Common stuff for all my devices' web server pages: css, login, log, ipv4, ipv6, firmware update, clock, reset info etc.
Dependents: oldheating gps motorhome heating
web-clock-class.inc
00001 "//Clock class\n" 00002 "'use strict';\n" 00003 "\n" 00004 "class Clock\n" 00005 "{\n" 00006 " constructor()\n" 00007 " {\n" 00008 " this.leapEnable = false;\n" 00009 " this.leapForward = false;\n" 00010 " this.leapMonth = 0;\n" 00011 " this.leapYear = 0;\n" 00012 " this.leaps = 0;\n" 00013 " this.ms = 0;\n" 00014 " }\n" 00015 " \n" 00016 " set months1970(value)\n" 00017 " {\n" 00018 " this.leapMonth = value % 12;\n" 00019 " this.leapYear = (value - this.leapMonth) / 12;\n" 00020 " this.leapMonth += 1;\n" 00021 " this.leapYear += 1970;\n" 00022 " }\n" 00023 " get months1970()\n" 00024 " {\n" 00025 " if (this.leapYear <= 0) return 0;\n" 00026 " if (this.leapMonth <= 0) return 0;\n" 00027 " return (this.leapYear - 1970) * 12 + this.leapMonth - 1;\n" 00028 " }\n" 00029 " \n" 00030 " formatNumbers00(i)\n" 00031 " {\n" 00032 " if (i < 10) return '0' + i;\n" 00033 " return i;\n" 00034 " }\n" 00035 " formatDayOfWeek(wday)\n" 00036 " {\n" 00037 " switch(wday)\n" 00038 " {\n" 00039 " case 0: return 'Sun';\n" 00040 " case 1: return 'Mon';\n" 00041 " case 2: return 'Tue';\n" 00042 " case 3: return 'Wed';\n" 00043 " case 4: return 'Thu';\n" 00044 " case 5: return 'Fri';\n" 00045 " case 6: return 'Sat';\n" 00046 " default: return '---';\n" 00047 " }\n" 00048 " }\n" 00049 " adjustLeap(baseMs)\n" 00050 " {\n" 00051 " if (this.ms == 0) return; //Don't attempt to adjust an invalid time\n" 00052 " \n" 00053 " if (!this.leapEnable) return; // Adjustment disabled\n" 00054 " \n" 00055 " //Get the calander date and time from the ms\n" 00056 " let now = this.ms + baseMs;\n" 00057 " let leapStart = Date.UTC(this.leapYear, this.leapMonth - 1, 1, 0, 0, this.leapForward ? 0: -1);\n" 00058 " \n" 00059 " if (now < leapStart) return; //Do nothing until reached the leap start\n" 00060 " \n" 00061 " if (this.leapForward) { this.ms -= 1000; this.leaps += 1; } //repeat 59\n" 00062 " else { this.ms += 1000; this.leaps -= 1; } //skip 59\n" 00063 " \n" 00064 " this.leapEnable = false;\n" 00065 " }\n" 00066 " displayTime(baseMs)\n" 00067 " {\n" 00068 " if (this.ms == 0) return; //Don't attempt to display an invalid time\n" 00069 " \n" 00070 " //Get the calander date and time from the ms\n" 00071 " let now = new Date(this.ms + baseMs);\n" 00072 " let y = now.getUTCFullYear();\n" 00073 " let n = now.getUTCMonth () + 1;\n" 00074 " let d = now.getUTCDate ();\n" 00075 " let w = now.getUTCDay (); // 0 == Sunday\n" 00076 " let h = now.getUTCHours ();\n" 00077 " let m = now.getUTCMinutes ();\n" 00078 " let s = now.getUTCSeconds ();\n" 00079 " \n" 00080 " //Format time\n" 00081 " n = this.formatNumbers00(n);\n" 00082 " d = this.formatNumbers00(d);\n" 00083 " h = this.formatNumbers00(h);\n" 00084 " m = this.formatNumbers00(m);\n" 00085 " s = this.formatNumbers00(s);\n" 00086 " w = this.formatDayOfWeek(w);\n" 00087 " \n" 00088 " //Display time\n" 00089 " let elem;\n" 00090 " elem = document.getElementById('ajax-date-utc');\n" 00091 " if (elem) elem.textContent = y + '-' + n + '-' + d + ' ' + w + ' ' + h + ':' + m + ':' + s + ' TAI-UTC=' + this.leaps;\n" 00092 " \n" 00093 " elem = document.getElementById('ajax-date-pc');\n" 00094 " let options = \n" 00095 " {\n" 00096 " year: 'numeric',\n" 00097 " month: 'short',\n" 00098 " day: '2-digit',\n" 00099 " weekday: 'short',\n" 00100 " hour: '2-digit',\n" 00101 " minute: '2-digit',\n" 00102 " second: '2-digit',\n" 00103 " timeZoneName: 'short'\n" 00104 " };\n" 00105 " if (elem) elem.textContent = now.toLocaleString(undefined, options);\n" 00106 " }\n" 00107 "}\n" 00108 ""
Generated on Wed Jul 13 2022 14:19:59 by
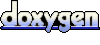