A stack which works with or without an Mbed os library. Provides IPv4 or IPv6 with a full 1500 byte buffer.
Dependents: oldheating gps motorhome heating
nrtest.c
00001 #include <stdbool.h> 00002 #include <stdint.h> 00003 00004 #include "nr.h" 00005 #include "ar6.h" 00006 #include "ndp.h" 00007 #include "dnsquery.h" 00008 #include "dns.h" 00009 #include "eth.h" 00010 #include "ip4addr.h" 00011 #include "ip6addr.h" 00012 #include "log.h" 00013 #include "mac.h" 00014 00015 char NrTest[NR_NAME_MAX_LENGTH]; 00016 00017 static int _ipProtocol = 0; 00018 static int _dnsProtocol = 0; 00019 static bool _makeRequestForNameFromAddr4 = false; 00020 static bool _makeRequestForNameFromAddr6 = false; 00021 static bool _makeRequestForAddr4FromName = false; 00022 static bool _makeRequestForAddr6FromName = false; 00023 00024 static uint32_t _addr4; 00025 static char _addr6[16]; 00026 00027 void NrTestSendRequest(int ipProtocol, int dnsProtocol) 00028 { 00029 _ipProtocol = ipProtocol; 00030 _dnsProtocol = dnsProtocol; 00031 00032 if (NrTest[0]) 00033 { 00034 _addr4 = Ip4AddrParse(NrTest); 00035 if (_addr4) 00036 { 00037 _makeRequestForNameFromAddr4 = true; 00038 } 00039 else 00040 { 00041 Ip6AddrParse(NrTest, _addr6); 00042 if (!Ip6AddrIsEmpty(_addr6)) 00043 { 00044 _makeRequestForNameFromAddr6 = true; 00045 } 00046 else 00047 { 00048 _makeRequestForAddr4FromName = true; 00049 _makeRequestForAddr6FromName = true; 00050 } 00051 } 00052 } 00053 } 00054 00055 static bool getMacOfDnsServer6() 00056 { 00057 //For IPv6 UDNS check if have the MAC for the DNS server and, if not, request it and stop 00058 if (_ipProtocol == ETH_IPV6 && _dnsProtocol == DNS_PROTOCOL_UDNS) 00059 { 00060 char mac[6]; 00061 Ar6IpToMac(NdpDnsServer, mac); 00062 if (MacIsEmpty(mac)) 00063 { 00064 Ar6MakeRequestForMacFromIp(NdpDnsServer); //The request is only repeated if made after a freeze time - call as often as you want. 00065 return false; 00066 } 00067 } 00068 return true; 00069 } 00070 00071 void NrTestMain(void) 00072 { 00073 if (DnsQueryIsBusy) return; 00074 00075 00076 if (_makeRequestForNameFromAddr4) 00077 { 00078 //if (!getMacOfDnsServer6()) return; 00079 LogTime("NrTest - making "); DnsProtocolLog(_dnsProtocol); Log(" request over "); EthProtocolLog(_ipProtocol); LogF(" for name from '%s'\r\n", NrTest); 00080 DnsQueryNameFromIp4(_addr4, _dnsProtocol, _ipProtocol); 00081 _makeRequestForNameFromAddr4 = false; 00082 return; 00083 } 00084 if (_makeRequestForNameFromAddr6) 00085 { 00086 //if (!getMacOfDnsServer6()) return; 00087 LogTime("NrTest - making "); DnsProtocolLog(_dnsProtocol); Log(" request over "); EthProtocolLog(_ipProtocol); LogF(" for name from '%s'\r\n", NrTest); 00088 DnsQueryNameFromIp6(_addr6, _dnsProtocol, _ipProtocol); 00089 _makeRequestForNameFromAddr6 = false; 00090 return; 00091 } 00092 if (_makeRequestForAddr4FromName) 00093 { 00094 //if (!getMacOfDnsServer6()) return; 00095 LogTime("NrTest - making "); DnsProtocolLog(_dnsProtocol); Log(" request over "); EthProtocolLog(_ipProtocol); LogF(" for A from '%s'\r\n", NrTest); 00096 DnsQueryIp4FromName(NrTest, _dnsProtocol, _ipProtocol); 00097 _makeRequestForAddr4FromName = false; 00098 return; 00099 } 00100 if (_makeRequestForAddr6FromName) 00101 { 00102 //if (!getMacOfDnsServer6()) return; 00103 LogTime("NrTest - making "); DnsProtocolLog(_dnsProtocol); Log(" request over "); EthProtocolLog(_ipProtocol); LogF(" for AAAA from '%s'\r\n", NrTest); 00104 DnsQueryIp6FromName(NrTest, _dnsProtocol, _ipProtocol); 00105 _makeRequestForAddr6FromName = false; 00106 return; 00107 } 00108 }
Generated on Tue Jul 12 2022 18:53:40 by
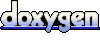