResponse(GapAdvertisingData::DataType type, const uint8_t *data, uint8_t len) { 00902 ble_error_t rc; 00903 if ((rc = _scanResponse.addData(type, data, len)) != BLE_ERROR_NONE) { 00904 return rc; 00905 } 00906 00907 return setAdvertisingData(); 00908 } 00909 00910 /** 00911 * Reset any scan response prepared from prior calls to 00912 * accumulateScanResponse(). 00913 * 00914 * Note: This should be followed by a call to setAdvertisingPayload() or 00915 * startAdvertising() before the update takes effect. 00916 */ 00917 void clearScanResponse(void) { 00918 _scanResponse.clear(); 00919 setAdvertisingData(); 00920 } 00921 00922 /** 00923 * Set up parameters for GAP scanning (observer mode). 00924 * @param[in] interval 00925 * Scan interval (in milliseconds) [valid values lie between 2.5ms and 10.24s]. 00926 * @param[in] window 00927 * Scan Window (in milliseconds) [valid values lie between 2.5ms and 10.24s]. 00928 * @param[in] timeout 00929 * Scan timeout (in seconds) between 0x0001 and 0xFFFF; 0x0000 disables the timeout. 00930 * @param[in] activeScanning 00931 * Set to True if active-scanning is required. This is used to fetch the 00932 * scan response from a peer if possible. 00933 * 00934 * The scanning window divided by the interval determines the duty cycle for 00935 * scanning. For example, if the interval is 100ms and the window is 10ms, 00936 * then the controller will scan for 10 percent of the time. It is possible 00937 * to have the interval and window set to the same value. In this case, 00938 * scanning is continuous, with a change of scanning frequency once every 00939 * interval. 00940 * 00941 * Once the scanning parameters have been configured, scanning can be 00942 * enabled by using startScan(). 00943 * 00944 * @Note: The scan interval and window are recommendations to the BLE stack. 00945 */ 00946 ble_error_t setScanParams(uint16_t interval = GapScanningParams::SCAN_INTERVAL_MAX, 00947 uint16_t window = GapScanningParams::SCAN_WINDOW_MAX, 00948 uint16_t timeout = 0, 00949 bool activeScanning = false) { 00950 ble_error_t rc; 00951 if (((rc = _scanningParams.setInterval(interval)) == BLE_ERROR_NONE) && 00952 ((rc = _scanningParams.setWindow(window)) == BLE_ERROR_NONE) && 00953 ((rc = _scanningParams.setTimeout(timeout)) == BLE_ERROR_NONE)) { 00954 _scanningParams.setActiveScanning(activeScanning); 00955 return BLE_ERROR_NONE; 00956 } 00957 00958 return rc; 00959 } 00960 00961 /** 00962 * Set up the scanInterval parameter for GAP scanning (observer mode). 00963 * @param[in] interval 00964 * Scan interval (in milliseconds) [valid values lie between 2.5ms and 10.24s]. 00965 * 00966 * The scanning window divided by the interval determines the duty cycle for 00967 * scanning. For example, if the interval is 100ms and the window is 10ms, 00968 * then the controller will scan for 10 percent of the time. It is possible 00969 * to have the interval and window set to the same value. In this case, 00970 * scanning is continuous, with a change of scanning frequency once every 00971 * interval. 00972 * 00973 * Once the scanning parameters have been configured, scanning can be 00974 * enabled by using startScan(). 00975 */ 00976 ble_error_t setScanInterval(uint16_t interval) { 00977 return _scanningParams.setInterval(interval); 00978 } 00979 00980 /** 00981 * Set up the scanWindow parameter for GAP scanning (observer mode). 00982 * @param[in] window 00983 * Scan Window (in milliseconds) [valid values lie between 2.5ms and 10.24s]. 00984 * 00985 * The scanning window divided by the interval determines the duty cycle for 00986 * scanning. For example, if the interval is 100ms and the window is 10ms, 00987 * then the controller will scan for 10 percent of the time. It is possible 00988 * to have the interval and window set to the same value. In this case, 00989 * scanning is continuous, with a change of scanning frequency once every 00990 * interval. 