Common stuff for all my devices' web server pages: css, login, log, ipv4, ipv6, firmware update, clock, reset info etc.
Dependents: oldheating gps motorhome heating
httpadd.c
00001 #include <time.h> 00002 #include <stdio.h> 00003 #include <stdbool.h> 00004 #include <stdint.h> 00005 #include <stdarg.h> 00006 00007 static uint32_t currentPositionInMessage; 00008 static uint32_t bufferPositionInMessage; 00009 static int bufferLength; 00010 static char* pBuffer; 00011 static char* p; 00012 00013 void HttpAddStart(uint32_t position, int mss, char *pData) 00014 { 00015 currentPositionInMessage = 0; 00016 bufferPositionInMessage = position; 00017 bufferLength = mss; 00018 pBuffer = pData; 00019 p = pData; 00020 } 00021 int HttpAddLength() 00022 { 00023 return p - pBuffer; 00024 } 00025 bool HttpAddFilled() 00026 { 00027 return p - pBuffer >= bufferLength; 00028 } 00029 00030 void HttpAddChar(char c) 00031 { 00032 //Add character if the current position is within the buffer 00033 if (currentPositionInMessage >= bufferPositionInMessage && 00034 currentPositionInMessage < bufferPositionInMessage + bufferLength) *p++ = c; 00035 00036 currentPositionInMessage++; 00037 } 00038 00039 void HttpAddFillChar (char c, int length) 00040 { 00041 while (length > 0) 00042 { 00043 HttpAddChar(c); 00044 length--; 00045 } 00046 } 00047 int HttpAddText (const char* text) 00048 { 00049 const char* start = text; 00050 while (*text) 00051 { 00052 HttpAddChar(*text); 00053 text++; 00054 } 00055 return text - start; 00056 } 00057 int HttpAddTextN (const char* text, int bufferLength) 00058 { 00059 const char* start = text; 00060 while (*text && text - start < bufferLength) 00061 { 00062 HttpAddChar(*text); 00063 text++; 00064 } 00065 return text - start; 00066 } 00067 int HttpAddV (char *fmt, va_list argptr) 00068 { 00069 int size = vsnprintf(NULL, 0, fmt, argptr); //Find the size required 00070 char text[size + 1]; //Allocate enough memory for the size required with an extra byte for the terminating null 00071 vsprintf(text, fmt, argptr); //Fill the new buffer 00072 return HttpAddText(text); //Add the text 00073 } 00074 int HttpAddF (char *fmt, ...) 00075 { 00076 va_list argptr; 00077 va_start(argptr, fmt); 00078 int size = HttpAddV(fmt, argptr); 00079 va_end(argptr); 00080 return size; 00081 } 00082 void HttpAddData (const char* data, int length) 00083 { 00084 while (length > 0) 00085 { 00086 HttpAddChar(*data); 00087 data++; 00088 length--; 00089 } 00090 } 00091 void HttpAddStream(void (*startFunction)(void), int (*enumerateFunction)(void)) 00092 { 00093 startFunction(); 00094 while (true) 00095 { 00096 int c = enumerateFunction(); 00097 if (c == EOF) break; 00098 HttpAddChar(c); 00099 } 00100 } 00101 void HttpAddNibbleAsHex(int nibble) 00102 { 00103 nibble &= 0x0F; 00104 char c; 00105 if (nibble < 0x0A) c = nibble + '0'; 00106 else if (nibble < 0x10) c = nibble - 0xA + 'A'; 00107 else c = '0'; 00108 HttpAddChar(c); 