A stack which works with or without an Mbed os library. Provides IPv4 or IPv6 with a full 1500 byte buffer.
Dependents: oldheating gps motorhome heating
ra.c
00001 #include <stdint.h> 00002 #include <stdbool.h> 00003 00004 #include "log.h" 00005 #include "clk.h" 00006 #include "net.h" 00007 #include "action.h" 00008 #include "ip6.h" 00009 #include "mac.h" 00010 #include "slaac.h" 00011 #include "ndp.h" 00012 00013 bool RaTrace = false; 00014 00015 static uint8_t hdrGetHop (char* pPacket) { return *(pPacket + 0); } 00016 static uint8_t hdrGetMo (char* pPacket) { return *(pPacket + 1); } 00017 static uint16_t hdrGetLifetime (char* pPacket) { uint16_t r; NetInvert16(&r, pPacket + 2); return r; } 00018 static uint32_t hdrGetReachable(char* pPacket) { uint32_t r; NetInvert32(&r, pPacket + 4); return r; } 00019 static uint32_t hdrGetRetrans (char* pPacket) { uint32_t r; NetInvert32(&r, pPacket + 8); return r; } 00020 static const int HEADER_LENGTH = 12; 00021 00022 void logHeader(char* pPacket, int dataLength) 00023 { 00024 char* pData = pPacket + HEADER_LENGTH; 00025 if (NetTraceVerbose) 00026 { 00027 Log("RA header\r\n"); 00028 LogF(" Hop limit %d\r\n", hdrGetHop (pPacket)); 00029 LogF(" M O %x\r\n", hdrGetMo (pPacket)); 00030 LogF(" Lifetime %d\r\n", hdrGetLifetime (pPacket)); 00031 LogF(" Reachable %d\r\n", hdrGetReachable(pPacket)); 00032 LogF(" Retrans %d\r\n", hdrGetRetrans (pPacket)); 00033 NdpLogOptionsVerbose(pData, dataLength); 00034 } 00035 else 00036 { 00037 Log("RA header"); 00038 NdpLogOptionsQuiet(pData, dataLength); 00039 Log("\r\n"); 00040 } 00041 } 00042 int RaHandleReceivedAdvertisement(void (*traceback)(void), char* pPacket, int* pSize) 00043 { 00044 char* pData = pPacket + HEADER_LENGTH; 00045 int dataLength = *pSize - HEADER_LENGTH; 00046 00047 NdpHopLimit = hdrGetHop (pPacket); 00048 NdpManagedConfiguration = hdrGetMo (pPacket) & 0x80; 00049 NdpOtherConfiguration = hdrGetMo (pPacket) & 0x40; 00050 NdpSetLease (hdrGetLifetime(pPacket)); //This resets the NdpElapsedTimer 00051 00052 if (RaTrace) 00053 { 00054 if (NetTraceNewLine) Log("\r\n"); 00055 LogTimeF("NDP received router advertise\r\n"); 00056 if (NetTraceStack) traceback(); 00057 logHeader(pPacket, dataLength); 00058 } 00059 NdpDecodeOptions(pData, dataLength, NdpRouterMac, NULL); 00060 00061 return DO_NOTHING; 00062 00063 }
Generated on Tue Jul 12 2022 18:53:40 by
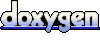