Common stuff for all my devices' web server pages: css, login, log, ipv4, ipv6, firmware update, clock, reset info etc.
Dependents: oldheating gps motorhome heating
httpsame.c
00001 #include <stdbool.h> 00002 #include <ctype.h> 00003 #include "http.h" 00004 00005 bool HttpSameStr(const char* pa, const char* pb) 00006 { 00007 if (!pa || !pb) return false; //Handle NULL references 00008 00009 while(true) 00010 { 00011 if ( *pa != *pb) return false; //If they are not the same return false 00012 if (!*pa) return true; //If finished return true; 00013 pa++; 00014 pb++; 00015 } 00016 } 00017 bool HttpSameStrCaseInsensitive(const char* pa, const char* pb) 00018 { 00019 if (!pa || !pb) return false; //Handle NULL references 00020 00021 while(true) 00022 { 00023 if ( toupper(*pa) != toupper(*pb)) return false; //If they are not the same return false 00024 if (!*pa) return true; //If finished return true; 00025 pa++; 00026 pb++; 00027 } 00028 } 00029 bool HttpSameDate(const char* date, const char* time, const char* pOtherDate) 00030 { 00031 if (!pOtherDate) return false; //Not the same if no lastModified 00032 00033 char pFileDate[HTTP_DATE_LENGTH]; 00034 HttpDateFromDateTime(date, time, pFileDate); 00035 00036 return HttpSameStr(pFileDate, pOtherDate); 00037 }
Generated on Wed Jul 13 2022 14:19:59 by
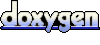