00109 } 00110 void HttpAddByteAsHex(int value) 00111 { 00112 HttpAddNibbleAsHex(value >> 4); 00113 HttpAddNibbleAsHex(value >> 0); 00114 } 00115 void HttpAddInt12AsHex(int value) 00116 { 00117 HttpAddNibbleAsHex(value >> 8); 00118 HttpAddNibbleAsHex(value >> 4); 00119 HttpAddNibbleAsHex(value >> 0); 00120 } 00121 void HttpAddInt16AsHex(int value) 00122 { 00123 HttpAddNibbleAsHex(value >> 12); 00124 HttpAddNibbleAsHex(value >> 8); 00125 HttpAddNibbleAsHex(value >> 4); 00126 HttpAddNibbleAsHex(value >> 0); 00127 } 00128 void HttpAddInt32AsHex(int value) 00129 { 00130 HttpAddNibbleAsHex(value >> 28); 00131 HttpAddNibbleAsHex(value >> 24); 00132 HttpAddNibbleAsHex(value >> 20); 00133 HttpAddNibbleAsHex(value >> 16); 00134 HttpAddNibbleAsHex(value >> 12); 00135 HttpAddNibbleAsHex(value >> 8); 00136 HttpAddNibbleAsHex(value >> 4); 00137 HttpAddNibbleAsHex(value >> 0); 00138 } 00139 void HttpAddInt64AsHex(int64_t value) 00140 { 00141 HttpAddNibbleAsHex(value >> 60); 00142 HttpAddNibbleAsHex(value >> 56); 00143 HttpAddNibbleAsHex(value >> 52); 00144 HttpAddNibbleAsHex(value >> 48); 00145 HttpAddNibbleAsHex(value >> 44); 00146 HttpAddNibbleAsHex(value >> 40); 00147 HttpAddNibbleAsHex(value >> 36); 00148 HttpAddNibbleAsHex(value >> 32); 00149 HttpAddNibbleAsHex(value >> 28); 00150 HttpAddNibbleAsHex(value >> 24); 00151 HttpAddNibbleAsHex(value >> 20); 00152 HttpAddNibbleAsHex(value >> 16); 00153 HttpAddNibbleAsHex(value >> 12); 00154 HttpAddNibbleAsHex(value >> 8); 00155 HttpAddNibbleAsHex(value >> 4); 00156 HttpAddNibbleAsHex(value >> 0); 00157 } 00158 void HttpAddBytesAsHex(const uint8_t* value, int size) 00159 { 00160 int i = 0; 00161 while(true) 00162 { 00163 HttpAddByteAsHex(value[i]); 00164 i++; 00165 if (i >= size) break; 00166 if (i % 16 == 0) HttpAddText("\r\n"); 00167 else HttpAddChar(' '); 00168 } 00169 } 00170 void HttpAddBytesAsHexRev(const uint8_t* value, int size) 00171 { 00172 int i = 0; 00173 while(true) 00174 { 00175 HttpAddByteAsHex(value[size - i - 1]); 00176 i++; 00177 if (i >= size) break; 00178 if (i % 16 == 0) HttpAddText("\r\n"); 00179 else HttpAddChar(' '); 00180 } 00181 } 00182 void HttpAddTm(struct tm* ptm) 00183 { 00184 HttpAddF("%d-%02d-%02d ", ptm->tm_year + 1900, ptm->tm_mon + 1, ptm->tm_mday); 00185 switch(ptm->tm_wday) 00186 { 00187 case 0: HttpAddText("Sun"); break; 00188 case 1: HttpAddText("Mon"); break; 00189 case 2: HttpAddText("Tue"); break; 00190 case 3: HttpAddText("Wed"); break; 00191 case 4: HttpAddText("Thu"); break; 00192 case 5: HttpAddText("Fri"); break; 00193 case 6: HttpAddText("Sat"); break; 00194 default: HttpAddText("???"); break; 00195 } 00196 HttpAddF(" %02d:%02d:%02d", ptm->tm_hour, ptm->tm_min, ptm->tm_sec); 00197 if (ptm->tm_isdst > 0) HttpAddText(" BST"); 00198 else if (ptm->tm_isdst == 0) HttpAddText(" GMT"); 00199 else HttpAddText(" UTC"); 00200 }
Generated on Wed Jul 13 2022 14:19:59 by
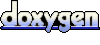