Common stuff for all my devices' web server pages: css, login, log, ipv4, ipv6, firmware update, clock, reset info etc.
Dependents: oldheating gps motorhome heating
httpquery.c
00001 #include <stdio.h> 00002 #include <stdlib.h> 00003 00004 static int hexToInt(char c) 00005 { 00006 int nibble; 00007 if (c >= '0' && c <= '9') nibble = c - '0'; 00008 if (c >= 'A' && c <= 'F') nibble = c - 'A' + 0xA; 00009 if (c >= 'a' && c <= 'f') nibble = c - 'a' + 0xA; 00010 return nibble; 00011 } 00012 void HttpQueryUnencode(char* pValue) 00013 { 00014 if (!pValue) return; 00015 char* pDst = pValue; 00016 int a; 00017 for (char* pSrc = pValue; *pSrc; pSrc++) 00018 { 00019 char c = *pSrc; 00020 switch (c) 00021 { 00022 case '+': 00023 c = ' '; 00024 break; 00025 case '%': 00026 c = *++pSrc; 00027 if (c == 0) break; 00028 a = hexToInt(c); 00029 a <<= 4; 00030 c = *++pSrc; 00031 if (c == 0) break; 00032 a += hexToInt(c); 00033 c = a; 00034 break; 00035 default: 00036 c = *pSrc; 00037 break; 00038 } 00039 *pDst++ = c; 00040 } 00041 *pDst = 0; 00042 } 00043 char* HttpQuerySplit(char* p, char** ppName, char** ppValue) //returns the start of the next name value pair 00044 { 00045 *ppName = p; //Record the start of the name 00046 *ppValue = NULL; 00047 00048 while (*p != '=') //Loop to an '=' 00049 { 00050 if (*p == 0) return 0; 00051 p++; 00052 } 00053 *p = 0; //Terminate the name by replacing the '=' with a NUL char 00054 p++; //Move on to the start of the value 00055 *ppValue = p; //Record the start of the value 00056 while (*p != '&') //Loop to a '&' 00057 { 00058 if (*p == 0) return 0; 00059 p++; 00060 } 00061 *p = 0; //Terminate the value by replacing the '&' with a NULL 00062 return p + 1; 00063 } 00064 int HttpQueryValueAsInt(char* pValue) 00065 { 00066 if (pValue) return (int)strtol(pValue, NULL, 10); 00067 return 0; 00068 } 00069 double HttpQueryValueAsDouble(char* pValue) 00070 { 00071 return strtod(pValue, NULL); 00072 } 00073 char* HttpCookiesSplit(char* p, char** ppName, char** ppValue) //returns the start of the next name value pair 00074 { 00075 *ppValue = NULL; 00076 *ppName = NULL; 00077 00078 *ppName = p; //Record the start of the name 00079 while (*p != '=') //Loop to an '=' 00080 { 00081 if (*p == 0) return 0; 00082 p++; 00083 } 00084 *p = 0; //Terminate the name by replacing the '=' with a NUL char 00085 p++; //Move on to the start of the value 00086 *ppValue = p; //Record the start of the value 00087 while (*p != ';') //Loop to a ';' 00088 { 00089 if (*p == 0) return 0; 00090 p++; 00091 } 00092 *p = 0; //Terminate the value by replacing the ';' with a NULL 00093 p++; 00094 if (*p == 0) return 0; 00095 while (*p == ' ') p++; //Move past any spaces after the ';' 00096 return p; 00097 }
Generated on Wed Jul 13 2022 14:19:59 by
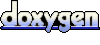