Common stuff for all my devices' web server pages: css, login, log, ipv4, ipv6, firmware update, clock, reset info etc.
Dependents: oldheating gps motorhome heating
web-net-query.c
00001 #include <string.h> 00002 00003 #include "http.h" 00004 #include "nr.h" 00005 #include "nrtest.h" 00006 #include "eth.h" 00007 #include "dns.h" 00008 00009 void WebNetQuery(char* pQuery) 00010 { 00011 while (pQuery) 00012 { 00013 char* pName; 00014 char* pValue; 00015 pQuery = HttpQuerySplit(pQuery, &pName, &pValue); 00016 HttpQueryUnencode(pValue); 00017 int value = HttpQueryValueAsInt(pValue); 00018 00019 if (HttpSameStr(pName, "name-to-resolve")) 00020 { 00021 strncpy(NrTest, pValue, NR_NAME_MAX_LENGTH); 00022 } 00023 if (HttpSameStr(pName, "request-ipv6-mdns")) 00024 { 00025 NrTestSendRequest(ETH_IPV6, DNS_PROTOCOL_MDNS); 00026 } 00027 if (HttpSameStr(pName, "request-ipv6-llmnr")) 00028 { 00029 NrTestSendRequest(ETH_IPV6, DNS_PROTOCOL_LLMNR); 00030 } 00031 if (HttpSameStr(pName, "request-ipv6-udns")) 00032 { 00033 NrTestSendRequest(ETH_IPV6, DNS_PROTOCOL_UDNS); 00034 } 00035 if (HttpSameStr(pName, "request-ipv4-mdns")) 00036 { 00037 NrTestSendRequest(ETH_IPV4, DNS_PROTOCOL_MDNS); 00038 } 00039 if (HttpSameStr(pName, "request-ipv4-llmnr")) 00040 { 00041 NrTestSendRequest(ETH_IPV4, DNS_PROTOCOL_LLMNR); 00042 } 00043 if (HttpSameStr(pName, "request-ipv4-udns")) 00044 { 00045 NrTestSendRequest(ETH_IPV4, DNS_PROTOCOL_UDNS); 00046 } 00047 } 00048 } 00049
Generated on Wed Jul 13 2022 14:19:59 by
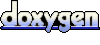