00991 * 00992 * Once the scanning parameters have been configured, scanning can be 00993 * enabled by using startScan(). 00994 * 00995 * If scanning is already active, the updated value of scanWindow will be 00996 * propagated to the underlying BLE stack. 00997 */ 00998 ble_error_t setScanWindow(uint16_t window) { 00999 ble_error_t rc; 01000 if ((rc = _scanningParams.setWindow(window)) != BLE_ERROR_NONE) { 01001 return rc; 01002 } 01003 01004 /* If scanning is already active, propagate the new setting to the stack. */ 01005 if (scanningActive) { 01006 return startRadioScan(_scanningParams); 01007 } 01008 01009 return BLE_ERROR_NONE; 01010 } 01011 01012 /** 01013 * Set up parameters for GAP scanning (observer mode). 01014 * @param[in] timeout 01015 * Scan timeout (in seconds) between 0x0001 and 0xFFFF; 0x0000 disables the timeout. 01016 * 01017 * Once the scanning parameters have been configured, scanning can be 01018 * enabled by using startScan(). 01019 * 01020 * If scanning is already active, the updated value of scanTimeout will be 01021 * propagated to the underlying BLE stack. 01022 */ 01023 ble_error_t setScanTimeout(uint16_t timeout) { 01024 ble_error_t rc; 01025 if ((rc = _scanningParams.setTimeout(timeout)) != BLE_ERROR_NONE) { 01026 return rc; 01027 } 01028 01029 /* If scanning is already active, propagate the new settings to the stack. */ 01030 if (scanningActive) { 01031 return startRadioScan(_scanningParams); 01032 } 01033 01034 return BLE_ERROR_NONE; 01035 } 01036 01037 /** 01038 * Set up parameters for GAP scanning (observer mode). 01039 * @param[in] activeScanning 01040 * Set to True if active-scanning is required. This is used to fetch the 01041 * scan response from a peer if possible. 01042 * 01043 * Once the scanning parameters have been configured, scanning can be 01044 * enabled by using startScan(). 01045 * 01046 * If scanning is already in progress, then active-scanning will be enabled 01047 * for the underlying BLE stack. 01048 */ 01049 ble_error_t setActiveScanning(bool activeScanning) { 01050 _scanningParams.setActiveScanning(activeScanning); 01051 01052 /* If scanning is already active, propagate the new settings to the stack. */ 01053 if (scanningActive) { 01054 return startRadioScan(_scanningParams); 01055 } 01056 01057 return BLE_ERROR_NONE; 01058 } 01059 01060 /** 01061 * Start scanning (Observer Procedure) based on the parameters currently in 01062 * effect. 01063 * 01064 * @param[in] callback 01065 * The application-specific callback to be invoked upon 01066 * receiving every advertisement report. This can be passed in 01067 * as NULL, in which case scanning may not be enabled at all. 01068 */ 01069 ble_error_t startScan(void (*callback)(const AdvertisementCallbackParams_t *params)) { 01070 ble_error_t err = BLE_ERROR_NONE; 01071 if (callback) { 01072 if ((err = startRadioScan(_scanningParams)) == BLE_ERROR_NONE) { 01073 scanningActive = true; 01074 onAdvertisementReport.attach(callback); 01075 } 01076 } 01077 01078 return err; 01079 } 01080 01081 /** 01082 * Same as above, but this takes an (object, method) pair for a callback. 01083 */ 01084 template<typename T> 01085 ble_error_t startScan(T *object, void (T::*callbackMember)(const AdvertisementCallbackParams_t *params)) { 01086 ble_error_t err = BLE_ERROR_NONE; 01087 if (object && callbackMember) { 01088 if ((err = startRadioScan(_scanningParams)) == BLE_ERROR_NONE) { 01089 scanningActive = true; 01090 onAdvertisementReport.attach(object, callbackMember); 01091 } 01092 } 01093 01094 return err; 01095 } 01096 01097 /** 01098 * Initialize radio-notification events to be generated from the stack. 01099 * This API doesn't need to be called directly. 