A stack which works with or without an Mbed os library. Provides IPv4 or IPv6 with a full 1500 byte buffer.
Dependents: oldheating gps motorhome heating
ntp.c
00001 #include <stdint.h> 00002 #include <stdbool.h> 00003 00004 #include "log.h" 00005 #include "net.h" 00006 #include "action.h" 00007 #include "udp.h" 00008 #include "ntpclient.h" 00009 #include "ntpserver.h" 00010 #include "clktime.h" 00011 #include "ntp.h" 00012 #include "ntphdr.h" 00013 #include "restart.h" 00014 00015 bool NtpTrace = false; 00016 00017 void NtpInit() 00018 { 00019 NtpClientInit(); 00020 } 00021 00022 void NtpLogHeader(char* pPacket) 00023 { 00024 if (NetTraceVerbose) Log ("NTP header\r\n "); 00025 else Log ("NTP header: "); 00026 00027 LogF("Mode %d", NtpHdrGetMode(pPacket)); 00028 LogF(", Version %d", NtpHdrGetVersion(pPacket)); 00029 LogF(", LI %d", NtpHdrGetLI(pPacket)); 00030 LogF(", Stratum %d", NtpHdrGetStratum(pPacket)); 00031 LogF(", Poll %d", NtpHdrGetPoll(pPacket)); 00032 LogF(", Precision %d", NtpHdrGetPrecision(pPacket)); 00033 LogF(", Root delay %d", NtpHdrGetRootDelay(pPacket)); 00034 LogF(", Dispersion %d", NtpHdrGetDispersion(pPacket)); 00035 Log (", Ident "); 00036 for (int i = 0; i < 4; i++) if (NtpHdrPtrRefIdentifier(pPacket)[i]) LogChar(NtpHdrPtrRefIdentifier(pPacket)[i]); 00037 Log ("\r\n"); 00038 00039 if (NetTraceVerbose) 00040 { 00041 LogF(" REF %llu\r\n", NtpHdrGetRefTimeStamp(pPacket)); 00042 LogF(" ORI %llu\r\n", NtpHdrGetOriTimeStamp(pPacket)); 00043 LogF(" REC %llu\r\n", NtpHdrGetRecTimeStamp(pPacket)); 00044 LogF(" TRA %llu\r\n", NtpHdrGetTraTimeStamp(pPacket)); 00045 } 00046 } 00047 00048 int NtpHandlePacketReceived(void (*traceback)(void), int sizeRx, char* pPacketRx, int* pSizeTx, char* pPacketTx) 00049 { 00050 int lastRestartPoint = RestartPoint; 00051 RestartPoint = FAULT_POINT_NtpHandlePacketReceived; 00052 00053 if (sizeRx != NTP_HEADER_LENGTH) 00054 { 00055 LogTimeF("\r\nNTP packet wrong size %d\r\n", sizeRx); 00056 return DO_NOTHING; 00057 } 00058 00059 int dest = DO_NOTHING; 00060 switch (NtpHdrGetMode(pPacketRx)) 00061 { 00062 case NTP_CLIENT: dest = NtpServerRequest(traceback, pPacketRx, pPacketTx); break; 00063 case NTP_SERVER: NtpClientReply (traceback, pPacketRx); break; 00064 default: LogTimeF("\r\nNTP packet unknown mode %d\r\n", NtpHdrGetMode(pPacketRx)); break; 00065 } 00066 00067 RestartPoint = lastRestartPoint; 00068 if (dest == DO_NOTHING) 00069 { 00070 return DO_NOTHING; 00071 } 00072 else 00073 { 00074 *pSizeTx = NTP_HEADER_LENGTH; 00075 return ActionMakeFromDestAndTrace(dest, NtpTrace && NetTraceStack); 00076 } 00077 } 00078 int NtpPollForPacketToSend(int type, char* pPacket, int* pSize) 00079 { 00080 int dest = NtpClientQueryPoll(type, pPacket, pSize); 00081 if (!dest) return DO_NOTHING; 00082 00083 if (NtpTrace) 00084 { 00085 if (NetTraceNewLine) Log("\r\n"); 00086 LogTimeF("Sending NTP request\r\n"); 00087 NtpLogHeader(pPacket); 00088 } 00089 return ActionMakeFromDestAndTrace(dest, NtpTrace && NetTraceStack); 00090 00091 00092 } 00093
Generated on Tue Jul 12 2022 18:53:40 by
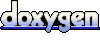