Common stuff for all my devices' web server pages: css, login, log, ipv4, ipv6, firmware update, clock, reset info etc.
Dependents: oldheating gps motorhome heating
http-connection.c
00001 #include <stdlib.h> 00002 00003 #include "http-connection.h" 00004 #include "mstimer.h" 00005 00006 #define MAX_CONNECTIONS 10 00007 00008 static struct HttpConnection connections[MAX_CONNECTIONS]; 00009 00010 static void zeroConnection(struct HttpConnection* p) 00011 { 00012 p->id = 0; 00013 p->lastUsed = 0; 00014 p->toDo = 0; 00015 p->postComplete = false; 00016 p->delayUntil = 0; 00017 } 00018 00019 struct HttpConnection* HttpConnectionNew(int connectionId) //Never fails so never returns NULL 00020 { 00021 struct HttpConnection* p; 00022 00023 //Look for an existing connection 00024 for (p = connections; p < connections + MAX_CONNECTIONS; p++) 00025 { 00026 if (p->id == connectionId) goto end; 00027 } 00028 00029 //look for an empty connection 00030 { 00031 struct HttpConnection* pOldest = 0; 00032 uint32_t ageOldest = 0; 00033 for (p = connections; p < connections + MAX_CONNECTIONS; p++) 00034 { 00035 if (!p->id) goto end; 00036 00037 //Otherwise record the oldest and keep going 00038 uint32_t age = MsTimerCount - p->lastUsed; 00039 if (age >= ageOldest) 00040 { 00041 ageOldest = age; 00042 pOldest = p; 00043 } 00044 } 00045 00046 //No empty ones found so use the oldest 00047 p = pOldest; 00048 } 00049 00050 end: 00051 zeroConnection(p); 00052 p->id = connectionId; 00053 p->lastUsed = MsTimerCount; 00054 return p; 00055 } 00056 struct HttpConnection* HttpConnectionOrNull(int connectionId) 00057 { 00058 for (struct HttpConnection* p = connections; p < connections + MAX_CONNECTIONS; p++) 00059 { 00060 if (p->id == connectionId) 00061 { 00062 p->lastUsed = MsTimerCount; 00063 return p; 00064 } 00065 } 00066 return NULL; 00067 } 00068 void HttpConnectionReset(int connectionId) 00069 { 00070 for (struct HttpConnection* p = connections; p < connections + MAX_CONNECTIONS; p++) 00071 { 00072 if (p->id == connectionId) zeroConnection(p); 00073 } 00074 }
Generated on Wed Jul 13 2022 14:19:59 by
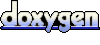