01100 * 01101 * Radio Notification is a feature that enables ACTIVE and INACTIVE 01102 * (nACTIVE) signals from the stack that notify the application when the 01103 * radio is in use. 01104 * 01105 * The ACTIVE signal is sent before the radio event starts. The nACTIVE 01106 * signal is sent at the end of the radio event. These signals can be used 01107 * by the application programmer to synchronize application logic with radio 01108 * activity. For example, the ACTIVE signal can be used to shut off external 01109 * devices, to manage peak current drawn during periods when the radio is on, 01110 * or to trigger sensor data collection for transmission in the Radio Event. 01111 * 01112 * @return BLE_ERROR_NONE on successful initialization, otherwise an error code. 01113 */ 01114 virtual ble_error_t initRadioNotification(void) { 01115 return BLE_ERROR_NOT_IMPLEMENTED; /* Requesting action from porter(s): override this API if this capability is supported. */ 01116 } 01117 01118 private: 01119 ble_error_t setAdvertisingData(void) { 01120 return setAdvertisingData(_advPayload, _scanResponse); 01121 } 01122 01123 private: 01124 virtual ble_error_t setAdvertisingData(const GapAdvertisingData &advData, const GapAdvertisingData &scanResponse) = 0; 01125 virtual ble_error_t startAdvertising(const GapAdvertisingParams &) = 0; 01126 01127 public: 01128 /** 01129 * Accessors to read back currently active advertising params. 01130 */ 01131 GapAdvertisingParams &getAdvertisingParams(void) { 01132 return _advParams; 01133 } 01134 const GapAdvertisingParams &getAdvertisingParams(void) const { 01135 return _advParams; 01136 } 01137 01138 /** 01139 * Set up a particular, user-constructed set of advertisement parameters for 01140 * the underlying stack. It would be uncommon for this API to be used 01141 * directly; there are other APIs to tweak advertisement parameters 01142 * individually. 01143 */ 01144 void setAdvertisingParams(const GapAdvertisingParams &newParams) { 01145 _advParams = newParams; 01146 } 01147 01148 /* Event callback handlers. */ 01149 public: 01150 /** 01151 * Set up a callback for timeout events. Refer to TimeoutSource_t for 01152 * possible event types. 01153 * @note It is possible to unregister callbacks using onTimeout().detach(callback) 01154 */ 01155 void onTimeout(TimeoutEventCallback_t callback) { 01156 timeoutCallbackChain.add(callback); 01157 } 01158 01159 /** 01160 * @brief provide access to the callchain of timeout event callbacks 01161 * It is possible to register callbacks using onTimeout().add(callback); 01162 * It is possible to unregister callbacks using onTimeout().detach(callback) 01163 * @return The timeout event callbacks chain 01164 */ 01165 TimeoutEventCallbackChain_t& onTimeout() { 01166 return timeoutCallbackChain; 01167 } 01168 01169 /** 01170 * Append to a chain of callbacks to be invoked upon GAP connection. 01171 * @note It is possible to unregister callbacks using onConnection().detach(callback) 01172 */ 01173 void onConnection(ConnectionEventCallback_t callback) {connectionCallChain.add(callback);} 01174 01175 template<typename T> 01176 void onConnection(T *tptr, void (T::*mptr)(const ConnectionCallbackParams_t*)) {connectionCallChain.add(tptr, mptr);} 01177 01178 /** 01179 * @brief provide access to the callchain of connection event callbacks 01180 * It is possible to register callbacks using onConnection().add(callback); 01181 * It is possible to unregister callbacks using onConnection().detach(callback) 01182 * @return The connection event callbacks chain 01183 */ 01184 ConnectionEventCallbackChain_t& onConnection() { 01185 return connectionCallChain; 01186 } 01187 01188 /** 01189 * Append to a chain of callbacks to be invoked upon GAP disconnection. 