A stack which works with or without an Mbed os library. Provides IPv4 or IPv6 with a full 1500 byte buffer.
Dependents: oldheating gps motorhome heating
resolve.c
00001 #include <stdbool.h> 00002 #include "eth.h" 00003 #include "ar4.h" 00004 #include "ar6.h" 00005 #include "mac.h" 00006 #include "nr.h" 00007 #include "ip6addr.h" 00008 00009 static bool resolve4(char* server, uint32_t* pip) 00010 { 00011 //Check if have IP, if not, then request it and stop 00012 NrNameToAddress4(server, pip); 00013 if (!*pip) 00014 { 00015 NrMakeRequestForAddress4FromName(server); //The request is only repeated if made after a freeze time - call as often as you want. 00016 return false; 00017 } 00018 00019 //Check if have MAC and, if not, request it and stop 00020 char mac[6]; 00021 Ar4IpToMac(*pip, mac); 00022 if (MacIsEmpty(mac)) 00023 { 00024 Ar4MakeRequestForMacFromIp(*pip); //The request is only repeated if made after a freeze time - call as often as you want. 00025 return false; 00026 } 00027 00028 return true; 00029 } 00030 static bool resolve6(char* server, char* pip) 00031 { 00032 //Check if have IP, if not, then request it and stop 00033 NrNameToAddress6(server, pip); 00034 if (Ip6AddrIsEmpty(pip)) 00035 { 00036 NrMakeRequestForAddress6FromName(server); //The request is only repeated if made after a freeze time - call as often as you want. 00037 return false; 00038 } 00039 00040 //Check if have MAC and, if not, request it and stop 00041 char mac[6]; 00042 Ar6IpToMac(pip, mac); 00043 if (MacIsEmpty(mac)) 00044 { 00045 Ar6MakeRequestForMacFromIp(pip); //The request is only repeated if made after a freeze time - call as often as you want. 00046 return false; 00047 } 00048 00049 return true; 00050 } 00051 bool Resolve(char* remoteHost, int type, uint32_t* pIp4Address, char* pIp6Address) 00052 { 00053 if (type == ETH_IPV4) return resolve4(remoteHost, pIp4Address); 00054 if (type == ETH_IPV6) return resolve6(remoteHost, pIp6Address); 00055 return false; 00056 }
Generated on Tue Jul 12 2022 18:53:40 by
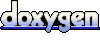