Common stuff for all my devices' web server pages: css, login, log, ipv4, ipv6, firmware update, clock, reset info etc.
Dependents: oldheating gps motorhome heating
web-login-session-name.c
00001 #include "web-site-name.h" 00002 00003 #define SESSION_LIFE 10 * 365 * 24 * 60 * 60 00004 00005 //Do not use ' ', ';', '=' as they are part of the cookie protocol: name1=value1; name2=value2; name3=value3 etc 00006 static char sessionName[9]; //Limit the name to 8 characters (plus terminating zero) to keep things quick 00007 00008 char* WebLoginSessionNameGet() 00009 { 00010 return sessionName; 00011 } 00012 00013 void WebLoginSessionNameCreate() 00014 { 00015 //Make the session name from the site name - eg "Heating" and "GPS Clock" will be "heat_sid" and "gpsc_sid" 00016 int i = 0; 00017 int j = 0; 00018 while (1) 00019 { 00020 if (i >= sizeof(sessionName) - 4 - 1) break; //Leave room for the "_sid" and the terminating NUL character 00021 char c = WEB_SITE_NAME[j++]; 00022 if (!c) break; //Stop if run out of site name 00023 if (c >= 'A' && c <= 'Z') c |= 0x20; //Make lower case 00024 if (c < 'a' || c > 'z') continue; //Skip anything other than letters 00025 sessionName[i++] = c; //Add the first characters of the site name for which there is room 00026 } 00027 for (int k = 0; k < 4; k++) //Add "_sid" 00028 { 00029 sessionName[i++] = "_sid"[k]; 00030 } 00031 sessionName[i] = 0; //Add the terminating NUL character 00032 } 00033 00034 int WebLoginSessionNameLife() 00035 { 00036 return SESSION_LIFE; 00037 }
Generated on Wed Jul 13 2022 14:19:59 by
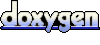