01190 * @note It is possible to unregister callbacks using onDisconnection().detach(callback) 01191 */ 01192 void onDisconnection(DisconnectionEventCallback_t callback) {disconnectionCallChain.add(callback);} 01193 01194 template<typename T> 01195 void onDisconnection(T *tptr, void (T::*mptr)(const DisconnectionCallbackParams_t*)) {disconnectionCallChain.add(tptr, mptr);} 01196 01197 /** 01198 * @brief provide access to the callchain of disconnection event callbacks 01199 * It is possible to register callbacks using onDisconnection().add(callback); 01200 * It is possible to unregister callbacks using onDisconnection().detach(callback) 01201 * @return The disconnection event callbacks chain 01202 */ 01203 DisconnectionEventCallbackChain_t& onDisconnection() { 01204 return disconnectionCallChain; 01205 } 01206 01207 /** 01208 * Set the application callback for radio-notification events. 01209 * 01210 * Radio Notification is a feature that enables ACTIVE and INACTIVE 01211 * (nACTIVE) signals from the stack that notify the application when the 01212 * radio is in use. 01213 * 01214 * The ACTIVE signal is sent before the radio event starts. The nACTIVE 01215 * signal is sent at the end of the radio event. These signals can be used 01216 * by the application programmer to synchronize application logic with radio 01217 * activity. For example, the ACTIVE signal can be used to shut off external 01218 * devices, to manage peak current drawn during periods when the radio is on, 01219 * or to trigger sensor data collection for transmission in the Radio Event. 01220 * 01221 * @param callback 01222 * The application handler to be invoked in response to a radio 01223 * ACTIVE/INACTIVE event. 01224 * 01225 * Or in the other version: 01226 * 01227 * @param tptr 01228 * Pointer to the object of a class defining the member callback 01229 * function (mptr). 01230 * @param mptr 01231 * The member callback (within the context of an object) to be 01232 * invoked in response to a radio ACTIVE/INACTIVE event. 01233 */ 01234 void onRadioNotification(void (*callback)(bool param)) { 01235 radioNotificationCallback.attach(callback); 01236 } 01237 template <typename T> 01238 void onRadioNotification(T *tptr, void (T::*mptr)(bool)) { 01239 radioNotificationCallback.attach(tptr, mptr); 01240 } 01241 01242 /** 01243 * Setup a callback to be invoked to notify the user application that the 01244 * Gap instance is about to shutdown (possibly as a result of a call 01245 * to BLE::shutdown()). 01246 * 01247 * @Note: It is possible to chain together multiple onShutdown callbacks 01248 * (potentially from different modules of an application) to be notified 01249 * before the Gap instance is shutdown. 01250 * 01251 * @Note: It is also possible to set up a callback into a member function of 01252 * some object. 01253 * 01254 * @Note It is possible to unregister a callback using onShutdown().detach(callback) 01255 */ 01256 void onShutdown(const GapShutdownCallback_t& callback) { 01257 shutdownCallChain.add(callback); 01258 } 01259 template <typename T> 01260 void onShutdown(T *objPtr, void (T::*memberPtr)(void)) { 01261 shutdownCallChain.add(objPtr, memberPtr); 01262 } 01263 01264 /** 01265 * @brief provide access to the callchain of shutdown event callbacks 01266 * It is possible to register callbacks using onShutdown().add(callback); 01267 * It is possible to unregister callbacks using onShutdown().detach(callback) 01268 * @return The shutdown event callbacks chain 01269 */ 01270 GapShutdownCallbackChain_t& onShutdown() { 01271 return shutdownCallChain; 01272 } 01273 01274 public: 01275 /** 01276 * Notify all registered onShutdown callbacks that the Gap instance is 01277 * about to be shutdown and clear all Gap state of the 01278 * associated object. 01279 * 01280 * This function is meant to be overridden in the platform-specific 01281 * sub-class. Nevertheless, the sub-class is only expected to reset its 01282 * state and not the data held in Gap members. This shall be achieved by a 01283 * call to Gap::reset() from the sub-class' reset() implementation. 