A stack which works with or without an Mbed os library. Provides IPv4 or IPv6 with a full 1500 byte buffer.
Dependents: oldheating gps motorhome heating
ntpclientquery.c
00001 #include <stdint.h> 00002 #include <string.h> 00003 #include <stdbool.h> 00004 00005 #include "log.h" 00006 #include "clk.h" 00007 #include "mstimer.h" 00008 #include "clktime.h" 00009 #include "ntptimestamp.h" 00010 #include "clkutc.h" 00011 #include "clkgov.h" 00012 #include "clktm.h" 00013 #include "net.h" 00014 #include "ntp.h" 00015 #include "ntphdr.h" 00016 #include "ntpclient.h" 00017 #include "dns.h" 00018 #include "ip4.h" 00019 #include "resolve.h" 00020 #include "action.h" 00021 #include "dnslabel.h" 00022 00023 #define ONE_BILLION 1000000000ULL 00024 #define ONE_MILLION 1000000LL 00025 00026 char NtpClientQueryServerName[DNS_MAX_LABEL_LENGTH+1]; 00027 int32_t NtpClientQueryInitialInterval; 00028 int32_t NtpClientQueryNormalInterval; 00029 int32_t NtpClientQueryRetryInterval; 00030 00031 bool NtpClientQuerySendRequestsViaIp4 = false; 00032 uint32_t NtpClientQueryServerIp4; 00033 char NtpClientQueryServerIp6[16]; 00034 00035 static uint64_t startNtpMs = 0; 00036 static int intervalTypeNtp = NTP_QUERY_INTERVAL_INITIAL; 00037 static bool intervalCompleteNtp() 00038 { 00039 uint32_t interval; 00040 switch(intervalTypeNtp) 00041 { 00042 case NTP_QUERY_INTERVAL_INITIAL: interval = NtpClientQueryInitialInterval; break; 00043 case NTP_QUERY_INTERVAL_NORMAL: interval = NtpClientQueryNormalInterval; break; 00044 case NTP_QUERY_INTERVAL_RETRY: interval = NtpClientQueryRetryInterval; break; 00045 } 00046 return MsTimerRelative(startNtpMs, interval * 1000); 00047 } 00048 void NtpClientQueryStartInterval(int type) 00049 { 00050 startNtpMs = MsTimerCount; 00051 intervalTypeNtp = type; 00052 } 00053 00054 void writeRequest(char* pPacket, int* pSize) 00055 { 00056 NtpHdrSetMode(pPacket, NTP_CLIENT); 00057 NtpHdrSetVersion (pPacket, 3); 00058 NtpHdrSetLI (pPacket, 0); 00059 NtpHdrSetStratum (pPacket, 0); 00060 NtpHdrSetPoll (pPacket, 0); 00061 NtpHdrSetPrecision (pPacket, 0); 00062 NtpHdrSetRootDelay (pPacket, 0); 00063 NtpHdrSetDispersion (pPacket, 0); 00064 char* p = NtpHdrPtrRefIdentifier(pPacket); 00065 *p++ = 0; 00066 *p++ = 0; 00067 *p++ = 0; 00068 *p = 0; 00069 NtpHdrSetRefTimeStamp(pPacket, 0); 00070 NtpHdrSetOriTimeStamp(pPacket, 0); 00071 NtpHdrSetRecTimeStamp(pPacket, 0); 00072 NtpClientQueryTime = NtpTimeStampFromClkTime(ClkTimeGet()); //Record the time to filter replies; use the costly time 00073 NtpHdrSetTraTimeStamp(pPacket, NtpClientQueryTime); 00074 00075 *pSize = NTP_HEADER_LENGTH; 00076 } 00077 00078 int NtpClientQueryPoll(int type, char* pPacket, int* pSize) 00079 { 00080 if (NtpClientQueryServerName[0]) //An empty name means ntp client is not enabled 00081 { 00082 if (intervalCompleteNtp()) //Wait for the time out 00083 { 00084 bool isMulticast = NtpClientQueryServerName[0] == '*'; 00085 if (isMulticast || Resolve(NtpClientQueryServerName, type, &NtpClientQueryServerIp4, NtpClientQueryServerIp6)) 00086 { 00087 ClkGovIsReceivingTime = false; 00088 NtpClientQueryStartInterval(NTP_QUERY_INTERVAL_RETRY); 00089 writeRequest(pPacket, pSize); 00090 00091 if (isMulticast) return MULTICAST_NTP; 00092 else return UNICAST_NTP; 00093 } 00094 } 00095 } 00096 return DO_NOTHING; 00097 }
Generated on Tue Jul 12 2022 18:53:40 by
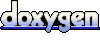