A stack which works with or without an Mbed os library. Provides IPv4 or IPv6 with a full 1500 byte buffer.
Dependents: oldheating gps motorhome heating
ntptimestamp.c
00001 #include <stdint.h> 00002 #include <stdbool.h> 00003 00004 #include "clktime.h" 00005 #include "clkutc.h" 00006 00007 #define SECONDS_BETWEEN_1900_AND_1970 2208988800ULL 00008 00009 #define ERA_BASE_LOW 1LL //Adjust this from 0 to 1 between 1968 and 2036; 1 to 2 between 2104 and 2168 00010 #define ERA_BASE_HGH 0LL //Adjust this from 0 to 1 between 2036 and 2104; 1 to 2 between 2172 and 2240 00011 00012 uint64_t NtpTimeStampFromClkTime(clktime tai) 00013 { 00014 clktime utc = ClkUtcFromTai(tai); 00015 uint64_t timestamp = utc << (32 - CLK_TIME_ONE_SECOND_SHIFT); 00016 00017 timestamp += SECONDS_BETWEEN_1900_AND_1970 << 32; //This should just wrap around the unsigned timestamp removing the unwanted era. 00018 00019 return timestamp; 00020 } 00021 clktime NtpTimeStampToClkTime(uint64_t timestamp) 00022 { 00023 bool isHigh = (timestamp & (1ULL << 63)) != 0; 00024 00025 clktime utc = timestamp - (SECONDS_BETWEEN_1900_AND_1970 << 32); 00026 utc >>= (32 - CLK_TIME_ONE_SECOND_SHIFT); 00027 00028 //Correct for era 00029 if (isHigh) utc += ERA_BASE_HGH << (32 + CLK_TIME_ONE_SECOND_SHIFT); 00030 else utc += ERA_BASE_LOW << (32 + CLK_TIME_ONE_SECOND_SHIFT); 00031 00032 clktime tai = ClkUtcToTai(utc); 00033 00034 return tai; 00035 } 00036
Generated on Tue Jul 12 2022 18:53:40 by
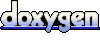