Common stuff for all my devices' web server pages: css, login, log, ipv4, ipv6, firmware update, clock, reset info etc.
Dependents: oldheating gps motorhome heating
web-clock-html.c
00001 #include <time.h> 00002 00003 #include "http.h" 00004 #include "web-nav-base.h" 00005 #include "web-add.h" 00006 00007 void WebClockHtml() 00008 { 00009 HttpOk("text/html; charset=UTF-8", "no-cache", NULL, NULL); 00010 WebAddHeader("Clock", "settings.css", "clock.js"); 00011 WebAddNav(CLOCK_PAGE); 00012 WebAddH1("Clock"); 00013 00014 WebAddH2("Status"); 00015 WebAddAjaxLed("RTC is set" , "ajax-rtc-set" ); 00016 WebAddAjaxLed("Clock is set" , "ajax-clock-set" ); 00017 WebAddAjaxLed("External source is ok", "ajax-source-ok" ); 00018 WebAddAjaxLed("Time synchronised" , "ajax-time-locked"); 00019 WebAddAjaxLed("Rate synchronised" , "ajax-rate-locked"); 00020 00021 WebAddH2("Server UTC time"); 00022 HttpAddText("<div id='ajax-date-utc'></div>\r\n"); 00023 00024 WebAddH2("Server local time"); 00025 HttpAddText("<div id='ajax-date-pc'></div>\r\n"); 00026 00027 WebAddH2("Server - PC (ms)"); 00028 HttpAddText("<div id='ajax-date-diff'></div>\r\n"); 00029 00030 WebAddH2("UTC"); 00031 WebAddAjaxInputToggle("Enable epoch change" , "ajax-leap-enable" , "chg-clock-leap-enable" ); 00032 WebAddAjaxInputToggle("Direction of next epoch" , "ajax-leap-forward" , "chg-clock-leap-forward"); 00033 WebAddAjaxInput ("Year next epoch starts" , 4, "ajax-leap-year" , "set-clock-leap-year" ); 00034 WebAddAjaxInput ("Month next epoch starts" , 4, "ajax-leap-month" , "set-clock-leap-month" ); 00035 WebAddAjaxInput ("Current era offset" , 4, "ajax-leap-count" , "set-clock-leap-count" ); 00036 00037 HttpAddText("<div><button type='button' onclick='displayLeap()'>Display leap</button></div>\r\n"); 00038 00039 HttpAddText("<div>The leap seconds list is available <a href='https://www.ietf.org/timezones/data/leap-seconds.list' target='_blank'>here</a></div>\r\n"); 00040 00041 WebAddH2("Governor"); 00042 WebAddAjaxInput ("Ppb" , 5, "ajax-ppb" , "ppb" ); 00043 WebAddAjaxInput ("Ppb divisor" , 5, "ajax-ppb-divisor" , "ppbdivisor" ); 00044 WebAddAjaxInput ("Ppb max change" , 5, "ajax-ppb-max-chg" , "ppbmaxchange" ); 00045 WebAddAjaxInput ("Ppb synced limit" , 5, "ajax-ppb-syn-lim" , "syncedlimitppb"); 00046 WebAddAjaxInput ("Ppb synced hysteresis" , 5, "ajax-ppb-syn-hys" , "syncedhysppb" ); 00047 WebAddAjaxInput ("Offset divisor" , 5, "ajax-off-divisor" , "slewdivisor" ); 00048 WebAddAjaxInput ("Offset max (ms)" , 5, "ajax-off-max" , "slewmax" ); 00049 WebAddAjaxInput ("Offset synced limit (ms)", 5, "ajax-off-syn-lim" , "syncedlimitns" ); 00050 WebAddAjaxInput ("Offset synced hys (ms)" , 5, "ajax-off-syn-hys" , "syncedhysns" ); 00051 WebAddAjaxInput ("Offset reset limit (s)" , 5, "ajax-off-rst-lim" , "maxoffsetsecs" ); 00052 WebAddAjaxInputToggle("Trace" , "ajax-gov-trace" , "clockgovtrace" ); 00053 00054 WebAddH2("NTP"); 00055 WebAddAjaxInput ("Server url" , 5, "ajax-ntp-server" , "ntpserver" ); 00056 WebAddAjaxInput ("Initial interval (s)" , 5, "ajax-ntp-initial" , "clockinitial" ); 00057 WebAddAjaxInput ("Normal interval (m)" , 5, "ajax-ntp-normal" , "clocknormal" ); 00058 WebAddAjaxInput ("Retry interval (s)" , 5, "ajax-ntp-retry" , "clockretry" ); 00059 WebAddAjaxInput ("Offset (ms)" , 5, "ajax-ntp-offset" , "clockoffset" ); 00060 WebAddAjaxInput ("Max delay (ms)" , 5, "ajax-ntp-max-delay", "clockmaxdelay" ); 00061 00062 WebAddH2("Scan times"); 00063 WebAddAjaxLabelled ("Program cycles avg", "ajax-scan-avg"); 00064 WebAddAjaxLabelled ("Program cycles max", "ajax-scan-max"); 00065 WebAddAjaxLabelled ("Program cycles min", "ajax-scan-min"); 00066 00067 WebAddEnd(); 00068 }
Generated on Wed Jul 13 2022 14:19:59 by
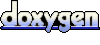