A stack which works with or without an Mbed os library. Provides IPv4 or IPv6 with a full 1500 byte buffer.
Dependents: oldheating gps motorhome heating
tcb.c
00001 #include <stdint.h> 00002 00003 #include "log.h" 00004 #include "tcp.h" 00005 #include "tcb.h" 00006 #include "http.h" 00007 #include "mstimer.h" 00008 00009 #define TCB_COUNT 10 00010 00011 struct tcb tcbs[TCB_COUNT]; 00012 00013 uint32_t TcbGetIsn() 00014 { 00015 static uint32_t isn = 0; 00016 isn += 100000; //Gives each tcb 100,000 packets and won't repeat before 42,940 tcbs. 00017 return isn; 00018 } 00019 struct tcb* TcbGetExisting(int ipType, int remArIndex, int locIpScope, uint16_t remPort, uint16_t locPort) 00020 { 00021 for (int i = 0; i < TCB_COUNT; i++) 00022 { 00023 struct tcb* pTcb = tcbs + i; 00024 if (pTcb->state && 00025 pTcb->ipType == ipType && 00026 pTcb->remArIndex == remArIndex && 00027 pTcb->locIpScope == locIpScope && 00028 pTcb->remPort == remPort && 00029 pTcb->locPort == locPort) return pTcb; 00030 } 00031 return NULL; 00032 } 00033 struct tcb* TcbGetEmpty() 00034 { 00035 for (int i = 0; i < TCB_COUNT; i++) 00036 { 00037 struct tcb* pTcb = tcbs + i; 00038 if (pTcb->state == TCB_EMPTY) return pTcb; 00039 } 00040 return NULL; 00041 } 00042 struct tcb* TcbGetNext(struct tcb* pTcb) 00043 { 00044 if (!pTcb) return tcbs; //Initialise if passed NULL 00045 ++pTcb; //Increment 00046 if (pTcb < tcbs + TCB_COUNT) return pTcb; 00047 else return tcbs; 00048 00049 } 00050 void TcbSendAjax() 00051 { 00052 for (int i = 0; i < TCB_COUNT; i++) 00053 { 00054 struct tcb* pTcb = tcbs + i; 00055 if (pTcb->state) 00056 { 00057 HttpAddByteAsHex (pTcb->state); HttpAddChar('\t'); 00058 HttpAddInt32AsHex(MsTimerCount - pTcb->timeLastRcvd); HttpAddChar('\t'); 00059 HttpAddInt16AsHex(pTcb->ipType); HttpAddChar('\t'); 00060 HttpAddInt32AsHex(pTcb->remArIndex); HttpAddChar('\t'); 00061 HttpAddInt16AsHex(pTcb->locPort); HttpAddChar('\t'); 00062 HttpAddInt16AsHex(pTcb->remPort); HttpAddChar('\t'); 00063 HttpAddInt32AsHex(pTcb->bytesRcvdFromRem); HttpAddChar('\t'); 00064 HttpAddInt32AsHex(pTcb->bytesSentToRem); HttpAddChar('\n'); 00065 } 00066 } 00067 } 00068 00069 int TcbGetId(struct tcb* pTcb) //0 means none 00070 { 00071 return pTcb - tcbs + 1; 00072 } 00073 void TcbInit() 00074 { 00075 for (int i = 0; i < TCB_COUNT; i++) tcbs[i].state = TCB_EMPTY; 00076 }
Generated on Tue Jul 12 2022 18:53:40 by
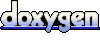