Common stuff for all my devices' web server pages: css, login, log, ipv4, ipv6, firmware update, clock, reset info etc.
Dependents: oldheating gps motorhome heating
web.c
00001 #include "http.h" 00002 #include "web-server-base.h" 00003 #include "web-server-this.h" 00004 #include "web.h" 00005 #include "web-pages-base.h" 00006 #include "http-connection.h" 00007 #include "log.h" 00008 #include "mstimer.h" 00009 00010 #define LOGIN_DELAY_MS 200 00011 00012 #define DO_LOGIN DO_SERVER + 0 00013 00014 bool WebTrace = false; 00015 00016 int WebDecideWhatToDo(char *pPath, char* pLastModified) 00017 { 00018 if (HttpSameStr(pPath, "/login")) return DO_LOGIN; 00019 00020 int todo; 00021 todo = WebServerBaseDecideWhatToDo(pPath, pLastModified); if (todo != DO_NOT_FOUND) return todo; 00022 todo = WebServerThisDecideWhatToDo(pPath, pLastModified); if (todo != DO_NOT_FOUND) return todo; 00023 return DO_NOT_FOUND; 00024 } 00025 int WebHandleQuery(char* pQuery, char* pCookies, int* pTodo, uint32_t* pDelayUntil) //return -1 on stop; 0 on continue 00026 { 00027 //If what to do is NOTHING, NOT_FOUND or NOT_MODIFIED then no query or post will be valid so stop now 00028 if (*pTodo < DO_LOGIN) return -1; 00029 00030 //If what to do is LOGIN then the user has just returned the login form 00031 if (*pTodo == DO_LOGIN) 00032 { 00033 WebLoginQuery(pQuery); //Read the password and the original location 00034 if (WebLoginQueryPasswordOk) 00035 { 00036 if (!WebLoginSessionIdIsSet()) //If there isn't a session id already 00037 { 00038 WebLoginSessionIdNew(); //Create a new session id 00039 } 00040 *pTodo = WebLoginOriginalToDo; //Load the original todo and SEND_SESSION_ID 00041 *pTodo += DO_SEND_SESSION_ID; 00042 } 00043 *pDelayUntil = MsTimerCount + LOGIN_DELAY_MS; //To prevent brute forcing the hash delay the reply to the login 00044 return -1; //Either way no query or post will be valid 00045 } 00046 00047 //Have a normal request so authenticate 00048 if (!WebLoginCookiesContainValidSessionId(pCookies)) 00049 { 00050 WebLoginOriginalToDo = *pTodo; //Record the original destination for redirection 00051 *pTodo = DO_LOGIN; 00052 return -1; //Ignore any query or post as the user is not authenticated 00053 } 00054 00055 if (WebServerBaseHandleQuery(*pTodo, pQuery)) return 0; 00056 if (WebServerThisHandleQuery(*pTodo, pQuery)) return 0; 00057 return 0; 00058 } 00059 void WebHandlePost(int todo, int contentLength, int contentStart, int size, char* pRequestStream, uint32_t positionInRequestStream, bool* pComplete) 00060 { 00061 if (WebServerBasePost(todo, contentLength, contentStart, size, pRequestStream, positionInRequestStream, pComplete)) return; 00062 if (WebServerThisPost(todo, contentLength, contentStart, size, pRequestStream, positionInRequestStream, pComplete)) return; 00063 *pComplete = true; 00064 } 00065 00066 void WebAddResponse(int todo) 00067 { 00068 //Check if todo includes the need to send a cookie 00069 if (todo >= DO_SEND_SESSION_ID) 00070 { 00071 HttpOkCookieName = WebLoginSessionNameGet(); 00072 HttpOkCookieValue = WebLoginSessionIdGet(); 00073 HttpOkCookieMaxAge = WebLoginSessionNameLife(); 00074 todo -= DO_SEND_SESSION_ID; 00075 } 00076 else 00077 { 00078 HttpOkCookieName = NULL; 00079 HttpOkCookieValue = NULL; 00080 HttpOkCookieMaxAge = -1; 00081 } 00082 00083 //Try all the base modules 00084 switch (todo) 00085 { 00086 case DO_LOGIN: WebLoginHtml (); return; 00087 case DO_NOT_FOUND: HttpNotFound (); return; 00088 case DO_NOT_MODIFIED: HttpNotModified (); return; 00089 } 00090 00091 //If not called then call the derived (child) module 00092 if (WebServerBaseReply(todo)) return; 00093 if (WebServerThisReply(todo)) return; 00094 } 00095 00096 void WebInit() 00097 { 00098 WebLoginInit(); 00099 }
Generated on Wed Jul 13 2022 14:19:59 by
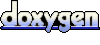