A stack which works with or without an Mbed os library. Provides IPv4 or IPv6 with a full 1500 byte buffer.
Dependents: oldheating gps motorhome heating
tcb.h
00001 #ifndef TCB_H 00002 #define TCB_H 00003 00004 #include <stdint.h> 00005 #include <stdbool.h> 00006 00007 #define TCB_EMPTY 0 00008 #define TCB_SYN_RECEIVED 1 00009 #define TCB_ESTABLISHED 2 00010 #define TCB_CLOSE_FIN_WAIT 3 00011 00012 struct tcb 00013 { 00014 int state; 00015 uint32_t timeSendsBeingAcked; //Used for RTO 00016 int countSendsNotAcked; //Used for RTO 00017 uint32_t timeLastRcvd; //Used for detect idle links 00018 int ipType; 00019 int remArIndex; //Unique per remote ip when taken with the ipType 00020 int locIpScope; //Unique per local ip 00021 uint16_t remPort; 00022 uint16_t locPort; 00023 uint32_t remMss; 00024 uint32_t window; 00025 00026 uint32_t remIsn; 00027 uint32_t bytesRcvdFromRem; 00028 uint32_t bytesAckdToRem; 00029 bool rcvdFin; 00030 00031 uint32_t locIsn; 00032 uint32_t bytesSentToRem; 00033 uint32_t bytesAckdByRem; 00034 bool sentFin; 00035 }; 00036 00037 extern uint32_t TcbGetIsn (void); 00038 extern struct tcb* TcbGetExisting(int ipType, int remArIndex, int locIpScope, uint16_t remPort, uint16_t locPort); 00039 extern struct tcb* TcbGetEmpty (void); 00040 extern struct tcb* TcbGetNext (struct tcb* pTcb); 00041 extern int TcbGetId (struct tcb* pTcb); //A unique identifier greater than 0. An id of zero means empty. 00042 extern void TcbInit (void); 00043 extern void TcbSendAjax (void); 00044 00045 #endif
Generated on Tue Jul 12 2022 18:53:40 by
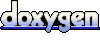