01284 * 01285 * @return BLE_ERROR_NONE on success. 01286 * 01287 * @note: Currently a call to reset() does not reset the advertising and 01288 * scan parameters to default values. 01289 */ 01290 virtual ble_error_t reset(void) { 01291 /* Notify that the instance is about to shutdown */ 01292 shutdownCallChain.call(this); 01293 shutdownCallChain.clear(); 01294 01295 /* Clear Gap state */ 01296 state.advertising = 0; 01297 state.connected = 0; 01298 01299 /* Clear scanning state */ 01300 scanningActive = false; 01301 01302 /* Clear advertising and scanning data */ 01303 _advPayload.clear(); 01304 _scanResponse.clear(); 01305 01306 /* Clear callbacks */ 01307 timeoutCallbackChain.clear(); 01308 connectionCallChain.clear(); 01309 disconnectionCallChain.clear(); 01310 radioNotificationCallback = NULL; 01311 onAdvertisementReport = NULL; 01312 01313 return BLE_ERROR_NONE; 01314 } 01315 01316 protected: 01317 Gap() : 01318 _advParams(), 01319 _advPayload(), 01320 _scanningParams(), 01321 _scanResponse(), 01322 state(), 01323 scanningActive(false), 01324 timeoutCallbackChain(), 01325 radioNotificationCallback(), 01326 onAdvertisementReport(), 01327 connectionCallChain(), 01328 disconnectionCallChain() { 01329 _advPayload.clear(); 01330 _scanResponse.clear(); 01331 } 01332 01333 /* Entry points for the underlying stack to report events back to the user. */ 01334 public: 01335 void processConnectionEvent(Handle_t handle, 01336 Role_t role, 01337 BLEProtocol::AddressType_t peerAddrType, 01338 const BLEProtocol::AddressBytes_t peerAddr, 01339 BLEProtocol::AddressType_t ownAddrType, 01340 const BLEProtocol::AddressBytes_t ownAddr, 01341 const ConnectionParams_t *connectionParams) { 01342 state.connected = 1; 01343 ConnectionCallbackParams_t callbackParams(handle, role, peerAddrType, peerAddr, ownAddrType, ownAddr, connectionParams); 01344 connectionCallChain.call(&callbackParams); 01345 } 01346 01347 void processDisconnectionEvent(Handle_t handle, DisconnectionReason_t reason) { 01348 state.connected = 0; 01349 DisconnectionCallbackParams_t callbackParams(handle, reason); 01350 disconnectionCallChain.call(&callbackParams); 01351 } 01352 01353 void processAdvertisementReport(const BLEProtocol::AddressBytes_t peerAddr, 01354 int8_t rssi, 01355 bool isScanResponse, 01356 GapAdvertisingParams::AdvertisingType_t type, 01357 uint8_t advertisingDataLen, 01358 const uint8_t *advertisingData) { 01359 AdvertisementCallbackParams_t params; 01360 memcpy(params.peerAddr, peerAddr, ADDR_LEN); 01361 params.rssi = rssi; 01362 params.isScanResponse = isScanResponse; 01363 params.type = type; 01364 params.advertisingDataLen = advertisingDataLen; 01365 params.advertisingData = advertisingData; 01366 onAdvertisementReport.call(¶ms); 01367 } 01368 01369 void processTimeoutEvent(TimeoutSource_t source) { 01370 if (timeoutCallbackChain) { 01371 timeoutCallbackChain(source); 01372 } 01373 } 01374 01375 protected: 01376 GapAdvertisingParams _advParams; 01377 GapAdvertisingData _advPayload; 01378 GapScanningParams _scanningParams; 01379 GapAdvertisingData _scanResponse; 01380 01381 GapState_t state; 01382 bool scanningActive; 01383 01384 protected: 01385 TimeoutEventCallbackChain_t timeoutCallbackChain; 01386 RadioNotificationEventCallback_t radioNotificationCallback; 01387 AdvertisementReportCallback_t onAdvertisementReport; 01388 ConnectionEventCallbackChain_t connectionCallChain; 01389 DisconnectionEventCallbackChain_t disconnectionCallChain; 01390 01391 private: 01392 GapShutdownCallbackChain_t shutdownCallChain; 01393 01394 private: 01395 /* Disallow copy and assignment. */ 01396 Gap(const Gap &); 01397 Gap& operator=(const Gap &); 01398 }; 01399 01400 #endif // ifndef __GAP_H__
Generated on Fri Jul 15 2022 20:49:45 by
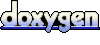