A stack which works with or without an Mbed os library. Provides IPv4 or IPv6 with a full 1500 byte buffer.
Dependents: oldheating gps motorhome heating
slaac.c
00001 #include <string.h> 00002 00003 #include "mac.h" 00004 #include "ip6addr.h" 00005 00006 char SlaacLinkLocalIp[16]; 00007 char SlaacUniqueLocalIp[16]; 00008 char SlaacGlobalIp[16]; 00009 00010 int SlaacScope(char* ip) 00011 { 00012 if (Ip6AddrIsSame(ip, SlaacLinkLocalIp)) return SCOPE_LINK_LOCAL; 00013 if (Ip6AddrIsSame(ip, SlaacGlobalIp )) return SCOPE_GLOBAL; 00014 return SCOPE_NONE; 00015 } 00016 void SlaacAddressFromScope(int scope, char* pSrcIp) 00017 { 00018 switch (scope) 00019 { 00020 case SCOPE_GLOBAL: Ip6AddrCopy(pSrcIp, SlaacGlobalIp ); break; 00021 case SCOPE_UNIQUE_LOCAL: Ip6AddrCopy(pSrcIp, SlaacUniqueLocalIp); break; 00022 default: Ip6AddrCopy(pSrcIp, SlaacLinkLocalIp ); break; 00023 } 00024 //Note that scope could be SCOPE_NONE if source was multicast in which case should return the link local ip. 00025 } 00026 00027 void SlaacMakeGlobal(char* pPrefix) 00028 { 00029 memcpy(SlaacGlobalIp, pPrefix, 8); 00030 char* p = SlaacGlobalIp + 8; 00031 *p++ = MacLocal[0] | 0x02; //Modified EUI-64 00032 *p++ = MacLocal[1]; 00033 *p++ = MacLocal[2]; 00034 *p++ = 0xFF; 00035 *p++ = 0xFE; 00036 *p++ = MacLocal[3]; 00037 *p++ = MacLocal[4]; 00038 *p++ = MacLocal[5]; 00039 00040 } 00041 void SlaacMakeUniqueLocal(char* pPrefix) 00042 { 00043 memcpy(SlaacUniqueLocalIp, pPrefix, 8); 00044 char* p = SlaacUniqueLocalIp + 8; 00045 *p++ = MacLocal[0] | 0x02; //Modified EUI-64 00046 *p++ = MacLocal[1]; 00047 *p++ = MacLocal[2]; 00048 *p++ = 0xFF; 00049 *p++ = 0xFE; 00050 *p++ = MacLocal[3]; 00051 *p++ = MacLocal[4]; 00052 *p++ = MacLocal[5]; 00053 00054 } 00055 void SlaacInit() 00056 { 00057 char* p = SlaacLinkLocalIp; //fe80::::202:f7ff:fef2:7d27 00058 *p++ = 0xFE; 00059 *p++ = 0x80; 00060 *p++ = 0x00; 00061 *p++ = 0x00; 00062 *p++ = 0x00; 00063 *p++ = 0x00; 00064 *p++ = 0x00; 00065 *p++ = 0x00; 00066 *p++ = MacLocal[0] | 0x02; //Modified EUI-64 00067 *p++ = MacLocal[1]; 00068 *p++ = MacLocal[2]; 00069 *p++ = 0xFF; 00070 *p++ = 0xFE; 00071 *p++ = MacLocal[3]; 00072 *p++ = MacLocal[4]; 00073 *p++ = MacLocal[5]; 00074 }
Generated on Tue Jul 12 2022 18:53:40 by
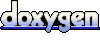