A stack which works with or without an Mbed os library. Provides IPv4 or IPv6 with a full 1500 byte buffer.
Dependents: oldheating gps motorhome heating
udptcp4.c
00001 #include <stdint.h> 00002 00003 #include "log.h" 00004 #include "net.h" 00005 #include "action.h" 00006 #include "dhcp.h" 00007 #include "eth.h" 00008 #include "ip.h" 00009 #include "ip4addr.h" 00010 #include "tcp.h" 00011 #include "tcprecv.h" 00012 #include "tcpsend.h" 00013 #include "tcphdr.h" 00014 #include "udp.h" 00015 #include "ar4.h" 00016 #include "led.h" 00017 #include "restart.h" 00018 #include "checksum.h" 00019 00020 static uint16_t calculateChecksum(uint8_t pro, uint32_t srcIp, uint32_t dstIp, int size, void* pPacket) 00021 { 00022 uint32_t sum = 0; 00023 uint16_t pro16 = pro; 00024 sum = CheckSumAddDirect(sum, 4, &srcIp ); 00025 sum = CheckSumAddDirect(sum, 4, &dstIp ); 00026 sum = CheckSumAddInvert(sum, 2, &pro16 ); 00027 sum = CheckSumAddInvert(sum, 2, &size ); 00028 return CheckSumFinDirect(sum, size, pPacket); 00029 } 00030 static void finalisePacket(uint8_t pro, int action, void* pPacket, int size, uint32_t* pSrcIp, uint32_t* pDstIp) 00031 { 00032 if (!action) return; 00033 00034 Ip4AddrFromDest(ActionGetDestPart(action), pDstIp); 00035 *pSrcIp = DhcpLocalIp; 00036 00037 switch (pro) 00038 { 00039 case TCP: TcpHdrWriteToPacket(pPacket); break; 00040 case UDP: UdpMakeHeader(size, pPacket); break; 00041 } 00042 00043 uint16_t checksum = calculateChecksum(pro, *pSrcIp, *pDstIp, size, pPacket); 00044 00045 switch (pro) 00046 { 00047 case TCP: TcpHdrSetChecksum(pPacket, checksum); break; 00048 case UDP: UdpHdrSetChecksum(pPacket, checksum); break; 00049 } 00050 00051 if (ActionGetTracePart(action)) 00052 { 00053 switch (pro) 00054 { 00055 case TCP: TcpHdrLog(0); break; 00056 case UDP: UdpLogHeader(0); break; 00057 } 00058 } 00059 } 00060 00061 static void (*pTraceBack)(void); 00062 static int tracePacketProtocol; 00063 static uint16_t calculatedChecksum; 00064 static void trace() 00065 { 00066 pTraceBack(); 00067 switch(tracePacketProtocol) 00068 { 00069 case UDP: UdpLogHeader(calculatedChecksum); break; 00070 case TCP: TcpHdrLog(calculatedChecksum); break; 00071 default: LogTimeF("UdpTcp4 - traceback unrecognised protocol %d\r\n", tracePacketProtocol); break; 00072 } 00073 } 00074 int Tcp4HandleReceivedPacket(void (*traceback)(void), void* pPacketRx, int sizeRx, void* pPacketTx, int* pSizeTx, uint32_t* pSrcIp, uint32_t* pDstIp, int remArIndex) 00075 { 00076 int lastRestartPoint = RestartPoint; 00077 RestartPoint = FAULT_POINT_Tcp4HandleReceivedPacket; 00078 00079 pTraceBack = traceback; 00080 tracePacketProtocol = TCP; 00081 calculatedChecksum = calculateChecksum(TCP, *pSrcIp, *pDstIp, sizeRx, pPacketRx); 00082 00083 int action = TcpHandleReceivedPacket(trace, sizeRx, pPacketRx, pSizeTx, pPacketTx, ETH_IPV4, remArIndex, 0); 00084 00085 *pDstIp = *pSrcIp; 00086 00087 finalisePacket(TCP, action, pPacketTx, *pSizeTx, pSrcIp, pDstIp); 00088 00089 RestartPoint = lastRestartPoint; 00090 return action; 00091 } 00092 00093 int Udp4HandleReceivedPacket(void (*traceback)(void), void* pPacketRx, int sizeRx, void* pPacketTx, int* pSizeTx, uint32_t* pSrcIp, uint32_t* pDstIp) 00094 { 00095 int lastRestartPoint = RestartPoint; 00096 RestartPoint = FAULT_POINT_Udp4HandleReceivedPacket; 00097 00098 pTraceBack = traceback; 00099 tracePacketProtocol = UDP; 00100 calculatedChecksum = calculateChecksum(UDP, *pSrcIp, *pDstIp, sizeRx, pPacketRx); 00101 00102 int action = UdpHandleReceivedPacket(trace, sizeRx, pPacketRx, pSizeTx, pPacketTx); 00103 00104 *pDstIp = *pSrcIp; 00105 00106 finalisePacket(UDP, action, pPacketTx, *pSizeTx, pSrcIp, pDstIp); //Note that the ports are reversed here 00107 00108 RestartPoint = lastRestartPoint; 00109 return action; 00110 } 00111 int Tcp4PollForPacketToSend(void* pPacket, int* pSize, uint32_t* pSrcIp, uint32_t* pDstIp) 00112 { 00113 int remArIndex = -1; 00114 int action = TcpPollForPacketToSend(pSize, pPacket, ETH_IPV4, &remArIndex, NULL); 00115 if (action && remArIndex >= 0) *pDstIp = Ar4IndexToIp(remArIndex); 00116 00117 finalisePacket(TCP, action, pPacket, *pSize, pSrcIp, pDstIp); 00118 00119 return action; 00120 } 00121 int Udp4PollForPacketToSend(void* pPacket, int* pSize, uint32_t* pSrcIp, uint32_t* pDstIp) 00122 { 00123 int action = UdpPollForPacketToSend(ETH_IPV4, pSize, pPacket); 00124 00125 finalisePacket(UDP, action, pPacket, *pSize, pSrcIp, pDstIp); 00126 00127 return action; 00128 }
Generated on Tue Jul 12 2022 18:53:40 by
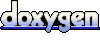