A stack which works with or without an Mbed os library. Provides IPv4 or IPv6 with a full 1500 byte buffer.
Dependents: oldheating gps motorhome heating
rs.c
00001 #include <stdint.h> 00002 #include <stdbool.h> 00003 00004 #include "log.h" 00005 #include "net.h" 00006 #include "ndp.h" 00007 #include "ip6.h" 00008 #include "ip6addr.h" 00009 #include "slaac.h" 00010 #include "action.h" 00011 #include "mac.h" 00012 #include "mstimer.h" 00013 00014 bool RsTrace = false; 00015 00016 bool RsSendSolicitation = false; 00017 00018 #define MAX_REPEAT_DELAY_TIME_MS 60000 00019 #define MIN_REPEAT_DELAY_TIME_MS 800 00020 static uint32_t repeatDelayMsTimer = (uint32_t)-MIN_REPEAT_DELAY_TIME_MS; //Initial value ensures no delay at startup 00021 static uint32_t delayMs = MIN_REPEAT_DELAY_TIME_MS; //Doubles on failure up to max; reset to min whenever an IP address request has been acknowledged 00022 00023 static void hdrSetReserved(char* pPacket, uint32_t value) { NetInvert32(pPacket + 0, &value); } 00024 static const int HEADER_LENGTH = 4; 00025 00026 static void logHeader(char* pPacket, int size) 00027 { 00028 char* pData = pPacket + HEADER_LENGTH; 00029 int dataLength = size - HEADER_LENGTH; 00030 00031 if (NetTraceVerbose) 00032 { 00033 Log("RS header\r\n"); 00034 LogF(" Size %d\r\n", size); 00035 NdpLogOptionsVerbose(pData, dataLength); 00036 } 00037 else 00038 { 00039 Log("RS header"); 00040 NdpLogOptionsQuiet(pData, dataLength); 00041 Log("\r\n"); 00042 } 00043 } 00044 int RsGetWaitingSolicitation(char* pPacket, int* pSize, uint8_t* pType, uint8_t* pCode) 00045 { 00046 //Check if time to update 00047 if (NdpIsFresh()) 00048 { 00049 delayMs = MIN_REPEAT_DELAY_TIME_MS; //Set the delay time back to minimum 00050 return DO_NOTHING; 00051 } 00052 00053 //Limit retries with a backoff delay 00054 if (!MsTimerRelative(repeatDelayMsTimer, delayMs)) return DO_NOTHING; //Don't retry within the delay time 00055 delayMs <<= 1; //Backoff (double) the delay time after each attempt 00056 if (delayMs > MAX_REPEAT_DELAY_TIME_MS) delayMs = MAX_REPEAT_DELAY_TIME_MS; //Don't go beyond a maximum 00057 repeatDelayMsTimer = MsTimerCount; //Start the delay timer 00058 00059 //Send the renewal request 00060 *pType = 133; //Router solicitation 00061 *pCode = 0; 00062 00063 hdrSetReserved(pPacket, 0); 00064 00065 char* pData = (char*)pPacket + HEADER_LENGTH; 00066 char* p = pData; 00067 p += NdpAddOptionSourceMac(p, MacLocal); 00068 00069 *pSize = HEADER_LENGTH + p - pData; 00070 00071 if (RsTrace) 00072 { 00073 if (NetTraceNewLine) Log("\r\n"); 00074 LogTime("NDP send router solicit\r\n"); 00075 logHeader(pPacket, *pSize); 00076 } 00077 00078 return ActionMakeFromDestAndTrace(MULTICAST_ROUTER, RsTrace && NetTraceStack); 00079 00080 }
Generated on Tue Jul 12 2022 18:53:40 by
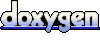